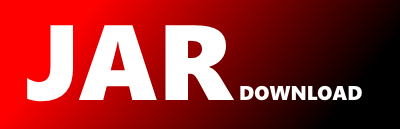
org.netbeans.lib.profiler.wireprotocol.VMPropertiesResponse Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.lib.profiler.wireprotocol;
import org.netbeans.lib.profiler.global.CommonConstants;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
/**
* This response is generated by the back end and contains the VM properties such as various class paths.
*
* @author Tomas Hurka
* @author Misha Dmitriev
* @author Ian Formanek
*/
public class VMPropertiesResponse extends Response {
//~ Instance fields ----------------------------------------------------------------------------------------------------------
private String bootClassPath;
private String javaClassPath;
private String javaCommand;
private String javaExtDirs;
private String jdkVersionString;
private String jvmArguments;
private String targetMachineOSName;
private String workingDir;
private boolean canInstrumentConstructor;
private int agentId;
private int agentVersion;
private long maxHeapSize;
private long startupTimeInCounts;
private long startupTimeMillis;
//~ Constructors -------------------------------------------------------------------------------------------------------------
public VMPropertiesResponse(String jdkVerString, String javaClassPath, String javaExtDirs, String bootClassPath,
String workingDir, String jvmArguments, String javaCommand, String targetMachineOSName,
boolean canInstrumentConstructor, long maxHeapSize, long startupTimeMillis,
long startupTimeInCounts, int agentId) {
super(true, VM_PROPERTIES);
this.jdkVersionString = jdkVerString;
this.javaClassPath = javaClassPath;
this.javaExtDirs = javaExtDirs;
this.bootClassPath = bootClassPath;
this.workingDir = workingDir;
this.jvmArguments = (jvmArguments != null) ? jvmArguments : ""; // NOI18N
this.javaCommand = (javaCommand != null) ? javaCommand : ""; // NOI18N
this.targetMachineOSName = targetMachineOSName;
this.canInstrumentConstructor = canInstrumentConstructor;
this.maxHeapSize = maxHeapSize;
this.startupTimeMillis = startupTimeMillis;
this.startupTimeInCounts = startupTimeInCounts & 0xFFFFFFFFFFFFFFL; // we use only 7 bytes for hi res timer
this.agentId = agentId;
this.agentVersion = CommonConstants.CURRENT_AGENT_VERSION;
}
// Custom serialization support
VMPropertiesResponse() {
super(true, VM_PROPERTIES);
}
//~ Methods ------------------------------------------------------------------------------------------------------------------
public int getAgentId() {
return agentId;
}
public int getAgentVersion() {
return agentVersion;
}
public String getBootClassPath() {
return bootClassPath;
}
public String getJDKVersionString() {
return jdkVersionString;
}
public String getJVMArguments() {
return jvmArguments;
}
public String getJavaClassPath() {
return javaClassPath;
}
public String getJavaCommand() {
return javaCommand;
}
public String getJavaExtDirs() {
return javaExtDirs;
}
public boolean canInstrumentConstructor() {
return canInstrumentConstructor;
}
public long getMaxHeapSize() {
return maxHeapSize;
}
public long getStartupTimeInCounts() {
return startupTimeInCounts;
}
public long getStartupTimeMillis() {
return startupTimeMillis;
}
public String getTargetMachineOSName() {
return targetMachineOSName;
}
public String getWorkingDir() {
return workingDir;
}
// For debugging
public String toString() {
return "VMPropertiesResponse:" // NOI18N
+ "\n jdkVersionString: " + jdkVersionString // NOI18N
+ "\n javaClassPath: " + javaClassPath // NOI18N
+ "\n javaExtDirs: " + javaExtDirs // NOI18N
+ "\n bootClassPath: " + bootClassPath // NOI18N
+ "\n workingDir: " + workingDir // NOI18N
+ "\n jvmArguments: " + jvmArguments // NOI18N
+ "\n javaCommand: " + javaCommand // NOI18N
+ "\n targetMachineOSName: " + targetMachineOSName // NOI18N
+ "\n canInstrumentConstructor: " + canInstrumentConstructor // NOI18N
+ "\n maxHeapSize: " + maxHeapSize // NOI18N
+ "\n startupTimeMillis: " + startupTimeMillis // NOI18N
+ "\n startupTimeInCounts: " + startupTimeInCounts // NOI18N
+ "\n agentId: " + agentId // NOI18N
+ "\n agentVersion: " + agentVersion // NOI18N
+ "\n" + super.toString(); // NOI18N
}
void readObject(ObjectInputStream in) throws IOException {
agentVersion = in.readInt();
jdkVersionString = in.readUTF();
javaClassPath = in.readUTF();
javaExtDirs = in.readUTF();
bootClassPath = in.readUTF();
workingDir = in.readUTF();
jvmArguments = in.readUTF();
javaCommand = in.readUTF();
targetMachineOSName = in.readUTF();
canInstrumentConstructor = in.readBoolean();
maxHeapSize = in.readLong();
startupTimeMillis = in.readLong();
startupTimeInCounts = in.readLong();
agentId = in.readInt();
}
void writeObject(ObjectOutputStream out) throws IOException {
out.writeInt(agentVersion);
out.writeUTF(jdkVersionString);
out.writeUTF(javaClassPath);
out.writeUTF(javaExtDirs);
out.writeUTF(bootClassPath);
out.writeUTF(workingDir);
out.writeUTF(jvmArguments);
out.writeUTF(javaCommand);
out.writeUTF(targetMachineOSName);
out.writeBoolean(canInstrumentConstructor);
out.writeLong(maxHeapSize);
out.writeLong(startupTimeMillis);
out.writeLong(startupTimeInCounts);
out.writeInt(agentId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy