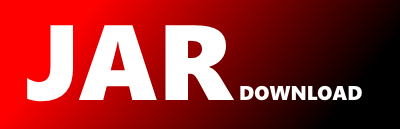
org.nhindirect.xd.soap.SafeThreadData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xd-common Show documentation
Show all versions of xd-common Show documentation
Library for common XD* operations and objects
/*
* Copyright (c) 2010, NHIN Direct Project
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. Neither the name of the the NHIN Direct Project (nhindirect.org)
* nor the names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.nhindirect.xd.soap;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Contains XDR Thread data
* @author Yan Wang
*/
public class SafeThreadData {
public static final String MESSAGE = "message";
public static final String ACTION = "action";
public static final String REPLY = "reply";
public static final String TO = "to";
public static final String RELATESTO = "relatesto";
public static final String THISHOST = "thishost";
public static final String REMOTEHOST = "remotehost";
public static final String PID = "pid";
public static final String FROM = "from";
public static final String DIRECT_TO = "directTo";
public static final String DIRECT_FROM = "directFrom";
public static final String DIRECT_METADATA_LEVEL = "directMetadataLevel";
public static final String REPLY_EMAIL = "replyEmail";
public static final String SUFFIX = "suffix";
public static final String ENDPOINT = "endpoint";
private Long threadId = null;
private String messageId;
private String action;
private String reply;
private String to;
private String relatesTo;
private String thisHost;
private String remoteHost;
private String pid;
private String from;
private String directTo;
private String directFrom;
private String directMetadataLevel;
private String replyEmail;
private String suffix;
private String endpoint;
/**
* Object to contain and relate all Thread data
*/
private static Map> threadMap = new HashMap>();
public SafeThreadData(Long id) {
this.threadId = id;
this.getSafeThreadData();
}
public static SafeThreadData GetThreadInstance(Long id){
SafeThreadData thread = new SafeThreadData(id);
return thread;
}
private void getSafeThreadData(){
Map map;
synchronized(threadMap){
if (threadMap.containsKey(this.threadId)){
map = threadMap.get(this.threadId);
} else{
map = new HashMap();
threadMap.put(this.threadId, map);
}
this.messageId = map.get(MESSAGE);
this.action = map.get(ACTION);
this.reply = map.get(REPLY);
this.to = map.get(TO);
this.relatesTo = map.get(RELATESTO);
this.thisHost = map.get(THISHOST);
this.remoteHost = map.get(REMOTEHOST);
this.pid = map.get(PID);
this.from = map.get(FROM);
this.directTo = map.get(DIRECT_TO);
this.directFrom = map.get(DIRECT_FROM);
this.directMetadataLevel = map.get(DIRECT_METADATA_LEVEL);
this.replyEmail = map.get(REPLY_EMAIL);
this.suffix = map.get(SUFFIX);
this.endpoint = map.get(ENDPOINT);
}
}
public void save(){
Map map= new HashMap();
synchronized(threadMap){
map.put(MESSAGE, this.messageId);
map.put(ACTION, this.action);
map.put(REPLY, this.reply);
map.put(TO, this.to);
map.put(RELATESTO, this.relatesTo);
map.put(THISHOST, this.thisHost);
map.put(REMOTEHOST, this.remoteHost);
map.put(PID, this.pid);
map.put(FROM, this.from);
map.put(DIRECT_TO, this.directTo);
map.put(DIRECT_FROM, this.directFrom);
map.put(DIRECT_METADATA_LEVEL, this.directMetadataLevel);
map.put(REPLY_EMAIL, this.replyEmail);
map.put(SUFFIX, this.suffix);
map.put(ENDPOINT, this.endpoint);
threadMap.put(threadId, map);
}
}
public static void clean(Long id){
synchronized(threadMap){
threadMap.remove(id);
}
}
/**
* Return a read-only copy of the threadMap object.
*
* @return a read-only copy of the threadmap object
*/
protected static Map> getThreadMapView() {
Map> value;
synchronized(threadMap){
value = Collections.unmodifiableMap(threadMap);
}
return value;
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
Map m;
synchronized(threadMap){
m = threadMap.get(this.threadId);
if (m != null) {
StringBuilder sb = new StringBuilder("ThreadData (threadId: " + this.threadId + ")" + "\n");
for (Map.Entry e : m.entrySet()) {
sb.append(" > ").append(e.getKey()).append(": ").append(e.getValue()).append("\n");
}
return sb.toString();
}
}
return "No map found for threadId: " + this.threadId;
}
public Long getThreadId() {
return threadId;
}
public void setThreadId(Long threadId) {
this.threadId = threadId;
}
public String getMessageId() {
return messageId;
}
public void setMessageId(String messageId) {
this.messageId = messageId;
}
public String getAction() {
return action;
}
public void setAction(String action) {
this.action = action;
}
public String getReply() {
return reply;
}
public void setReply(String reply) {
this.reply = reply;
}
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
public String getRelatesTo() {
return relatesTo;
}
public void setRelatesTo(String relatesTo) {
this.relatesTo = relatesTo;
}
public String getThisHost() {
return thisHost;
}
public void setThisHost(String thisHost) {
this.thisHost = thisHost;
}
public String getRemoteHost() {
return remoteHost;
}
public void setRemoteHost(String remoteHost) {
this.remoteHost = remoteHost;
}
public String getPid() {
return pid;
}
public void setPid(String pid) {
this.pid = pid;
}
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public String getDirectTo() {
return directTo;
}
public void setDirectTo(String directTo) {
this.directTo = directTo;
}
public void setDirectTo(List list) {
this.directTo = "";
for (String addr : list){
if (!this.directTo.isEmpty()){
this.directTo += ";";
}
this.directTo += "mailto:" + addr;
}
}
public String getDirectFrom() {
return directFrom;
}
public void setDirectFrom(String directFrom) {
this.directFrom = directFrom;
}
public String getDirectMetadataLevel() {
return directMetadataLevel;
}
public void setDirectMetadataLevel(String directMetadataLevel) {
this.directMetadataLevel = directMetadataLevel;
}
public String getReplyEmail() {
return replyEmail;
}
public void setReplyEmail(String replyEmail) {
this.replyEmail = replyEmail;
}
public String getSuffix() {
return suffix;
}
public void setSuffix(String suffix) {
this.suffix = suffix;
}
public String getEndpoint() {
return endpoint;
}
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
public static Map> getThreadMap() {
return threadMap;
}
public static void setThreadMap(Map> threadMap) {
SafeThreadData.threadMap = threadMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy