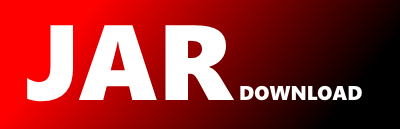
ninja.postoffice.common.MailImpl Maven / Gradle / Ivy
/**
* Copyright (C) 2012-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ninja.postoffice.common;
import ninja.postoffice.Mail;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/**
* Simple implementation of Mail.
*
* Does nothing special. In particular it does NOT validate any content.
*
* @author rbauer
*
*/
public class MailImpl implements Mail {
private String subject;
private String from;
private final Collection replyTos;
private String charset;
private final Map headers;
private final Collection tos;
private final Collection ccs;
private final Collection bccs;
private String bodyText;
private String bodyHtml;
public MailImpl() {
// make sure stuff gets initialized
headers = new HashMap();
tos = new ArrayList();
ccs = new ArrayList();
bccs = new ArrayList();
replyTos = new ArrayList();
}
@Override
public void setSubject(String subject) {
this.subject = subject;
}
@Override
public void setFrom(String from) {
this.from = from;
}
@Override
public void addReplyTo(String... replyTos) {
for (String replyTo : replyTos) {
this.replyTos.add(replyTo);
}
}
@Override
public void setCharset(String charset) {
this.charset = charset;
}
@Override
public void addHeader(String key, String value) {
this.headers.put(key, value);
}
@Override
public void addCc(String... ccs) {
for (String ccRecipient : ccs) {
this.ccs.add(ccRecipient);
}
}
@Override
public void addBcc(String... bccs) {
for (String bccRecipient : bccs) {
this.bccs.add(bccRecipient);
}
}
@Override
public void addTo(String... tos) {
for (String to : tos) {
this.tos.add(to);
}
}
@Override
public String getSubject() {
return subject;
}
@Override
public String getFrom() {
return from;
}
@Override
public Collection getReplyTo() {
return replyTos;
}
@Override
public String getCharset() {
return charset;
}
@Override
public Map getHeaders() {
return headers;
}
@Override
public Collection getCcs() {
return ccs;
}
@Override
public Collection getBccs() {
return bccs;
}
@Override
public Collection getTos() {
return tos;
}
@Override
public void setBodyHtml(String bodyHtml) {
this.bodyHtml = bodyHtml;
}
@Override
public String getBodyHtml() {
return bodyHtml;
}
@Override
public void setBodyText(String bodyText) {
this.bodyText = bodyText;
}
@Override
public String getBodyText() {
return this.bodyText;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy