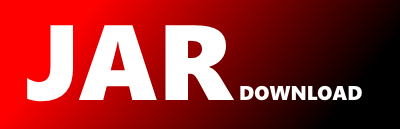
graphql.solon.annotation.BaseSchemaMappingAnnoHandler Maven / Gradle / Ivy
package graphql.solon.annotation;
import graphql.schema.DataFetcher;
import graphql.solon.fetcher.DataFetcherWrap;
import graphql.solon.fetcher.SchemaMappingDataFetcher;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.LinkedList;
import java.util.List;
import org.noear.solon.core.AppContext;
import org.noear.solon.core.BeanExtractor;
import org.noear.solon.core.BeanWrap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* @author fuzi1996
* @since 2.3
*/
public abstract class BaseSchemaMappingAnnoHandler implements
BeanExtractor {
private static Logger log = LoggerFactory.getLogger(BaseSchemaMappingAnnoHandler.class);
protected final List wrapList;
protected final AppContext context;
public BaseSchemaMappingAnnoHandler(AppContext context) {
this.wrapList = new LinkedList<>();
this.context = context;
}
public List getWrapList() {
return wrapList;
}
@Override
public void doExtract(BeanWrap wrap, Method method,
T schemaMapping) throws Throwable {
String typeName = this.getTypeName(wrap, method, schemaMapping);
String fieldName = this.getFieldName(wrap, method, schemaMapping);
DataFetcher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy