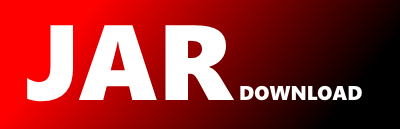
org.smartboot.http.common.io.ChunkedInputStream Maven / Gradle / Ivy
package org.smartboot.http.common.io;
import org.smartboot.http.common.enums.HttpStatus;
import org.smartboot.http.common.exception.HttpException;
import org.smartboot.http.common.utils.Constant;
import org.smartboot.socket.transport.AioSession;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Consumer;
/**
* @author 三刀([email protected])
* @version V1.0 , 2022/12/6
*/
public class ChunkedInputStream extends BodyInputStream {
private final ByteArrayOutputStream buffer = new ByteArrayOutputStream(8);
private Map trailerFields;
/**
* 剩余可读字节数
*/
private long remainingThreshold;
private final Consumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy