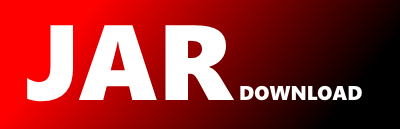
graphql.solon.support.DefaultExecutionGraphQlResponse Maven / Gradle / Ivy
/*
* Copyright 2002-2022 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package graphql.solon.support;
import graphql.ErrorClassification;
import graphql.ExecutionInput;
import graphql.ExecutionResult;
import graphql.ExecutionResultImpl;
import graphql.GraphQLError;
import graphql.language.SourceLocation;
import graphql.solon.util.Assert;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.noear.solon.lang.Nullable;
/**
* {@link GraphQlResponse} for server use that wraps the {@link ExecutionResult}
* returned from {@link graphql.GraphQL} and also exposes the actual
* {@link ExecutionInput} instance passed into it.
*
* @author Rossen Stoyanchev
* @since 1.0.0
*/
public class DefaultExecutionGraphQlResponse extends AbstractGraphQlResponse implements
ExecutionGraphQlResponse {
private final ExecutionInput input;
private final ExecutionResult result;
/**
* Constructor to create initial instance.
*/
public DefaultExecutionGraphQlResponse(ExecutionInput input, ExecutionResult result) {
Assert.notNull(input, "ExecutionInput is required");
Assert.notNull(result, "ExecutionResult is required");
this.input = input;
this.result = result;
}
/**
* Constructor to re-wrap from transport specific subclass.
*/
protected DefaultExecutionGraphQlResponse(ExecutionGraphQlResponse response) {
this(response.getExecutionInput(), response.getExecutionResult());
}
@Override
public ExecutionInput getExecutionInput() {
return this.input;
}
@Override
public ExecutionResult getExecutionResult() {
return this.result;
}
@Override
public boolean isValid() {
return (this.result.isDataPresent() && this.result.getData() != null);
}
@Nullable
@Override
public T getData() {
return this.result.getData();
}
@Override
public List getErrors() {
return this.result.getErrors().stream().map(Error::new).collect(Collectors.toList());
}
@Override
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy