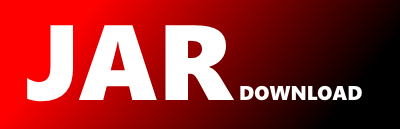
org.netbeans.modules.maven.grammar.ShowEffPomDiffPanel Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.maven.grammar;
import java.awt.Component;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.StringTokenizer;
import javax.swing.ComboBoxModel;
import javax.swing.DefaultComboBoxModel;
import javax.swing.DefaultListCellRenderer;
import javax.swing.JList;
import org.netbeans.modules.maven.api.MavenConfiguration;
import org.netbeans.modules.maven.spi.customizer.TextToValueConversions;
import org.netbeans.spi.project.ProjectConfiguration;
import org.netbeans.spi.project.ProjectConfigurationProvider;
/**
*
* @author mkleint
*/
class ShowEffPomDiffPanel extends javax.swing.JPanel {
/**
* Creates new form ShowEffPomDiffPanel
*/
public ShowEffPomDiffPanel(ProjectConfigurationProvider configs) {
initComponents();
ComboBoxModel model = new DefaultComboBoxModel(configs.getConfigurations().toArray(new MavenConfiguration[0]));
comConfiguration.setModel(model);
comConfiguration.setEditable(false);
comConfiguration.setRenderer(new DefaultListCellRenderer() {
@Override
public Component getListCellRendererComponent(JList list, Object value, int index, boolean isSelected, boolean cellHasFocus) {
return super.getListCellRendererComponent(list, ((ProjectConfiguration)value).getDisplayName(), index, isSelected, cellHasFocus);
}
});
comConfiguration.setSelectedItem(configs.getActiveConfiguration());
enableFields();
epProperties.setContentType("text/x-properties");
}
MavenConfiguration getSelectedConfig() {
return (MavenConfiguration) comConfiguration.getSelectedItem();
}
boolean isConfigurationSelected() {
return rbConfiguration.isSelected();
}
List getSelectedProfiles() {
StringTokenizer tok = new StringTokenizer(txtProfiles.getText().trim(), " ,");
ArrayList lst = new ArrayList();
while (tok.hasMoreTokens()) {
lst.add(tok.nextToken());
}
return lst;
}
Map getSelectedProperties() {
return TextToValueConversions.convertStringToActionProperties(epProperties.getText());
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
buttonGroup1 = new javax.swing.ButtonGroup();
lblConfiguration = new javax.swing.JLabel();
comConfiguration = new javax.swing.JComboBox();
rbConfiguration = new javax.swing.JRadioButton();
rbCustom = new javax.swing.JRadioButton();
lblProfiles = new javax.swing.JLabel();
txtProfiles = new javax.swing.JTextField();
lblProperties = new javax.swing.JLabel();
jScrollPane1 = new javax.swing.JScrollPane();
epProperties = new javax.swing.JEditorPane();
lblConfiguration.setLabelFor(comConfiguration);
org.openide.awt.Mnemonics.setLocalizedText(lblConfiguration, org.openide.util.NbBundle.getMessage(ShowEffPomDiffPanel.class, "ShowEffPomDiffPanel.lblConfiguration.text")); // NOI18N
buttonGroup1.add(rbConfiguration);
rbConfiguration.setSelected(true);
org.openide.awt.Mnemonics.setLocalizedText(rbConfiguration, org.openide.util.NbBundle.getMessage(ShowEffPomDiffPanel.class, "ShowEffPomDiffPanel.rbConfiguration.text")); // NOI18N
rbConfiguration.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
rbConfigurationActionPerformed(evt);
}
});
buttonGroup1.add(rbCustom);
org.openide.awt.Mnemonics.setLocalizedText(rbCustom, org.openide.util.NbBundle.getMessage(ShowEffPomDiffPanel.class, "ShowEffPomDiffPanel.rbCustom.text")); // NOI18N
rbCustom.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
rbCustomActionPerformed(evt);
}
});
org.openide.awt.Mnemonics.setLocalizedText(lblProfiles, org.openide.util.NbBundle.getMessage(ShowEffPomDiffPanel.class, "ShowEffPomDiffPanel.lblProfiles.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(lblProperties, org.openide.util.NbBundle.getMessage(ShowEffPomDiffPanel.class, "ShowEffPomDiffPanel.lblProperties.text")); // NOI18N
jScrollPane1.setViewportView(epProperties);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(rbConfiguration)
.addComponent(rbCustom))
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addGap(29, 29, 29)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, 359, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addComponent(lblProfiles)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(txtProfiles))
.addGroup(layout.createSequentialGroup()
.addComponent(lblConfiguration)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(comConfiguration, 0, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addComponent(lblProperties)
.addGap(0, 0, Short.MAX_VALUE)))))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(rbConfiguration)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lblConfiguration)
.addComponent(comConfiguration, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(rbCustom)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lblProfiles)
.addComponent(txtProfiles, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(lblProperties)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 110, Short.MAX_VALUE)
.addContainerGap())
);
}// //GEN-END:initComponents
private void rbConfigurationActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_rbConfigurationActionPerformed
enableFields();
}//GEN-LAST:event_rbConfigurationActionPerformed
private void rbCustomActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_rbCustomActionPerformed
enableFields();
}//GEN-LAST:event_rbCustomActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.ButtonGroup buttonGroup1;
private javax.swing.JComboBox comConfiguration;
private javax.swing.JEditorPane epProperties;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JLabel lblConfiguration;
private javax.swing.JLabel lblProfiles;
private javax.swing.JLabel lblProperties;
private javax.swing.JRadioButton rbConfiguration;
private javax.swing.JRadioButton rbCustom;
private javax.swing.JTextField txtProfiles;
// End of variables declaration//GEN-END:variables
private void enableFields() {
comConfiguration.setEnabled(rbConfiguration.isSelected());
epProperties.setEnabled(rbCustom.isSelected());
txtProfiles.setEnabled(rbCustom.isSelected());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy