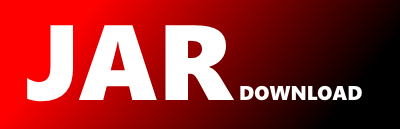
org.netbeans.lib.profiler.heap.AbstractLongMap Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.lib.profiler.heap;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
/**
* @author Tomas Hurka
*/
abstract class AbstractLongMap {
//~ Inner Classes ------------------------------------------------------------------------------------------------------------
abstract class Entry {
}
//~ Instance fields ----------------------------------------------------------------------------------------------------------
private final int VALUE_SIZE;
final int ENTRY_SIZE;
private File tempFile;
long fileSize;
private long keys;
final int KEY_SIZE;
final int ID_SIZE;
final int FOFFSET_SIZE;
Data dumpBuffer;
CacheDirectory cacheDirectory;
//~ Constructors -------------------------------------------------------------------------------------------------------------
AbstractLongMap(int size,int idSize,int foffsetSize,int valueSize,CacheDirectory cacheDir) throws FileNotFoundException, IOException {
assert idSize == 4 || idSize == 8;
assert foffsetSize == 4 || foffsetSize == 8;
keys = (size * 4L) / 3L;
ID_SIZE = idSize;
FOFFSET_SIZE = foffsetSize;
KEY_SIZE = ID_SIZE;
VALUE_SIZE = valueSize;
ENTRY_SIZE = KEY_SIZE + VALUE_SIZE;
fileSize = keys * ENTRY_SIZE;
tempFile = cacheDir.createTempFile("NBProfiler", ".map"); // NOI18N
RandomAccessFile file = tempFile.newRandomAccessFile("rw"); // NOI18N
if (Boolean.getBoolean("org.netbeans.lib.profiler.heap.zerofile")) { // NOI18N
byte[] zeros = new byte[512*1024];
while(file.length() Integer.MAX_VALUE) {
dumpBuffer = new LongMemoryMappedData(file, length, ENTRY_SIZE);
} else {
dumpBuffer = new MemoryMappedData(file, length);
}
} catch (IOException ex) {
if (ex.getCause() instanceof OutOfMemoryError) {
dumpBuffer = new FileData(file, length);
} else {
throw ex;
}
}
}
long getID(long index) {
if (ID_SIZE == 4) {
return ((long)dumpBuffer.getInt(index)) & 0xFFFFFFFFL;
}
return dumpBuffer.getLong(index);
}
void putID(long index,long key) {
if (ID_SIZE == 4) {
dumpBuffer.putInt(index,(int)key);
} else {
dumpBuffer.putLong(index,key);
}
}
long getFoffset(long index) {
if (FOFFSET_SIZE == 4) {
return dumpBuffer.getInt(index);
}
return dumpBuffer.getLong(index);
}
void putFoffset(long index,long key) {
if (FOFFSET_SIZE == 4) {
dumpBuffer.putInt(index,(int)key);
} else {
dumpBuffer.putLong(index,key);
}
}
//---- Serialization support
void writeToStream(DataOutputStream out) throws IOException {
out.writeLong(keys);
out.writeInt(ID_SIZE);
out.writeInt(FOFFSET_SIZE);
out.writeInt(VALUE_SIZE);
out.writeUTF(tempFile.getAbsolutePath());
dumpBuffer.force(tempFile);
}
AbstractLongMap(DataInputStream dis, CacheDirectory cacheDir) throws IOException {
keys = dis.readLong();
ID_SIZE = dis.readInt();
FOFFSET_SIZE = dis.readInt();
VALUE_SIZE = dis.readInt();
tempFile = cacheDir.getCacheFile(dis.readUTF());
KEY_SIZE = ID_SIZE;
ENTRY_SIZE = KEY_SIZE + VALUE_SIZE;
fileSize = keys * ENTRY_SIZE;
RandomAccessFile file = tempFile.newRandomAccessFile("rw"); // NOI18N
setDumpBuffer(file);
cacheDirectory = cacheDir;
}
private long getIndex(long key) {
long hash = key & 0x7FFFFFFFFFFFFFFFL;
return (hash % keys) * ENTRY_SIZE;
}
private long getNextIndex(long index) {
index += ENTRY_SIZE;
if (index >= fileSize) {
index = 0;
}
return index;
}
abstract Entry createEntry(long index);
abstract Entry createEntry(long index,long value);
interface Data {
//~ Methods --------------------------------------------------------------------------------------------------------------
byte getByte(long index);
int getInt(long index);
long getLong(long index);
void putByte(long index, byte data);
void putInt(long index, int data);
void putLong(long index, long data);
void force(File bufferFile) throws IOException;
}
private class FileData implements Data {
//~ Instance fields ------------------------------------------------------------------------------------------------------
RandomAccessFile file;
byte[] buf;
boolean bufferModified;
long offset;
static final int BUFFER_SIZE = 128;
//~ Constructors ---------------------------------------------------------------------------------------------------------
FileData(RandomAccessFile f, long length) throws IOException {
file = f;
buf = new byte[ENTRY_SIZE*BUFFER_SIZE];
}
//~ Methods --------------------------------------------------------------------------------------------------------------
public synchronized byte getByte(long index) {
int i = loadBufferIfNeeded(index);
return buf[i];
}
public synchronized int getInt(long index) {
int i = loadBufferIfNeeded(index);
int ch1 = ((int) buf[i++]) & 0xFF;
int ch2 = ((int) buf[i++]) & 0xFF;
int ch3 = ((int) buf[i++]) & 0xFF;
int ch4 = ((int) buf[i]) & 0xFF;
return ((ch1 << 24) + (ch2 << 16) + (ch3 << 8) + (ch4 << 0));
}
public synchronized long getLong(long index) {
int i = loadBufferIfNeeded(index);
return (((long)buf[i++] << 56) +
((long)(buf[i++] & 255) << 48) +
((long)(buf[i++] & 255) << 40) +
((long)(buf[i++] & 255) << 32) +
((long)(buf[i++] & 255) << 24) +
((buf[i++] & 255) << 16) +
((buf[i++] & 255) << 8) +
((buf[i++] & 255) << 0));
}
public synchronized void putByte(long index, byte data) {
int i = loadBufferIfNeeded(index);
buf[i] = data;
bufferModified = true;
}
public synchronized void putInt(long index, int data) {
int i = loadBufferIfNeeded(index);
buf[i++] = (byte) (data >>> 24);
buf[i++] = (byte) (data >>> 16);
buf[i++] = (byte) (data >>> 8);
buf[i++] = (byte) (data >>> 0);
bufferModified = true;
}
public synchronized void putLong(long index, long data) {
int i = loadBufferIfNeeded(index);
buf[i++] = (byte) (data >>> 56);
buf[i++] = (byte) (data >>> 48);
buf[i++] = (byte) (data >>> 40);
buf[i++] = (byte) (data >>> 32);
buf[i++] = (byte) (data >>> 24);
buf[i++] = (byte) (data >>> 16);
buf[i++] = (byte) (data >>> 8);
buf[i++] = (byte) (data >>> 0);
bufferModified = true;
}
private int loadBufferIfNeeded(long index) {
int i = (int) (index % (ENTRY_SIZE * BUFFER_SIZE));
long newOffset = index - i;
if (offset != newOffset) {
try {
flush();
file.seek(newOffset);
file.readFully(buf,0,getBufferSize(newOffset));
} catch (IOException ex) {
Systems.printStackTrace(ex);
}
offset = newOffset;
}
return i;
}
private int getBufferSize(long off) {
int size = buf.length;
if (fileSize-off> BUFFER_SIZE_BITS);
}
private int getBufferOffset(long index) {
return (int) (index & BUFFER_SIZE_MASK);
}
@Override
public void force(File bufferFile) throws IOException {
dumpBuffer = bufferFile.force(MemoryMappedData.MAP_MODE, dumpBuffer, BUFFER_SIZE, BUFFER_EXT, entrySize);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy