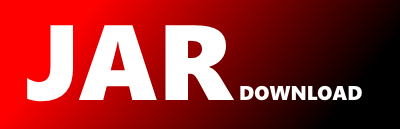
org.netbeans.modules.options.keymap.SpecialkeyPanel Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/*
* SpecialkeyPanel.java
*
* Created on Jan 13, 2009, 12:04:21 PM
*/
package org.netbeans.modules.options.keymap;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import javax.swing.JButton;
import javax.swing.JTextField;
import javax.swing.Popup;
/**
* Little keyboard offering special keys to add to shortcut input textfields
*
* @author Max Sauer
*/
public class SpecialkeyPanel extends javax.swing.JPanel implements ActionListener {
/** parent popup */
private Popupable parent;
private JTextField target;
/** Creates new form SpecialkeyPanel */
public SpecialkeyPanel(final Popupable parent, JTextField target) {
this.parent = parent;
this.target = target;
initComponents();
target.addFocusListener(new FocusAdapter() {
@Override
public void focusLost(FocusEvent e) {
parent.hidePopup();
}
});
downButton.addActionListener(this);
enterButton.addActionListener(this);
escButton.addActionListener(this);
leftButton.addActionListener(this);
rightButton.addActionListener(this);
tabButton.addActionListener(this);
upButton.addActionListener(this);
wheelUpButton.addActionListener(this);
wheelDownButton.addActionListener(this);
mouseButton4.addActionListener(this);
mouseButton5.addActionListener(this);
mouseButton6.addActionListener(this);
mouseButton7.addActionListener(this);
mouseButton8.addActionListener(this);
mouseButton9.addActionListener(this);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
tabButton = new javax.swing.JButton();
escButton = new javax.swing.JButton();
upButton = new javax.swing.JButton();
enterButton = new javax.swing.JButton();
leftButton = new javax.swing.JButton();
downButton = new javax.swing.JButton();
rightButton = new javax.swing.JButton();
wheelUpButton = new javax.swing.JButton();
wheelDownButton = new javax.swing.JButton();
mouseButton4 = new javax.swing.JButton();
mouseButton5 = new javax.swing.JButton();
mouseButton6 = new javax.swing.JButton();
mouseButton7 = new javax.swing.JButton();
mouseButton8 = new javax.swing.JButton();
mouseButton9 = new javax.swing.JButton();
setBorder(javax.swing.BorderFactory.createEtchedBorder());
tabButton.setText("Tab"); // NOI18N
escButton.setFont(new java.awt.Font("Lucida Grande", 0, 9)); // NOI18N
escButton.setText("ESC"); // NOI18N
escButton.setAlignmentY(0.0F);
escButton.setIconTextGap(0);
upButton.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modules/options/keymap/up.png"))); // NOI18N
upButton.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.upButton.text")); // NOI18N
enterButton.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modules/options/keymap/enter.png"))); // NOI18N
enterButton.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.enterButton.text")); // NOI18N
leftButton.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modules/options/keymap/left.png"))); // NOI18N
leftButton.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.leftButton.text_1")); // NOI18N
downButton.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modules/options/keymap/down.png"))); // NOI18N
rightButton.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modules/options/keymap/right.png"))); // NOI18N
rightButton.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.rightButton.text")); // NOI18N
wheelUpButton.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.wheelUpButton.text_1")); // NOI18N
wheelDownButton.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.wheelDownButton.text_1")); // NOI18N
mouseButton4.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.mouseButton4.text")); // NOI18N
mouseButton5.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.mouseButton5.text")); // NOI18N
mouseButton6.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.mouseButton6.text")); // NOI18N
mouseButton7.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.mouseButton7.text")); // NOI18N
mouseButton8.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.mouseButton8.text")); // NOI18N
mouseButton9.setText(org.openide.util.NbBundle.getMessage(SpecialkeyPanel.class, "SpecialkeyPanel.mouseButton9.text")); // NOI18N
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(tabButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(escButton, javax.swing.GroupLayout.PREFERRED_SIZE, 64, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(leftButton, javax.swing.GroupLayout.PREFERRED_SIZE, 50, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(downButton, javax.swing.GroupLayout.PREFERRED_SIZE, 50, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(upButton, javax.swing.GroupLayout.PREFERRED_SIZE, 50, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(rightButton, javax.swing.GroupLayout.PREFERRED_SIZE, 53, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(wheelDownButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addComponent(enterButton, javax.swing.GroupLayout.PREFERRED_SIZE, 53, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(wheelUpButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))))
.addGroup(layout.createSequentialGroup()
.addComponent(mouseButton4, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(mouseButton5, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(mouseButton6, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addComponent(mouseButton7, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(mouseButton8, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(mouseButton9, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)))
.addContainerGap())
);
layout.linkSize(javax.swing.SwingConstants.HORIZONTAL, new java.awt.Component[] {downButton, enterButton, escButton, leftButton, rightButton, upButton});
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(tabButton, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(upButton, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(enterButton, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(escButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(wheelUpButton, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(rightButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(downButton, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(leftButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(wheelDownButton, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(mouseButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(mouseButton7, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(mouseButton8, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(mouseButton9, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(mouseButton6, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(mouseButton5, javax.swing.GroupLayout.PREFERRED_SIZE, 30, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.linkSize(javax.swing.SwingConstants.VERTICAL, new java.awt.Component[] {downButton, enterButton, escButton, leftButton, rightButton, upButton});
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton downButton;
private javax.swing.JButton enterButton;
private javax.swing.JButton escButton;
private javax.swing.JButton leftButton;
private javax.swing.JButton mouseButton4;
private javax.swing.JButton mouseButton5;
private javax.swing.JButton mouseButton6;
private javax.swing.JButton mouseButton7;
private javax.swing.JButton mouseButton8;
private javax.swing.JButton mouseButton9;
private javax.swing.JButton rightButton;
private javax.swing.JButton tabButton;
private javax.swing.JButton upButton;
private javax.swing.JButton wheelDownButton;
private javax.swing.JButton wheelUpButton;
// End of variables declaration//GEN-END:variables
public void actionPerformed(ActionEvent e) {
String text;
final Object source = e.getSource();
//someone clicked a button, hide popup and add text
if ((source instanceof JButton) && parent != null) {
parent.hidePopup();
if (source == upButton) {
text = "UP"; // NOI18N
} else if (source == downButton) {
text = "DOWN"; // NOI18N
} else if (source == leftButton) {
text = "LEFT"; // NOI18N
} else if (source == rightButton) {
text = "RIGHT"; // NOI18N
} else if (source == enterButton) {
text = "ENTER"; // NOI18N
} else if (source == escButton) {
text = "ESCAPE"; // NOI18N
} else if (source == wheelUpButton) {
text = "MOUSE_WHEEL_UP"; // NOI18N
} else if (source == wheelDownButton) {
text = "MOUSE_WHEEL_DOWN"; // NOI18N
} else if (source == mouseButton4) {
text = "MOUSE_BUTTON4"; // NOI18N
} else if (source == mouseButton5) {
text = "MOUSE_BUTTON5"; // NOI18N
} else if (source == mouseButton6) {
text = "MOUSE_BUTTON6"; // NOI18N
} else if (source == mouseButton7) {
text = "MOUSE_BUTTON7"; // NOI18N
} else if (source == mouseButton8) {
text = "MOUSE_BUTTON8"; // NOI18N
} else if (source == mouseButton9) {
text = "MOUSE_BUTTON9"; // NOI18N
} else {
text = ((JButton) source).getText().toUpperCase().replace(" ", "_"); // NOI18N
}
//add the text to target textfield
final String space = " "; //NOI18N
String scText = target.getText();
if (scText.length() == 0 || scText.endsWith(space) || scText.endsWith("+")) { // NOI18N
target.setText(scText + text);
} else {
target.setText(scText + space + text);
}
// set focus
target.requestFocus();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy