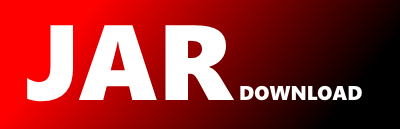
org.nlpub.watset.util.Maximizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of watset Show documentation
Show all versions of watset Show documentation
An open source implementation of the Watset algorithm for fuzzy graph clustering.
The newest version!
/*
* Copyright 2018 Dmitry Ustalov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.nlpub.watset.util;
import java.util.ArrayList;
import java.util.Optional;
import java.util.Random;
import java.util.function.Function;
import java.util.function.Predicate;
import static java.util.Objects.isNull;
/**
* Utilities for searching arguments of the maxima of the function.
*/
public final class Maximizer {
private Maximizer() {
throw new UnsupportedOperationException("This is a utility class and cannot be instantiated");
}
/**
* A predicate that is always true.
*
* @param the type
* @return the absolute truth
*/
public static Predicate alwaysTrue() {
return (o) -> true;
}
/**
* A predicate that is always false.
*
* @param the type
* @return the absolute non-truth
*/
public static Predicate alwaysFalse() {
return (o) -> false;
}
/**
* Find the first argument of the maximum (argmax) of the function.
*
* @param iterable the finite iterator
* @param checker the predicate that evaluates the suitability of the argument
* @param scorer the scoring function
* @param the argument type
* @param the score type
* @return a non-empty optional that contains the first found argmax, otherwise the empty one
*/
public static > Optional argmax(Iterable iterable, Predicate checker, Function scorer) {
V result = null;
S score = null;
for (final var current : iterable) {
if (!checker.test(current)) continue;
final var currentScore = scorer.apply(current);
if (isNull(score) || (currentScore.compareTo(score) > 0)) {
result = current;
score = currentScore;
}
}
return Optional.ofNullable(result);
}
/**
* Find the first argument of the maximum (argmax) of the function.
*
* @param iterable the finite iterator
* @param scorer the scoring function
* @param the argument type
* @param the score type
* @return a non-empty optional that contains the first found argmax, otherwise the empty one
* @see #argmax(Iterable, Predicate, Function)
*/
public static > Optional argmax(Iterable iterable, Function scorer) {
return argmax(iterable, alwaysTrue(), scorer);
}
/**
* Find the arguments of the maxima (argmax) of the function and randomly choose any of them.
*
* @param iterable the finite iterator
* @param scorer the scoring function
* @param random the random number generator
* @param the argument type
* @param the score type
* @return a non-empty optional that contains the randomly chosen argmax, otherwise the empty one
*/
public static > Optional argrandmax(Iterable iterable, Function scorer, Random random) {
final var results = new ArrayList();
S score = null;
for (final var current : iterable) {
final var currentScore = scorer.apply(current);
final var compare = isNull(score) ? 1 : currentScore.compareTo(score);
if (compare > 0) {
results.clear();
score = currentScore;
}
if (compare >= 0) {
results.add(current);
}
}
return results.isEmpty() ? Optional.empty() : Optional.of(results.get(random.nextInt(results.size())));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy