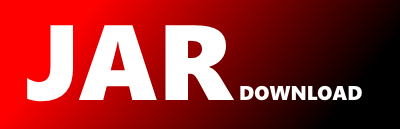
org.netbeans.modules.java.disco.BrowsePanel Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.java.disco;
import static org.netbeans.modules.java.disco.SwingWorker2.submit;
import java.io.File;
import javax.swing.JFileChooser;
import org.checkerframework.checker.guieffect.qual.UIEffect;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.openide.util.NbBundle;
@NbBundle.Messages({
"DiscoBrowsePanel.searching=Searching...",
"DiscoBrowsePanel.error=Requested JDK not found."
})
public class BrowsePanel extends javax.swing.JPanel {
private final BrowseWizardPanel panel;
private final WizardState state;
private final Client discoClient;
public BrowsePanel(BrowseWizardPanel panel, WizardState state) {
this.panel = panel;
this.state = state;
this.discoClient = Client.getInstance();
init();
}
@UIEffect
private void init() {
setName("Browse");
initComponents();
}
@Override
@UIEffect
public void addNotify() {
super.addNotify();
//we do this every time
if (state.selection.get(null) == null) {
jdkDescription.setText(Bundle.DiscoBrowsePanel_searching());
//OK, we have a quick selection so the file name was not the best, let's try to load it
submit(() -> {
return state.selection.get(discoClient);
}).then(pkg -> {
//re-set the name
jdkDescription.setText(state.selection.getFileName());
panel.fireChangeListeners();
}).handle(ex -> {
jdkDescription.setText(Bundle.DiscoBrowsePanel_error());
}).execute();
} else {
jdkDescription.setText(state.selection.getFileName());
}
}
public boolean isOK() {
return state.selection.get(null) != null
&& !downloadPathText.getText().isEmpty();
}
@NonNull
public String getDefaultDownloadFolder() {
File f = OS.getDefaultDownloadFolder();
if (f == null)
return "";
return f.getAbsolutePath();
}
public String getUserDownloadFolder() {
return downloadPathText.getText();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
javax.swing.JLabel selectedLabel = new javax.swing.JLabel();
jdkDescription = new javax.swing.JLabel();
javax.swing.JLabel downloadLabel = new javax.swing.JLabel();
downloadPathText = new javax.swing.JTextField();
chooseButton = new javax.swing.JToggleButton();
org.openide.awt.Mnemonics.setLocalizedText(selectedLabel, org.openide.util.NbBundle.getMessage(BrowsePanel.class, "BrowsePanel.selectedLabel.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jdkDescription, org.openide.util.NbBundle.getMessage(BrowsePanel.class, "BrowsePanel.jdkDescription.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(downloadLabel, org.openide.util.NbBundle.getMessage(BrowsePanel.class, "BrowsePanel.downloadLabel.text")); // NOI18N
downloadPathText.setEditable(false);
downloadPathText.setText(getDefaultDownloadFolder());
org.openide.awt.Mnemonics.setLocalizedText(chooseButton, org.openide.util.NbBundle.getMessage(BrowsePanel.class, "BrowsePanel.chooseButton.text")); // NOI18N
chooseButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
chooseButtonActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(downloadLabel)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(downloadPathText, javax.swing.GroupLayout.DEFAULT_SIZE, 194, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(chooseButton))
.addGroup(layout.createSequentialGroup()
.addComponent(selectedLabel)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jdkDescription, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addContainerGap())))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(selectedLabel)
.addComponent(jdkDescription))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(downloadLabel)
.addComponent(downloadPathText, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(chooseButton))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
}// //GEN-END:initComponents
private void chooseButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_chooseButtonActionPerformed
JFileChooser fileChooser = new JFileChooser();
fileChooser.setCurrentDirectory(new File("."));
fileChooser.setDialogTitle("Select destination folder");
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
fileChooser.setAcceptAllFileFilterUsed(false);
if (fileChooser.showOpenDialog(this) == JFileChooser.APPROVE_OPTION) {
File folder = fileChooser.getSelectedFile();
if (folder != null) {
String destinationFolder = folder.getAbsolutePath();
downloadPathText.setText(destinationFolder);
}
}
}//GEN-LAST:event_chooseButtonActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JToggleButton chooseButton;
private javax.swing.JTextField downloadPathText;
private javax.swing.JLabel jdkDescription;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy