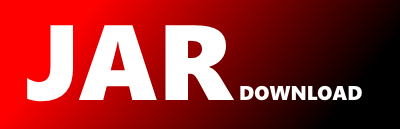
ninja.ControllerMethods Maven / Gradle / Ivy
/**
* Copyright (C) the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ninja;
import java.io.Serializable;
/**
* Functional interfaces for Ninja controller methods accepting up to X
* number of arguments with type inference.
*/
public class ControllerMethods {
/**
* Marker interface that all functional interfaces will extend. Useful for
* simple validation an object is a ControllerMethod.
*/
static public interface ControllerMethod extends Serializable { }
@FunctionalInterface
static public interface ControllerMethod0 extends ControllerMethod {
Result apply() throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod1 extends ControllerMethod {
Result apply(A a) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod2 extends ControllerMethod {
Result apply(A a, B b) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod3 extends ControllerMethod {
Result apply(A a, B b, C c) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod4 extends ControllerMethod {
Result apply(A a, B b, C c, D d) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod5 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod6 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod7 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod8 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod9 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod10 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i, J j) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod11 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i, J j, K k) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod12 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i, J j, K k, L l) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod13 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i, J j, K k, L l, M m) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod14 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i, J j, K k, L l, M m, N n) throws Exception;
}
@FunctionalInterface
static public interface ControllerMethod15 extends ControllerMethod {
Result apply(A a, B b, C c, D d, E e, F f, G g, H h, I i, J j, K k, L l, M m, N n, O o) throws Exception;
}
// if you need more than 15 arguments then we recommend using the
// legacy Class, methodName strategy
// helper methods to allow classes to accept `ControllerMethod` but still
// have the compiler create the correct functional method under-the-hood
static public ControllerMethod0 of(ControllerMethod0 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod1 of(ControllerMethod1 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod2 of(ControllerMethod2 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod3 of(ControllerMethod3 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod4 of(ControllerMethod4 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod5 of(ControllerMethod5 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod6 of(ControllerMethod6 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod7 of(ControllerMethod7 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod8 of(ControllerMethod8 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod9 of(ControllerMethod9 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod10 of(ControllerMethod10 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod11 of(ControllerMethod11 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod12 of(ControllerMethod12 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod13 of(ControllerMethod13 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod14 of(ControllerMethod14 functionalMethod) {
return functionalMethod;
}
static public ControllerMethod15 of(ControllerMethod15 functionalMethod) {
return functionalMethod;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy