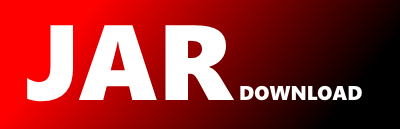
org.nmdp.ngs.hml.jaxb.ConsensusSequenceBlock Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.10-b140310.1920
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.05.15 at 04:24:12 PM CDT
//
package org.nmdp.ngs.hml.jaxb;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAnyAttribute;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlIDREF;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.namespace.QName;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://schemas.nmdp.org/spec/hml/1.0.1}sequence"/>
* <element ref="{http://schemas.nmdp.org/spec/hml/1.0.1}variant" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://schemas.nmdp.org/spec/hml/1.0.1}sequence-quality" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="reference-sequence-id" use="required" type="{http://www.w3.org/2001/XMLSchema}IDREF" />
* <attribute name="start" type="{http://schemas.nmdp.org/spec/hml/1.0.1}position-type" />
* <attribute name="end" type="{http://schemas.nmdp.org/spec/hml/1.0.1}position-type" />
* <attribute name="strand">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="-1"/>
* <enumeration value="1"/>
* <enumeration value="+"/>
* <enumeration value="-"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="phasing-group" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="phase-set" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="continuity" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* <attribute name="expected-copy-number">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}int">
* <minInclusive value="0"/>
* </restriction>
* </simpleType>
* </attribute>
* <attribute name="description">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <minLength value="1"/>
* </restriction>
* </simpleType>
* </attribute>
* <anyAttribute/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"sequence",
"variant",
"sequenceQuality"
})
@XmlRootElement(name = "consensus-sequence-block")
public class ConsensusSequenceBlock {
@XmlElement(required = true)
protected Sequence sequence;
protected List variant;
@XmlElement(name = "sequence-quality")
protected List sequenceQuality;
@XmlAttribute(name = "reference-sequence-id", required = true)
@XmlIDREF
@XmlSchemaType(name = "IDREF")
protected Object referenceSequenceId;
@XmlAttribute(name = "start")
protected Long start;
@XmlAttribute(name = "end")
protected Long end;
@XmlAttribute(name = "strand")
protected String strand;
@XmlAttribute(name = "phasing-group")
protected String phasingGroup;
@XmlAttribute(name = "phase-set")
protected String phaseSet;
@XmlAttribute(name = "continuity")
protected Boolean continuity;
@XmlAttribute(name = "expected-copy-number")
protected Integer expectedCopyNumber;
@XmlAttribute(name = "description")
protected String description;
@XmlAnyAttribute
private Map otherAttributes = new HashMap();
/**
* Gets the value of the sequence property.
*
* @return
* possible object is
* {@link Sequence }
*
*/
public Sequence getSequence() {
return sequence;
}
/**
* Sets the value of the sequence property.
*
* @param value
* allowed object is
* {@link Sequence }
*
*/
public void setSequence(Sequence value) {
this.sequence = value;
}
/**
* Gets the value of the variant property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the variant property.
*
*
* For example, to add a new item, do as follows:
*
* getVariant().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Variant }
*
*
*/
public List getVariant() {
if (variant == null) {
variant = new ArrayList();
}
return this.variant;
}
/**
* Gets the value of the sequenceQuality property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sequenceQuality property.
*
*
* For example, to add a new item, do as follows:
*
* getSequenceQuality().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SequenceQuality }
*
*
*/
public List getSequenceQuality() {
if (sequenceQuality == null) {
sequenceQuality = new ArrayList();
}
return this.sequenceQuality;
}
/**
* Gets the value of the referenceSequenceId property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getReferenceSequenceId() {
return referenceSequenceId;
}
/**
* Sets the value of the referenceSequenceId property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setReferenceSequenceId(Object value) {
this.referenceSequenceId = value;
}
/**
* Gets the value of the start property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getStart() {
return start;
}
/**
* Sets the value of the start property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setStart(Long value) {
this.start = value;
}
/**
* Gets the value of the end property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getEnd() {
return end;
}
/**
* Sets the value of the end property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setEnd(Long value) {
this.end = value;
}
/**
* Gets the value of the strand property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStrand() {
return strand;
}
/**
* Sets the value of the strand property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStrand(String value) {
this.strand = value;
}
/**
* Gets the value of the phasingGroup property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhasingGroup() {
return phasingGroup;
}
/**
* Sets the value of the phasingGroup property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPhasingGroup(String value) {
this.phasingGroup = value;
}
/**
* Gets the value of the phaseSet property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhaseSet() {
return phaseSet;
}
/**
* Sets the value of the phaseSet property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPhaseSet(String value) {
this.phaseSet = value;
}
/**
* Gets the value of the continuity property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isContinuity() {
return continuity;
}
/**
* Sets the value of the continuity property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setContinuity(Boolean value) {
this.continuity = value;
}
/**
* Gets the value of the expectedCopyNumber property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getExpectedCopyNumber() {
return expectedCopyNumber;
}
/**
* Sets the value of the expectedCopyNumber property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setExpectedCopyNumber(Integer value) {
this.expectedCopyNumber = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets a map that contains attributes that aren't bound to any typed property on this class.
*
*
* the map is keyed by the name of the attribute and
* the value is the string value of the attribute.
*
* the map returned by this method is live, and you can add new attribute
* by updating the map directly. Because of this design, there's no setter.
*
*
* @return
* always non-null
*/
public Map getOtherAttributes() {
return otherAttributes;
}
}