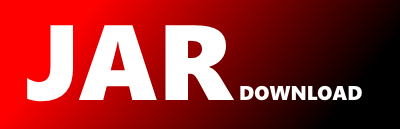
org.nlpub.watset.eval.NormalizedModifiedPurity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of watset Show documentation
Show all versions of watset Show documentation
An open source implementation of the Watset algorithm for fuzzy graph clustering.
/*
* Copyright 2018 Dmitry Ustalov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.nlpub.watset.eval;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import static java.util.Objects.requireNonNull;
import static java.util.function.Function.identity;
/**
* Normalized modified purity evaluation measure for overlapping clustering.
*
* Please be especially careful with the {@code hashCode} and {@code equals} methods of the elements.
*
* @param the type of cluster elements
* @see Evaluation of clustering
* @see Kawahara et al. (ACL 2014)
* @see Ustalov et al. (COLI 45:3)
*/
public class NormalizedModifiedPurity {
/**
* Transform a collection of clusters into a collection of weighted cluster elements.
*
* @param clusters the collection of clusters
* @param the type of cluster elements
* @return a collection of weighted cluster elements
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy