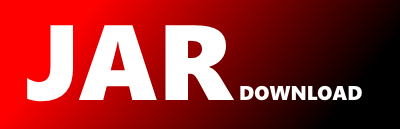
org.netbeans.modules.terminal.nb.PinPanel Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.terminal.nb;
import java.awt.Color;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import org.netbeans.modules.terminal.support.TerminalPinSupport.TerminalCreationDetails;
import org.netbeans.modules.terminal.support.TerminalPinSupport.TerminalDetails;
import org.netbeans.modules.terminal.support.TerminalPinSupport.TerminalPinningDetails;
import org.openide.util.NbBundle;
/**
*
* @author ilia
*/
public class PinPanel extends javax.swing.JPanel {
public static final String PROPERTY_VALID = "valid"; //NOI18N
/**
* Creates new form PinPanel
*/
public PinPanel(TerminalDetails details) {
initComponents();
TerminalCreationDetails creationDetails = details.getCreationDetails();
TerminalPinningDetails pinningDetails = details.getPinningDetails();
envField.setText(creationDetails.getExecEnv());
titleField.setText(pinningDetails.getTitle());
customCheckBox.setSelected(pinningDetails.isCustomTitle());
customStateChanged(pinningDetails.isCustomTitle());
if (pinningDetails.getCwd() != null) {
directoryField.setText(pinningDetails.getCwd());
} else {
directoryField.setText("");
}
directoryField.getDocument().addDocumentListener(new Validator());
}
public String getTitle() {
return titleField.getText();
}
public boolean isCustomTitle() {
return customCheckBox.isSelected();
}
public String getDirectory() {
return directoryField.getText();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
envLabel = new javax.swing.JLabel();
envField = new javax.swing.JTextField();
titleLabel = new javax.swing.JLabel();
titleField = new javax.swing.JTextField();
customCheckBox = new javax.swing.JCheckBox();
directoryLabel = new javax.swing.JLabel();
directoryField = new javax.swing.JTextField();
statusLabel = new javax.swing.JLabel();
envLabel.setLabelFor(envField);
org.openide.awt.Mnemonics.setLocalizedText(envLabel, org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.envLabel.text")); // NOI18N
envField.setEditable(false);
envField.setText(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.envField.text")); // NOI18N
envField.setFocusable(false);
envField.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
envFieldActionPerformed(evt);
}
});
titleLabel.setLabelFor(titleField);
org.openide.awt.Mnemonics.setLocalizedText(titleLabel, org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.titleLabel.text")); // NOI18N
titleField.setText(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.titleField.text")); // NOI18N
customCheckBox.setSelected(true);
org.openide.awt.Mnemonics.setLocalizedText(customCheckBox, org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.customCheckBox.text")); // NOI18N
customCheckBox.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
customCheckBoxActionPerformed(evt);
}
});
directoryLabel.setLabelFor(directoryField);
org.openide.awt.Mnemonics.setLocalizedText(directoryLabel, org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.directoryLabel.text")); // NOI18N
directoryField.setText(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.directoryField.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(statusLabel, org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.statusLabel.text")); // NOI18N
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(statusLabel, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(directoryLabel)
.addComponent(titleLabel)
.addComponent(envLabel))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(envField)
.addGroup(layout.createSequentialGroup()
.addComponent(titleField, javax.swing.GroupLayout.DEFAULT_SIZE, 133, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(customCheckBox))
.addComponent(directoryField))))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(envLabel)
.addComponent(envField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(titleLabel)
.addComponent(titleField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(customCheckBox))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(directoryLabel)
.addComponent(directoryField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(statusLabel, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addContainerGap())
);
envLabel.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.envLabel.AccessibleContext.accessibleDescription")); // NOI18N
envField.getAccessibleContext().setAccessibleName(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.envField.AccessibleContext.accessibleName")); // NOI18N
envField.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.envField.AccessibleContext.accessibleDescription")); // NOI18N
titleLabel.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.titleLabel.AccessibleContext.accessibleDescription")); // NOI18N
titleField.getAccessibleContext().setAccessibleName(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.titleField.AccessibleContext.accessibleName")); // NOI18N
titleField.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.titleField.AccessibleContext.accessibleDescription")); // NOI18N
customCheckBox.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.customCheckBox.AccessibleContext.accessibleDescription")); // NOI18N
directoryLabel.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.directoryLabel.AccessibleContext.accessibleDescription")); // NOI18N
directoryField.getAccessibleContext().setAccessibleName(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.directoryField.AccessibleContext.accessibleName")); // NOI18N
directoryField.getAccessibleContext().setAccessibleDescription(org.openide.util.NbBundle.getMessage(PinPanel.class, "PinPanel.directoryField.AccessibleContext.accessibleDescription")); // NOI18N
}// //GEN-END:initComponents
private void envFieldActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_envFieldActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_envFieldActionPerformed
private void customCheckBoxActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_customCheckBoxActionPerformed
customStateChanged(customCheckBox.isSelected());
}//GEN-LAST:event_customCheckBoxActionPerformed
private void customStateChanged(boolean newState) {
if (newState) {
titleField.setEnabled(true);
titleField.requestFocus();
} else {
titleField.setEnabled(false);
}
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JCheckBox customCheckBox;
private javax.swing.JTextField directoryField;
private javax.swing.JLabel directoryLabel;
private javax.swing.JTextField envField;
private javax.swing.JLabel envLabel;
private javax.swing.JLabel statusLabel;
private javax.swing.JTextField titleField;
private javax.swing.JLabel titleLabel;
// End of variables declaration//GEN-END:variables
public class Validator implements DocumentListener {
private boolean validOld = true;
@Override
public void insertUpdate(DocumentEvent e) {
updateText();
}
@Override
public void removeUpdate(DocumentEvent e) {
updateText();
}
@Override
public void changedUpdate(DocumentEvent e) {
updateText();
}
private boolean isValid() {
String text = directoryField.getText();
// The most basic check for path validity
return text.startsWith("/") || text.startsWith("~") || text.startsWith("$"); //NOI18N
}
private void updateText() {
boolean validNew = isValid();
if (validNew && !validOld) {
firePropertyChange(PROPERTY_VALID, validOld, validNew);
validOld = true;
statusLabel.setText(""); //NOI18N
} else if (!validNew && validOld) {
firePropertyChange(PROPERTY_VALID, validOld, validNew);
validOld = false;
statusLabel.setText(NbBundle.getMessage(PinPanel.class, "ERR_RelativeDir")); //NOI18N
statusLabel.setForeground(Color.RED);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy