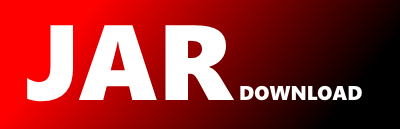
org.openide.nodes.NodeLookup Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.openide.nodes;
import java.awt.EventQueue;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import java.util.concurrent.Executor;
import org.openide.util.Lookup.Template;
import org.openide.util.lookup.AbstractLookup;
/** A lookup that represents content of a Node.getCookie and the node itself.
*
*
* @author Jaroslav Tulach
*/
final class NodeLookup extends AbstractLookup {
/** See #40734 and NodeLookupTest and CookieActionIsTooSlowTest.
* When finding action state for FilterNode, the action might been
* triggered way to many times, due to initialization in beforeLookup
* that triggered LookupListener and PROP_COOKIE change.
*/
static final ThreadLocal NO_COOKIE_CHANGE = new ThreadLocal();
private final AggregatingExecutor EXECUTOR = new AggregatingExecutor();
/** Set of Classes that we have already queried Class */
private Collection queriedCookieClasses = new ArrayList<>();
/** node we are associated with
*/
private Node node;
/** New flat lookup.
*/
public NodeLookup(Node n) {
super();
this.node = n;
addPair(new CookieSetLkp.SimpleItem(n));
}
/** Calls into Node to find out if it has a cookie of given class.
* It does special tricks to make CookieSet.Entry work.
*
* @param node node to ask
* @param c class to query
* @param collection to put Pair into if found
*/
private static void addCookie(Node node, Class> c,
Collection collection,
java.util.Map fromPairToClass) {
Object res;
Collection pairs;
Object prev = CookieSet.entryQueryMode(c);
try {
@SuppressWarnings("unchecked")
Class extends Node.Cookie> fake = (Class extends Node.Cookie>)c;
res = node.getCookie(fake);
} finally {
pairs = CookieSet.exitQueryMode(prev);
}
if (pairs == null) {
if (res == null) {
return;
}
CookieSetLkp.SimpleItem
© 2015 - 2025 Weber Informatics LLC | Privacy Policy