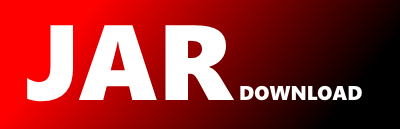
org.nlpcraft.examples.misc.apixu.beans.Current Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nlpcraft Show documentation
Show all versions of nlpcraft Show documentation
An API to convert natural language into actions.
/*
* "Commons Clause" License, https://commonsclause.com/
*
* The Software is provided to you by the Licensor under the License,
* as defined below, subject to the following condition.
*
* Without limiting other conditions in the License, the grant of rights
* under the License will not include, and the License does not grant to
* you, the right to Sell the Software.
*
* For purposes of the foregoing, "Sell" means practicing any or all of
* the rights granted to you under the License to provide to third parties,
* for a fee or other consideration (including without limitation fees for
* hosting or consulting/support services related to the Software), a
* product or service whose value derives, entirely or substantially, from
* the functionality of the Software. Any license notice or attribution
* required by the License must also include this Commons Clause License
* Condition notice.
*
* Software: NLPCraft
* License: Apache 2.0, https://www.apache.org/licenses/LICENSE-2.0
* Licensor: Copyright (C) 2018 DataLingvo, Inc. https://www.datalingvo.com
*
* _ ____ ______ ______
* / | / / /___ / ____/________ _/ __/ /_
* / |/ / / __ \/ / / ___/ __ `/ /_/ __/
* / /| / / /_/ / /___/ / / /_/ / __/ /_
* /_/ |_/_/ .___/\____/_/ \__,_/_/ \__/
* /_/
*/
package org.nlpcraft.examples.misc.apixu.beans;
import com.google.gson.annotations.SerializedName;
/**
* REST parsing bean.
*/
public class Current {
@SerializedName("last_updated") private String lastUpdated;
@SerializedName("last_updated_epoch") private int lastUpdatedEpoch;
@SerializedName("temp_c") private Double tempC;
@SerializedName("temp_f") private Double tempF;
@SerializedName("feelslike_c") private Double feelsLikeC;
@SerializedName("feelslike_f") private Double feelsLikeF;
private Condition condition;
@SerializedName("wind_mph") private Double windMph;
@SerializedName("wind_kph") private Double windKph;
@SerializedName("wind_dir") private String windDir;
@SerializedName("wind_degree") private int windDegree;
@SerializedName("pressure_mb") private Double pressureMb;
@SerializedName("pressure_in") private Double pressureIn;
@SerializedName("precip_mm") private Double precipMm;
@SerializedName("precip_in") private Double precipIn;
private int humidity;
private int cloud;
@SerializedName("is_day") private int isDay;
public String getLastUpdated() {
return lastUpdated;
}
public void setLastUpdated(String lastUpdated) {
this.lastUpdated = lastUpdated;
}
public int getLastUpdatedEpoch() {
return lastUpdatedEpoch;
}
public void setLastUpdatedEpoch(int lastUpdatedEpoch) {
this.lastUpdatedEpoch = lastUpdatedEpoch;
}
public Double getTempC() {
return tempC;
}
public void setTempC(Double tempC) {
this.tempC = tempC;
}
public Double getTempF() {
return tempF;
}
public void setTempF(Double tempF) {
this.tempF = tempF;
}
public Double getFeelsLikeC() {
return feelsLikeC;
}
public void setFeelsLikeC(Double feelsLikeC) {
this.feelsLikeC = feelsLikeC;
}
public Double getFeelsLikeF() {
return feelsLikeF;
}
public void setFeelsLikeF(Double feelsLikeF) {
this.feelsLikeF = feelsLikeF;
}
public Condition getCondition() {
return condition;
}
public void setCondition(Condition condition) {
this.condition = condition;
}
public Double getWindMph() {
return windMph;
}
public void setWindMph(Double windMph) {
this.windMph = windMph;
}
public Double getWindKph() {
return windKph;
}
public void setWindKph(Double windKph) {
this.windKph = windKph;
}
public String getWindDir() {
return windDir;
}
public void setWindDir(String windDir) {
this.windDir = windDir;
}
public int getWindDegree() {
return windDegree;
}
public void setWindDegree(int windDegree) {
this.windDegree = windDegree;
}
public Double getPressureMb() {
return pressureMb;
}
public void setPressureMb(Double pressureMb) {
this.pressureMb = pressureMb;
}
public Double getPressureIn() {
return pressureIn;
}
public void setPressureIn(Double pressureIn) {
this.pressureIn = pressureIn;
}
public Double getPrecipMm() {
return precipMm;
}
public void setPrecipMm(Double precipMm) {
this.precipMm = precipMm;
}
public Double getPrecipIn() {
return precipIn;
}
public void setPrecipIn(Double precipIn) {
this.precipIn = precipIn;
}
public int getHumidity() {
return humidity;
}
public void setHumidity(int humidity) {
this.humidity = humidity;
}
public int getCloud() {
return cloud;
}
public void setCloud(int cloud) {
this.cloud = cloud;
}
public int getIsDay() {
return isDay;
}
public void setIsDay(int isDay) {
this.isDay = isDay;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy