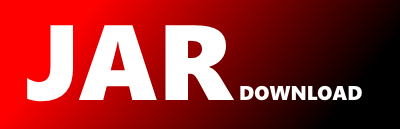
org.nlpub.watset.graph.SenseInduction Maven / Gradle / Ivy
/*
* Copyright 2018 Dmitry Ustalov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.nlpub.watset.graph;
import org.jgrapht.Graph;
import org.jgrapht.alg.interfaces.ClusteringAlgorithm;
import org.nlpub.watset.util.Neighbors;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static java.util.Objects.requireNonNull;
import static org.jgrapht.GraphTests.requireUndirected;
/**
* A simple graph-based word sense induction approach that clusters node neighborhoods.
*
* @param the type of nodes in the graph
* @param the type of edges in the graph
* @see Biemann (TextGraphs-1)
* @see Dorow & Widdows (EACL '03)
* @see Ustalov et al. (COLI 45:3)
*/
public class SenseInduction {
/**
* The graph.
*/
protected final Graph graph;
/**
* The local clustering algorithm supplier.
*/
protected final ClusteringAlgorithmBuilder local;
/**
* Create an instance of {@code SenseInduction}.
*
* @param graph the graph
* @param local the neighborhood clustering algorithm supplier
*/
public SenseInduction(Graph graph, ClusteringAlgorithmBuilder local) {
this.graph = requireUndirected(graph);
this.local = requireNonNull(local);
}
/**
* Get the induced sense clusters.
*
* @param target the target node
* @return a map of senses to their contexts
*/
public ClusteringAlgorithm.Clustering clustering(V target) {
final var ego = Neighbors.graph(graph, requireNonNull(target));
final var clustering = local.apply(ego);
return clustering.getClustering();
}
/**
* Get the induced senses and their non-disambiguated contexts.
*
* @param target the target node
* @return maps of senses to their contexts
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy