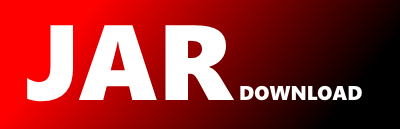
org.netbeans.modules.glassfish.tooling.GlassFishStatus Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.glassfish.tooling;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import org.netbeans.modules.glassfish.tooling.data.DataException;
import org.netbeans.modules.glassfish.tooling.data.GlassFishServer;
import org.netbeans.modules.glassfish.tooling.data.GlassFishServerStatus;
import org.netbeans.modules.glassfish.tooling.logging.Logger;
import org.netbeans.modules.glassfish.tooling.server.state.GlassFishStatusEntity;
import org.netbeans.modules.glassfish.tooling.server.state.StatusJob;
import org.netbeans.modules.glassfish.tooling.server.state.StatusScheduler;
/**
* GlassFish server status.
* Local server can be in 4 possible states:
* - Offline: Server is not running
* - no process
* - no network listeners
* - no administration interface responses
* - Startup/Restart: Server start or restart was requested
* - active process (PID should change for restart)
* - network listeners may or may not be active
* - no administration interface responses
* - Online: Server is running
* - active process
* - active network listeners
* - valid administration interface responses
* - Shutdown: Server shutdown was requested but server is still running
* - active process
* - network listeners may or may not be active
* - administration interface may or may not be active
* Remote server can be in 4 possible states:
* - Offline: Server is not running
*
- no network listeners
*
- no administration interface responses
* - Restart: Server restart was requested
* - network listeners may or may not be active
* - no administration interface responses
* - Online: Server is running
* - active network listeners
* - valid administration interface responses
* - Shutdown: Server shutdown was requested but server is still running
* - network listeners may or may not be active
* - administration interface may or may not be active
*
* @author Tomas Kraus
*/
public enum GlassFishStatus {
////////////////////////////////////////////////////////////////////////////
// Enum values //
////////////////////////////////////////////////////////////////////////////
/** Server status is unknown. */
UNKNOWN,
/** Server is offline (not running or not responding). */
OFFLINE,
/** Server start or restart was requested but server is still not
* fully responding. */
STARTUP,
/** Server is running an responding. */
ONLINE,
/** Server shutdown was requested but server is still running
* or responding. */
SHUTDOWN;
////////////////////////////////////////////////////////////////////////////
// Class attributes //
////////////////////////////////////////////////////////////////////////////
/** Logger instance for this class. */
private static final Logger LOGGER = new Logger(GlassFishStatus.class);
/** GlassFish version enumeration length. */
public static final int length = GlassFishStatus.values().length;
/** A String
representation of UNKNOWN value. */
private static final String UNKNOWN_STR = "UNKNOWN";
/** A String
representation of OFFLINE value. */
private static final String OFFLINE_STR = "OFFLINE";
/** A String
representation of STARTUP value. */
private static final String STARTUP_STR = "STARTUP";
/** A String
representation of ONLINE value. */
private static final String ONLINE_STR = "ONLINE";
/** A String
representation of SHUTDOWN value. */
private static final String SHUTDOWN_STR = "SHUTDOWN";
/** Stored String
values for backward String
* conversion. */
private static final Map stringValuesMap
= new HashMap<>(values().length);
static {
for (GlassFishStatus state : GlassFishStatus.values()) {
stringValuesMap.put(state.toString().toUpperCase(), state);
}
}
////////////////////////////////////////////////////////////////////////////
// Static methods //
////////////////////////////////////////////////////////////////////////////
/**
* Returns a GlassFishStatus
with a value represented by the
* specified String
.
*
* The GlassFishStatus
returned represents existing value only
* if specified String
matches any String
returned
* by toString
method. Otherwise null
value
* is returned.
*
* @param name Value containing GlassFishStatus
* toString
representation.
* @return GlassFishStatus
value represented
* by String
or null
if value
* was not recognized.
*/
public static GlassFishStatus toValue(final String name) {
if (name != null) {
return (stringValuesMap.get(name.toUpperCase()));
} else {
return null;
}
}
/**
* Initialize GlassFish server status task scheduler to use external
* executor.
*
* This method must be called before adding first GlassFisg server instance
* into scheduler.
*
* @param executor External executor to be used in scheduler.
*/
public static void initScheduler(
final ScheduledThreadPoolExecutor executor) {
StatusScheduler.init(executor);
}
/**
* Register GlassFish server instance into scheduler and launch server
* status checking jobs.
*
* It is possible to call this method for the same GlassFisg server instance
* many times. Server instance will be added only if it is not already
* registered.
*
* @param srv GlassFish server instance to be registered.
* @return Value of true
when server instance was successfully
* added into scheduler and status checking job was started
* or false
otherwise.
*/
public static boolean add(final GlassFishServer srv) {
StatusScheduler scheduler = StatusScheduler.getInstance();
if (!scheduler.exists(srv)) {
GlassFishStatusEntity status = new GlassFishStatusEntity(srv);
return StatusScheduler.getInstance().add(status);
} else {
return false;
}
}
/**
* Register GlassFish server instance into scheduler, register server status
* listener and launch server status checking jobs.
*
* It is possible to call this method for the same GlassFisg server instance
* many times. Server instance and listener will be added only if it is not
* already registered.
*
* @param srv GlassFish server instance to be registered.
* @param listener Server status listener to be registered.
* @param currentState Notify about current server status after every check
* when true
.
* @param newState Notify about server status change for new states
* provided as this argument.
* @return Value of true
when server instance was successfully
* added into scheduler and status checking job was started
* or false
otherwise.
*/
public static boolean add(final GlassFishServer srv,
final GlassFishStatusListener listener, final boolean currentState,
final GlassFishStatus... newState) {
StatusScheduler scheduler = StatusScheduler.getInstance();
if (!scheduler.exists(srv)) {
GlassFishStatusEntity status = new GlassFishStatusEntity(srv);
return StatusScheduler.getInstance()
.add(status, listener, currentState, newState);
} else {
return false;
}
}
/**
* Get current GlassFish server instance status.
*
* When status checking is disabled, it will restart it and return current
* status which is probably UNKNOWN
.
*
* @param srv GlassFish server instance to be searched.
* @return GlassFish server instance status. Returns UNKNOWN
* value for unregistered server instance.
*/
public static GlassFishStatus getStatus(final GlassFishServer srv) {
GlassFishServerStatus status
= StatusScheduler.getInstance().get(srv, null);
return status != null ? status.getStatus() : GlassFishStatus.UNKNOWN;
}
/**
* Get current GlassFish server instance status.
*
* When status checking is disabled, it will restart it and return current
* status which is probably UNKNOWN
. If listener is provided,
* it will be registered to receive any state change notification following
* status checking restart. This listener won't be unregistered
* automatically so caller should handle it properly.
*
* @param srv GlassFish server instance to be searched.
* @param listener Server status listener to be registered when status
* checking is being restarted.
* @return GlassFish server instance status. Returns UNKNOWN
* value for unregistered server instance.
*/
public static GlassFishStatus getStatus(final GlassFishServer srv,
final GlassFishStatusListener listener) {
GlassFishServerStatus status
= StatusScheduler.getInstance().get(srv, listener);
return status != null ? status.getStatus() : GlassFishStatus.UNKNOWN;
}
/**
* Get current GlassFish server instance {@link GlassFishServerStatus}
* object.
*
* When status checking is disabled, it will restart it and return current
* status which is probably UNKNOWN
.
*
* @param srv GlassFish server instance to be searched.
* @return GlassFish server status {@link GlassFishServerStatus} object.
* Returns null
value for unregistered server instance.
*/
public static GlassFishServerStatus get(final GlassFishServer srv) {
return StatusScheduler.getInstance().get(srv, null);
}
/**
* Get current GlassFish server instance {@link GlassFishServerStatus}
* object.
*
* When status checking is disabled, it will restart it and return current
* status which is probably UNKNOWN
. If listener is provided,
* it will be registered to receive any state change notification following
* status checking restart. This listener won't be unregistered
* automatically so caller should handle it properly.
*
* @param srv GlassFish server instance to be searched.
* @param listener Server status listener to be registered when status
* checking is being restarted.
* @return GlassFish server status {@link GlassFishServerStatus} object.
* Returns null
value for unregistered server instance.
*/
public static GlassFishServerStatus get(final GlassFishServer srv,
final GlassFishStatusListener listener) {
return StatusScheduler.getInstance().get(srv, listener);
}
/**
* Trigger startup mode for GlassFish server instance.
*
* This will switch status monitoring into startup mode where server
* is being checked more often.
*
* @param srv GlassFish server instance to be switched
* into startup mode.
* @param force Force startup mode for GlassFish server instance
* from any state then true
.
* @param listener Server status listener to be registered together with
* switching into startup mode.
* @param newState Notify about server status change for new states
* provided as this argument.
* @return Value of true
when server instance was successfully
* added into scheduler and status checking job was started
* or false
otherwise.
*/
public static boolean start(final GlassFishServer srv, final boolean force,
final GlassFishStatusListener listener,
final GlassFishStatus... newState) {
return StatusScheduler.getInstance().start(
srv, force, listener, newState);
}
/**
* Trigger startup mode for GlassFish server instance.
*
* This will switch status monitoring into startup mode where server
* is being checked more often.
*
* @param srv GlassFish server instance to be switched into startup mode.
* @return Value of true
when server instance was successfully
* added into scheduler and status checking job was started
* or false
otherwise.
*/
public static boolean start(final GlassFishServer srv) {
return StatusScheduler.getInstance().start(srv, false, null);
}
/**
* Trigger shutdown mode for GlassFish server instance.
*
* This will switch status monitoring into shutdown mode where server
* is being checked more often.
*
* @param srv GlassFish server instance to be switched into shutdown mode.
* @return Value of true
when server instance was successfully
* added into scheduler and status checking job was started
* or false
otherwise.
*/
public static boolean shutdown(final GlassFishServer srv) {
return StatusScheduler.getInstance().shutdown(srv);
}
/**
* Remove GlassFish server instance from scheduler and stop server
* status checking jobs.
*
* It is possible to call this method for the same GlassFisg server instance
* many times. Server instance will be removed only if it is registered.
*
* @param srv GlassFish server instance to be removed.
* @return Value of true
when server instance was successfully
* removed from scheduler and status checking job was stopped
* or false
when server instance was not registered.
*/
public static boolean remove(final GlassFishServer srv) {
return StatusScheduler.getInstance().remove(srv);
}
/**
* Suspend server status monitoring for GlassFisg server instance.
*
* @param srv GlassFish server instance for which to suspend monitoring.
* @return Value of true
when server instance monitoring
* was suspended or false
when server instance
* is not registered.
*/
public static boolean suspend(final GlassFishServer srv) {
return StatusScheduler.getInstance().suspend(srv);
}
/**
* Register server status listener to be notified about current server
* status after every check.
*
* @param srv GlassFish server instance being monitored.
* @param listener Server status listener to be registered.
* @return Value of true
when listener was added
* or false
when this listener was already registered
* or GlassFish server instance was not registered.
*/
public static boolean addCheckListener(final GlassFishServer srv,
final GlassFishStatusListener listener) {
final StatusJob job = StatusScheduler.getInstance().getJob(srv);
if (job != null) {
return job.addCurrStatusListener(listener);
} else {
return false;
}
}
/**
* Register server status listener to be notified about server status
* change.
*
* @param srv GlassFish server instance being monitored.
* @param listener Server status listener to be registered.
* @param newState Notify about server status change for new states
* provided as this argument.
* @return Value of true
when listener was added in at least
* one list or false
when this listener was already
* registered in all requested lists or GlassFish server instance
* was not registered.
*/
public static boolean addChangeListener(final GlassFishServer srv,
final GlassFishStatusListener listener,
final GlassFishStatus... newState) {
final StatusJob job = StatusScheduler.getInstance().getJob(srv);
if (job != null) {
return job.addNewStatusListener(listener, newState);
} else {
return false;
}
}
/**
* Register server status listener to be notified about server status
* change.
*
* @param srv GlassFish server instance being monitored.
* @param listener Server status listener to be registered.
* @return Value of true
when listener was added in at least
* one list or false
when this listener was already
* registered in all requested lists or GlassFish server instance
* was not registered.
*/
public static boolean addErrorListener(final GlassFishServer srv,
final GlassFishStatusListener listener) {
final StatusJob job = StatusScheduler.getInstance().getJob(srv);
if (job != null) {
return job.addErrorListener(listener);
} else {
return false;
}
}
/**
* Register server status listener.
*
* @param srv GlassFish server instance being monitored.
* @param listener Server status listener to be registered.
* @param currentState Notify about current server status after every check
* when true
.
* @param newState Notify about server status change for new states
* provided as this argument.
* @return Value of true
when listener was added in at least
* one list or false
when this listener was already
* registered in all requested lists or GlassFish server instance
* was not registered.
*/
public static boolean addListener(final GlassFishServer srv,
final GlassFishStatusListener listener, final boolean currentState,
final GlassFishStatus... newState) {
final StatusJob job = StatusScheduler.getInstance().getJob(srv);
if (job != null) {
return job.addStatusListener(listener, currentState, newState);
} else {
return false;
}
}
/**
* Unregister server status listener.
*
* @param srv GlassFish server instance being monitored.
* @param listener Server status listener to be unregistered.
* @return Value of true
when listener was found and removed
* or false
when listener was not found among
* registered listeners or GlassFish server instance was not
* registered.
*/
public static boolean removeListener(final GlassFishServer srv,
final GlassFishStatusListener listener) {
final StatusJob job = StatusScheduler.getInstance().getJob(srv);
if (job != null) {
return job.removeStatusListener(listener);
} else {
return false;
}
}
////////////////////////////////////////////////////////////////////////////
// Methods //
////////////////////////////////////////////////////////////////////////////
/**
* Convert GlassFishStatus
value to String
.
*
* @return A String
representation of the value of this object.
*/
@Override
public String toString() {
final String METHOD = "toString";
switch (this) {
case UNKNOWN: return UNKNOWN_STR;
case OFFLINE: return OFFLINE_STR;
case STARTUP: return STARTUP_STR;
case ONLINE: return ONLINE_STR;
case SHUTDOWN: return SHUTDOWN_STR;
// This is unrecheable. Being here means this class does not handle
// all possible values correctly.
default: throw new DataException(
LOGGER.excMsg(METHOD, "invalidState"));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy