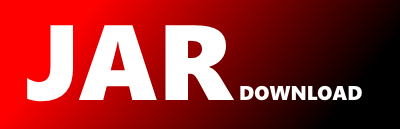
org.nqcx.tree.TreeBuilder Maven / Gradle / Ivy
/*
* Copyright 2012-2013 nqcx.org All right reserved. This software is the
* confidential and proprietary information of nqcx.org ("Confidential
* Information"). You shall not disclose such Confidential Information and shall
* use it only in accordance with the terms of the license agreement you entered
* into with nqcx.org.
*/
package org.nqcx.tree;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* tree builder
*
* @author huangbaoguang 2013-3-27 下午5:02:48
*
* @param
*/
public class TreeBuilder implements Serializable {
private static final long serialVersionUID = -3889238862426715948L;
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy