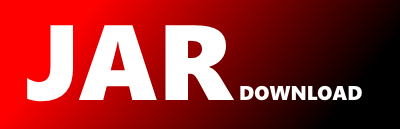
org.netbeans.lib.profiler.heap.HprofHeap Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.lib.profiler.heap;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Properties;
import static org.netbeans.lib.profiler.heap.Systems.DEBUG;
/**
*
* @author Tomas Hurka
*/
class HprofHeap implements Heap {
//~ Static fields/initializers -----------------------------------------------------------------------------------------------
// dump tags
static final int STRING = 1;
static final int LOAD_CLASS = 2;
private static final int UNLOAD_CLASS = 3;
static final int STACK_FRAME = 4;
static final int STACK_TRACE = 5;
private static final int ALLOC_SITES = 6;
static final int HEAP_SUMMARY = 7;
private static final int START_THREAD = 0xa;
private static final int END_THREAD = 0xb;
private static final int HEAP_DUMP = 0xc;
private static final int HEAP_DUMP_SEGMENT = 0x1c;
private static final int HEAP_DUMP_END = 0x2c;
private static final int CPU_SAMPLES = 0xd;
private static final int CONTROL_SETTINGS = 0xe;
// heap dump tags
static final int ROOT_UNKNOWN = 0xff;
static final int ROOT_JNI_GLOBAL = 1;
static final int ROOT_JNI_LOCAL = 2;
static final int ROOT_JAVA_FRAME = 3;
static final int ROOT_NATIVE_STACK = 4;
static final int ROOT_STICKY_CLASS = 5;
static final int ROOT_THREAD_BLOCK = 6;
static final int ROOT_MONITOR_USED = 7;
static final int ROOT_THREAD_OBJECT = 8;
static final int CLASS_DUMP = 0x20;
static final int INSTANCE_DUMP = 0x21;
static final int OBJECT_ARRAY_DUMP = 0x22;
static final int PRIMITIVE_ARRAY_DUMP = 0x23;
// HPROF HEAP 1.0.3 tags
static final int HEAP_DUMP_INFO = 0xfe;
static final int ROOT_INTERNED_STRING = 0x89;
static final int ROOT_FINALIZING = 0x8a;
static final int ROOT_DEBUGGER = 0x8b;
static final int ROOT_REFERENCE_CLEANUP = 0x8c;
static final int ROOT_VM_INTERNAL = 0x8d;
static final int ROOT_JNI_MONITOR = 0x8e;
static final int UNREACHABLE = 0x90; /* deprecated */
static final int PRIMITIVE_ARRAY_NODATA_DUMP = 0xc3;
// basic type
static final int OBJECT = 2;
static final int BOOLEAN = 4;
static final int CHAR = 5;
static final int FLOAT = 6;
static final int DOUBLE = 7;
static final int BYTE = 8;
static final int SHORT = 9;
static final int INT = 10;
static final int LONG = 11;
private static final String SNAPSHOT_ID = "NBPHD";
private static final int SNAPSHOT_VERSION = 2;
//~ Instance fields ----------------------------------------------------------------------------------------------------------
HprofByteBuffer dumpBuffer;
LongMap idToOffsetMap;
private NearestGCRoot nearestGCRoot;
final HprofGCRoots gcRoots;
private ComputedSummary computedSummary;
private final Object computedSummaryLock = new Object();
private DominatorTree domTree;
private TagBounds allInstanceDumpBounds;
private TagBounds heapDumpSegment;
private TagBounds[] heapTagBounds;
private TagBounds[] tagBounds = new TagBounds[0xff];
private boolean instancesCountComputed;
private final Object instancesCountLock = new Object();
private boolean referencesComputed;
private final Object referencesLock = new Object();
private boolean retainedSizeComputed;
private final Object retainedSizeLock = new Object();
private boolean retainedSizeByClassComputed;
private final Object retainedSizeByClassLock = new Object();
private int idMapSize;
private final int segment;
// for serialization
final File heapDumpFile;
final CacheDirectory cacheDirectory;
//~ Constructors -------------------------------------------------------------------------------------------------------------
HprofHeap(File dumpFile, int seg, CacheDirectory cacheDir) throws FileNotFoundException, IOException {
cacheDirectory = cacheDir;
dumpBuffer = HprofByteBuffer.createHprofByteBuffer(dumpFile);
segment = seg;
fillTagBounds(dumpBuffer.getHeaderSize());
heapDumpSegment = computeHeapDumpStart();
if (heapDumpSegment != null) {
fillHeapTagBounds();
}
idToOffsetMap = new LongMap(idMapSize,dumpBuffer.getIDSize(),dumpBuffer.getFoffsetSize(), cacheDirectory);
nearestGCRoot = new NearestGCRoot(this);
gcRoots = new HprofGCRoots(this);
heapDumpFile = dumpFile;
}
HprofHeap(ByteBuffer bb, int seg, CacheDirectory cacheDir) throws IOException {
cacheDirectory = cacheDir;
dumpBuffer = HprofByteBuffer.createHprofByteBuffer(bb);
segment = seg;
fillTagBounds(dumpBuffer.getHeaderSize());
heapDumpSegment = computeHeapDumpStart();
if (heapDumpSegment != null) {
fillHeapTagBounds();
}
idToOffsetMap = new LongMap(idMapSize,dumpBuffer.getIDSize(),dumpBuffer.getFoffsetSize(), cacheDirectory);
nearestGCRoot = new NearestGCRoot(this);
gcRoots = new HprofGCRoots(this);
heapDumpFile = null;
}
//~ Methods ------------------------------------------------------------------------------------------------------------------
public List /**/ getAllClasses() {
ClassDumpSegment classDumpBounds;
if (heapDumpSegment == null) {
return Collections.EMPTY_LIST;
}
classDumpBounds = getClassDumpSegment();
if (classDumpBounds == null) {
return Collections.EMPTY_LIST;
}
return classDumpBounds.createClassCollection();
}
public List getBiggestObjectsByRetainedSize(int number) {
long[] ids;
List bigObjects = new ArrayList(number);
computeRetainedSize();
ids = idToOffsetMap.getBiggestObjectsByRetainedSize(number);
for (int i=0;i broken heap dump
throw new IOException("Heap dump is broken.\nTag 0x"+Integer.toHexString(tag)+" at offset "+start+" has zero length.");
}
if (bounds == null) {
TagBounds newBounds;
if (tag == LOAD_CLASS) {
newBounds = new LoadClassSegment(this, start, end);
} else if (tag == STRING) {
newBounds = new StringSegment(this, start, end);
} else if (tag == STACK_TRACE) {
newBounds = new StackTraceSegment(this, start, end);
} else if (tag == STACK_FRAME) {
newBounds = new StackFrameSegment(this, start, end);
} else {
newBounds = new TagBounds(tag, start, end);
}
tagBounds[tag] = newBounds;
} else {
bounds.endOffset = end;
}
}
}
private int readConstantPool(long[] offset) {
long start = offset[0];
int size = dumpBuffer.getShort(start);
offset[0] += 2;
for (int i = 0; i < size; i++) {
offset[0] += 2;
readValue(offset);
}
return (int) (offset[0] - start);
}
private int readInstanceFields(long[] offset) {
long position = offset[0];
int fields = dumpBuffer.getShort(offset[0]);
offset[0] += 2;
if (DEBUG) {
for (int i = 0; i < fields; i++) {
long nameId = dumpBuffer.getID(offset[0]);
offset[0] += dumpBuffer.getIDSize();
byte type = dumpBuffer.get(offset[0]++);
Systems.debug("Instance field name ID " + nameId + " Type " + type); // NOI18N
}
} else {
offset[0] += (fields * (dumpBuffer.getIDSize() + 1));
}
return (int) (offset[0] - position);
}
private int readStaticFields(long[] offset) {
long start = offset[0];
int fields = dumpBuffer.getShort(start);
offset[0] += 2;
int idSize = dumpBuffer.getIDSize();
for (int i = 0; i < fields; i++) {
if (DEBUG) {
long nameId = dumpBuffer.getID(offset[0]);
Systems.debug("Static field name ID " + nameId + " "); // NOI18N
}
offset[0] += idSize;
byte type = readValue(offset);
}
return (int) (offset[0] - start);
}
private byte readValue(long[] offset) {
byte type = dumpBuffer.get(offset[0]++);
offset[0] += getValueSize(type);
return type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy