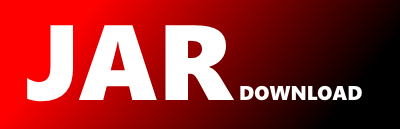
org.netbeans.modules.languages.hcl.SourceRef Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.languages.hcl;
import java.util.Collections;
import java.util.IdentityHashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.TreeMap;
import org.netbeans.modules.csl.api.OffsetRange;
import org.netbeans.modules.languages.hcl.ast.HCLElement;
import org.netbeans.modules.languages.hcl.ast.HCLElementFactory;
import org.netbeans.modules.parsing.api.Snapshot;
import org.openide.filesystems.FileObject;
/**
*
* @author Laszlo Kishalmi
*/
public class SourceRef {
public final Snapshot source;
private Map elementOffsets = new IdentityHashMap<>();
private TreeMap elementAt = new TreeMap<>((o1, o2) -> o1.getStart() != o2.getStart() ? o1.getStart() - o2.getStart() : o2.getEnd() - o1.getEnd());
public SourceRef(Snapshot source) {
this.source = source;
}
private void add(HCLElement e, OffsetRange r) {
if (!elementOffsets.containsKey(e)) {
// Only add the most specific range for the expression.
elementOffsets.put(e, r);
elementAt.put(r, e);
}
}
void elementCreated(HCLElementFactory.CreateContext ctx) {
add(ctx.element(), new OffsetRange(ctx.start().getStartIndex(), ctx.stop().getStopIndex() + 1));
}
public FileObject getFileObject() {
return source.getSource().getFileObject();
}
public Optional getOffsetRange(HCLElement e) {
return Optional.ofNullable(elementOffsets.get(e));
}
public List extends HCLElement> elementsAt(int index) {
if (index < 0) {
return Collections.emptyList();
}
List ret = new LinkedList<>();
Iterator> it = elementAt.entrySet().iterator();
while (it.hasNext()) {
Map.Entry e = it.next();
OffsetRange or = e.getKey();
if (or.getStart() > index) {
break;
}
if (or.getEnd() <= index) {
continue;
}
ret.add(e.getValue());
}
return ret.isEmpty() ? Collections.emptyList() : Collections.unmodifiableList(ret);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy