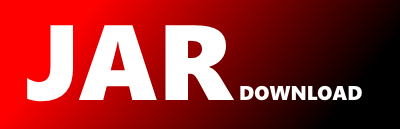
org.netbeans.lib.profiler.heap.LongSet Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.lib.profiler.heap;
import java.util.Arrays;
import java.util.Set;
/**
* Set for longs. IdentityHashMap was used as template.
* Zero cannot be used as key. Load factor is 3/4.
* @author Tomas Hurka
*/
class LongSet
{
/**
* The initial capacity used by the no-args constructor.
* MUST be a power of two. The value 32 corresponds to the
* (specified) expected maximum size of 21, given a load factor
* of 2/3.
*/
private static final int DEFAULT_CAPACITY = 32;
/**
* The minimum capacity, used if a lower value is implicitly specified
* by either of the constructors with arguments. The value 4 corresponds
* to an expected maximum size of 2, given a load factor of 2/3.
* MUST be a power of two.
*/
private static final int MINIMUM_CAPACITY = 4;
/**
* The maximum capacity, used if a higher value is implicitly specified
* by either of the constructors with arguments.
* MUST be a power of two <= 1<<29.
*/
private static final int MAXIMUM_CAPACITY = 1 << 30;
/**
* The table, resized as necessary. Length MUST always be a power of two.
*/
private transient long[] table;
/**
* The number of key-value mappings contained in this identity hash map.
*
* @serial
*/
private int size;
/**
* The number of modifications, to support fast-fail iterators
*/
private transient volatile int modCount;
/**
* The next size value at which to resize (capacity * load factor).
*/
private transient int threshold;
/**
* Constructs a new, empty identity hash map with a default expected
* maximum size (21).
*/
LongSet() {
init(DEFAULT_CAPACITY);
}
/**
* Constructs a new, empty map with the specified expected maximum size.
* Putting more than the expected number of key-value mappings into
* the map may cause the internal data structure to grow, which may be
* somewhat time-consuming.
*
* @param expectedMaxSize the expected maximum size of the map
* @throws IllegalArgumentException if expectedMaxSize is negative
*/
LongSet(int expectedMaxSize) {
if (expectedMaxSize < 0)
throw new IllegalArgumentException("expectedMaxSize is negative: "
+ expectedMaxSize);
init(capacity(expectedMaxSize));
}
/**
* Returns the appropriate capacity for the specified expected maximum
* size. Returns the smallest power of two between MINIMUM_CAPACITY
* and MAXIMUM_CAPACITY, inclusive, that is greater than
* (4 * expectedMaxSize)/3, if such a number exists. Otherwise
* returns MAXIMUM_CAPACITY. If (4 * expectedMaxSize)/3 is negative, it
* is assumed that overflow has occurred, and MAXIMUM_CAPACITY is returned.
*/
private int capacity(int expectedMaxSize) {
// Compute min capacity for expectedMaxSize given a load factor of 3/4
int minCapacity = (4 * expectedMaxSize)/3;
// Compute the appropriate capacity
int result;
if (minCapacity > MAXIMUM_CAPACITY || minCapacity < 0) {
result = MAXIMUM_CAPACITY;
} else {
result = MINIMUM_CAPACITY;
while (result < minCapacity)
result <<= 1;
}
return result;
}
/**
* Initializes object to be an empty map with the specified initial
* capacity, which is assumed to be a power of two between
* MINIMUM_CAPACITY and MAXIMUM_CAPACITY inclusive.
*/
private void init(int initCapacity) {
// assert (initCapacity & -initCapacity) == initCapacity; // power of 2
// assert initCapacity >= MINIMUM_CAPACITY;
// assert initCapacity <= MAXIMUM_CAPACITY;
threshold = (initCapacity * 3) / 4;
table = new long[initCapacity];
}
/**
* Constructs a new identity hash map containing the keys-value mappings
* in the specified map.
*
* @param m the map whose mappings are to be placed into this map
* @throws NullPointerException if the specified map is null
*/
LongSet(Set m) {
// Allow for a bit of growth
this((int) ((1 + m.size()) * 1.1));
putAll(m);
}
/**
* Returns the number of key-value mappings in this identity hash map.
*
* @return the number of key-value mappings in this map
*/
int size() {
return size;
}
/**
* Returns true if this identity hash map contains no key-value
* mappings.
*
* @return true if this identity hash map contains no key-value
* mappings
*/
boolean isEmpty() {
return size == 0;
}
/**
* Returns index for Object x.
*/
private static int hash(long x, int length) {
int h = (int)(x ^ (x >>> 32));
// This function ensures that hashCodes that differ only by
// constant multiples at each bit position have a bounded
// number of collisions (approximately 8 at default load factor).
h ^= (h >>> 20) ^ (h >>> 12);
h ^= (h >>> 7) ^ (h >>> 4);
return (h) & (length - 1);
}
/**
* Circularly traverses table of size len.
*/
private static int nextKeyIndex(int i, int len) {
return (i + 1 < len ? i + 1 : 0);
}
/**
* Tests whether the specified object reference is a key in this identity
* hash map.
*
* @param key possible key
* @return true
if the specified object reference is a key
* in this map
* @see #containsValue(Object)
*/
boolean contains(long key) {
assert key != 0;
long[] tab = table;
int len = tab.length;
int i = hash(key, len);
while (true) {
long item = tab[i];
if (item == key)
return true;
if (item == 0)
return false;
i = nextKeyIndex(i, len);
}
}
/**
* Associates the specified value with the specified key in this identity
* hash map. If the map previously contained a mapping for the key, the
* old value is replaced.
*
* @param key the key with which the specified value is to be associated
* @param value the value to be associated with the specified key
* @return the previous value associated with key, or
* null if there was no mapping for key.
* (A null return can also indicate that the map
* previously associated null with key.)
* @see Object#equals(Object)
* @see #get(Object)
* @see #containsKey(Object)
*/
boolean add(long key) {
assert key != 0;
long[] tab = table;
int len = tab.length;
int i = hash(key, len);
long item;
while ( (item = tab[i]) != 0) {
if (item == key) {
return true;
}
i = nextKeyIndex(i, len);
}
modCount++;
tab[i] = key;
if (++size >= threshold)
resize(2*len);
return false;
}
/**
* Resize the table to hold given capacity.
*
* @param newCapacity the new capacity, must be a power of two.
*/
private void resize(int newCapacity) {
// assert (newCapacity & -newCapacity) == newCapacity; // power of 2
int newLength = newCapacity;
long[] oldTable = table;
int oldLength = oldTable.length;
if (oldLength == MAXIMUM_CAPACITY) { // can't expand any further
if (threshold == MAXIMUM_CAPACITY-1)
throw new IllegalStateException("Capacity exhausted.");
threshold = MAXIMUM_CAPACITY-1; // Gigantic map!
return;
}
if (oldLength >= newLength)
return;
long[] newTable = new long[newLength];
threshold = (newLength * 3) / 4;
for (int j = 0; j < oldLength; j++) {
long key = oldTable[j];
if (key != 0) {
int i = hash(key, newLength);
while (newTable[i] != 0)
i = nextKeyIndex(i, newLength);
newTable[i] = key;
}
}
table = newTable;
}
/**
* Copies all of the mappings from the specified map to this map.
* These mappings will replace any mappings that this map had for
* any of the keys currently in the specified map.
*
* @param m mappings to be stored in this map
* @throws NullPointerException if the specified map is null
*/
void putAll(Set m) {
int n = m.size();
if (n == 0)
return;
if (n > threshold) // conservatively pre-expand
resize(capacity(n));
for (Long e : m) {
add(e);
}
}
/**
* Removes the mapping for this key from this map if present.
*
* @param key key whose mapping is to be removed from the map
* @return the previous value associated with key, or
* null if there was no mapping for key.
* (A null return can also indicate that the map
* previously associated null with key.)
*/
boolean remove(long key) {
key++;
long[] tab = table;
int len = tab.length;
int i = hash(key, len);
while (true) {
long item = tab[i];
if (item == key) {
modCount++;
size--;
tab[i] = 0;
closeDeletion(i);
return true;
}
if (item == 0)
return false;
i = nextKeyIndex(i, len);
}
}
/**
* Rehash all possibly-colliding entries following a
* deletion. This preserves the linear-probe
* collision properties required by get, put, etc.
*
* @param d the index of a newly empty deleted slot
*/
private void closeDeletion(int d) {
// Adapted from Knuth Section 6.4 Algorithm R
long[] tab = table;
int len = tab.length;
// Look for items to swap into newly vacated slot
// starting at index immediately following deletion,
// and continuing until a null slot is seen, indicating
// the end of a run of possibly-colliding keys.
long item;
for (int i = nextKeyIndex(d, len); (item = tab[i]) != 0;
i = nextKeyIndex(i, len) ) {
// The following test triggers if the item at slot i (which
// hashes to be at slot r) should take the spot vacated by d.
// If so, we swap it in, and then continue with d now at the
// newly vacated i. This process will terminate when we hit
// the null slot at the end of this run.
// The test is messy because we are using a circular table.
int r = hash(item, len);
if ((i < r && (r <= d || d <= i)) || (r <= d && d <= i)) {
tab[d] = item;
tab[i] = 0;
d = i;
}
}
}
/**
* Removes all of the mappings from this map.
* The map will be empty after this call returns.
*/
void clear() {
modCount++;
long[] tab = table;
Arrays.fill(tab,0);
size = 0;
}
/**
* Compares the specified object with this map for equality. Returns
* true if the given object is also a map and the two maps
* represent identical object-reference mappings. More formally, this
* map is equal to another map m if and only if
* this.entrySet().equals(m.entrySet()).
*
* Owing to the reference-equality-based semantics of this map it is
* possible that the symmetry and transitivity requirements of the
* Object.equals contract may be violated if this map is compared
* to a normal map. However, the Object.equals contract is
* guaranteed to hold among IdentityHashMap instances.
*
* @param o object to be compared for equality with this map
* @return true if the specified object is equal to this map
* @see Object#equals(Object)
*/
public boolean equals(Object o) {
if (o == this) {
return true;
} else if (o instanceof LongSet) {
LongSet m = (LongSet) o;
if (m.size() != size)
return false;
long[] tab = m.table;
for (int i = 0; i < tab.length; i++) {
long k = tab[i];
if (k != 0 && !contains(k))
return false;
}
return true;
} else {
return false; // o is not a Map
}
}
/**
* Returns the hash code value for this map. The hash code of a map is
* defined to be the sum of the hash codes of each entry in the map's
* entrySet() view. This ensures that m1.equals(m2)
* implies that m1.hashCode()==m2.hashCode() for any two
* IdentityHashMap instances m1 and m2, as
* required by the general contract of {@link Object#hashCode}.
*
*
Owing to the reference-equality-based semantics of the
* Map.Entry instances in the set returned by this map's
* entrySet method, it is possible that the contractual
* requirement of Object.hashCode mentioned in the previous
* paragraph will be violated if one of the two objects being compared is
* an IdentityHashMap instance and the other is a normal map.
*
* @return the hash code value for this map
* @see Object#equals(Object)
* @see #equals(Object)
*/
public int hashCode() {
int result = 0;
long[] tab = table;
for (int i = 0; i < tab.length; i ++) {
long key = tab[i];
if (key != 0) {
result += hash(key, tab.length);
}
}
return result;
}
}