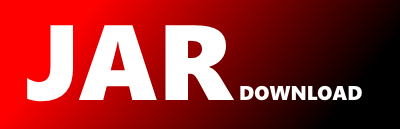
org.openide.util.lookup.SimpleLookup Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.openide.util.lookup;
import org.openide.util.Lookup;
import org.openide.util.LookupListener;
import java.util.*;
/**
* Simple lookup implementation. It can be used to create temporary lookups
* that do not change over time. The result stores references to all objects
* passed in the constructor. Those objecst are the only ones returned as
* result.
* @author David Strupl
*/
class SimpleLookup extends org.openide.util.Lookup {
/** This variable is initialized in constructor and thus null
* value is not allowed as its value. */
private Collection- > allItems;
/**
* Creates new Result object with supplied instances parameter.
* @param instances to be used to return from the lookup
*/
SimpleLookup(Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy