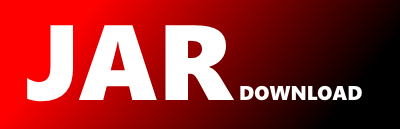
ninja.NinjaScheduler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ninja-quartz-scheduler-module Show documentation
Show all versions of ninja-quartz-scheduler-module Show documentation
This is an easily plugable module for the Ninja web framework to work with Quartz Scheduler.
The newest version!
package ninja;
import static org.quartz.CronScheduleBuilder.cronSchedule;
import static org.quartz.JobBuilder.newJob;
import static org.quartz.TriggerBuilder.newTrigger;
import ninja.lifecycle.Start;
import org.quartz.Job;
import org.quartz.JobDetail;
import org.quartz.Scheduler;
import org.quartz.SchedulerException;
import org.quartz.SchedulerFactory;
import org.quartz.Trigger;
import org.quartz.impl.StdSchedulerFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import quartz.QuartzJobFactory;
import com.google.common.base.Preconditions;
import com.google.inject.Inject;
import com.google.inject.Singleton;
/**
*
* @author svenkubiak
*
*/
@Singleton
public class NinjaScheduler {
private static final Logger LOG = LoggerFactory.getLogger(NinjaScheduler.class);
private Scheduler scheduler = null;
@Inject
private QuartzJobFactory quartzJobFactory;
@Start(order=80)
public void init() {
SchedulerFactory schedulerFactory = new StdSchedulerFactory();
try {
this.scheduler = schedulerFactory.getScheduler();
this.scheduler.setJobFactory(quartzJobFactory);
} catch (SchedulerException e) {
LOG.error("Failed to get scheduler from schedulerFactory", e);
}
}
/**
* Starts the scheduler if initialized
*/
public void start() {
Preconditions.checkNotNull(this.scheduler);
try {
this.scheduler.start();
if (this.scheduler.isStarted()) {
LOG.info("Successfully started quartz scheduler");
} else {
LOG.error("Scheduler is not started");
}
} catch (SchedulerException e) {
LOG.error("Failed to start schedulder", e);
}
}
/**
* Stops the scheduler if started
*/
public void shutdown() {
Preconditions.checkNotNull(this.scheduler);
try {
this.scheduler.shutdown();
if (this.scheduler.isShutdown()) {
LOG.info("Successfully shutdown quartz scheduler");
} else {
LOG.error("Failed to shutdown scheduler");
}
} catch (SchedulerException e) {
LOG.error("Failed to shutdown schedulder", e);
}
}
/**
* Adds a job to the schedulder based on a JobDetail and a Trigger
*
* @param jobDetail The JobDetail
* @param trigger The Trigger
*/
public void schedule(JobDetail jobDetail, Trigger trigger) {
Preconditions.checkNotNull(this.scheduler);
try {
this.scheduler.scheduleJob(jobDetail, trigger);
} catch (SchedulerException e) {
LOG.error("Failed to schedule new job", e);
}
}
/**
* Convinent method for creating a new Trigger based on
* a cron expression
*
* @param identity The identity in quartz for the trigger
* @param cronExpression The cron expression (e.g. 0 4 * * * ?)
* @param triggerGroupName The name of the trigger grop in quartz
*
* @return A new Trigger object
*/
public Trigger getTrigger(String identity, String cronExpression, String triggerGroupName) {
Preconditions.checkNotNull(identity);
Preconditions.checkNotNull(cronExpression);
Preconditions.checkNotNull(triggerGroupName);
return newTrigger()
.withIdentity(identity, triggerGroupName)
.withSchedule(cronSchedule(cronExpression))
.build();
}
/**
* Convinient method for creating a new JobDetail
*
* @param clazz The job class to execute
* @param identity The identity in quartz for the job
* @param jobGroupName The name of the job group in quartz
*
* @return A new JobDetail object for scheduling
*/
public JobDetail getJobDetail(Class clazz, String identity, String jobGroupName) {
Preconditions.checkNotNull(clazz);
Preconditions.checkNotNull(identity);
Preconditions.checkNotNull(jobGroupName);
return newJob(clazz)
.withIdentity(identity, jobGroupName)
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy