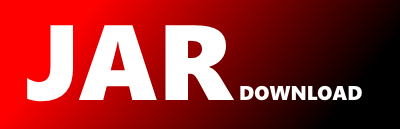
org.smartboot.http.common.io.BufferOutputStream Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2017-2021, org.smartboot. All rights reserved.
* project name: smart-http
* file name: BufferOutputStream.java
* Date: 2021-02-07
* Author: sandao ([email protected])
******************************************************************************/
package org.smartboot.http.common.io;
import org.smartboot.http.common.Reset;
import org.smartboot.http.common.utils.Constant;
import org.smartboot.socket.transport.WriteBuffer;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Consumer;
import java.util.function.Supplier;
/**
* @author 三刀
* @version V1.0 , 2020/12/7
*/
public abstract class BufferOutputStream extends OutputStream implements Reset {
protected final WriteBuffer writeBuffer;
protected boolean committed = false;
protected boolean chunkedSupport = true;
/**
* 当前流是否完结
*/
protected boolean closed = false;
protected long remaining = -1;
private Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy