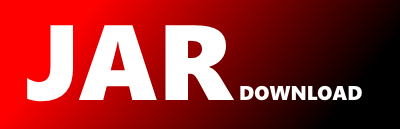
org.nosceon.connellite.Poller Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.nosceon.connellite;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Properties;
import org.nosceon.connellite.PropertiesSupplier.Checkpoint;
/**
* @author Johan Siebens
*/
class Poller {
private static class State {
private Checkpoint checkpoint = Checkpoint.INITIAL_CHECKPOINT;
private PropertiesSupplier supplier;
public State(PropertiesSupplier supplier) {
this.supplier = supplier;
}
public PollResult update() throws Exception {
PollResult s = supplier.poll(checkpoint);
this.checkpoint = s.checkpoint();
return s;
}
}
protected static Node createNode(Collection> sources) {
return createNode(resolvingProperties(sources));
}
private static Node createNode(ResolvingProperties cp) {
Node newNode = Node.newNode();
for (String key : cp.keys()) {
newNode.add(key, cp.getProperty(key));
}
return newNode;
}
private static ResolvingProperties newResolvedProperties(Properties source, ResolvingProperties parent) {
return new ResolvingProperties(source, parent);
}
private static ResolvingProperties resolvingProperties(Collection> suppliers) {
ResolvingProperties cp = rootResolvedProperties();
for (PollResult source : suppliers) {
cp = newResolvedProperties(source.get(), cp);
}
return cp;
}
private static ResolvingProperties rootResolvedProperties() {
return new ResolvingProperties();
}
private Node node = Node.newNode();
private List states = new ArrayList<>();
public Poller(List suppliers) {
for (PropertiesSupplier supplier : suppliers) {
states.add(new State(supplier));
}
}
public PollResult poll() {
try {
boolean hasChanges = false;
List> sources = new ArrayList<>();
for (State state : states) {
PollResult s = state.update();
sources.add(s);
hasChanges = hasChanges || s.hasChanges();
}
if (hasChanges) {
node = createNode(sources);
}
return new PollResult(Checkpoint.INITIAL_CHECKPOINT, hasChanges, node);
}
catch (ConfigurationException e) {
throw e;
}
catch (Exception e) {
throw new ConfigurationException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy