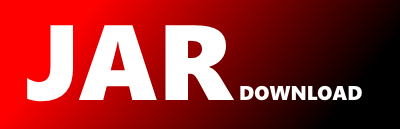
org.nuiton.eugene.models.object.xml.ObjectModelAttributeImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eugene Show documentation
Show all versions of eugene Show documentation
Efficient Universal Generator.
/*
* #%L
* EUGene :: EUGene
* %%
* Copyright (C) 2004 - 2010 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.eugene.models.object.xml;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Iterables;
import com.google.common.collect.Sets;
import org.apache.commons.lang3.StringUtils;
import org.nuiton.eugene.EugeneStereoTypes;
import org.nuiton.eugene.GeneratorUtil;
import org.nuiton.eugene.models.object.ObjectModelAttribute;
import org.nuiton.eugene.models.object.ObjectModelClass;
import org.nuiton.eugene.models.object.ObjectModelClassifier;
import org.nuiton.eugene.models.object.ObjectModelJavaModifier;
import org.nuiton.eugene.models.object.ObjectModelModifier;
import org.nuiton.eugene.models.object.ObjectModelUMLModifier;
import java.util.Set;
/**
* ObjectModelAttributeImpl.
*
* Created: 14 janv. 2004
*
* @author Cédric Pineau - [email protected] Copyright Code Lutin
*/
public class ObjectModelAttributeImpl extends ObjectModelParameterImpl
implements ObjectModelAttribute {
public static final String ATTRIBUTE_TYPE_AGGREGATE = "aggregate";
public static final String ATTRIBUTE_TYPE_SHARED = "shared";
public static final String ATTRIBUTE_TYPE_COMPOSITE = "composite";
public static final ObjectModelJavaModifier DEFAULT_VISIBILITY = ObjectModelJavaModifier.PROTECTED;
protected String reverseAttributeName;
protected int reverseMaxMultiplicity = -1;
protected ObjectModelClassifier reference;
protected String associationClassName;
private static Set authorizedModifiers;
public ObjectModelAttributeImpl() {
addModifier(ObjectModelUMLModifier.NAVIGABLE); // Navigable by default
}
public void postInit() {
if (name == null) {
name = GeneratorUtil.toLowerCaseFirstLetter(GeneratorUtil.getClassNameFromQualifiedName(type));
}
super.postInit();
}
public void setReverseAttributeName(String reverseAttributeName) {
this.reverseAttributeName = reverseAttributeName;
}
public void setAssociationType(String associationType) {
removeModifiers(ObjectModelUMLModifier.associationTypes);
if (ATTRIBUTE_TYPE_SHARED.equals(associationType) || ATTRIBUTE_TYPE_AGGREGATE.equals(associationType)) {
addModifier(ObjectModelUMLModifier.AGGREGATE);
} else if (ATTRIBUTE_TYPE_COMPOSITE.equals(associationType)) {
addModifier(ObjectModelUMLModifier.COMPOSITE);
} else {
throw new IllegalArgumentException("Unexpected association type: " + associationType);
}
}
public void setReverseMaxMultiplicity(int reverseMaxMultiplicity) {
this.reverseMaxMultiplicity = reverseMaxMultiplicity;
}
public void setVisibility(String visibility) {
ObjectModelModifier modifier = ObjectModelJavaModifier.fromVisibility(visibility);
removeModifiers(ObjectModelJavaModifier.visibilityModifiers);
if (modifier == null) {
modifier = DEFAULT_VISIBILITY; // default visibility
}
addModifier(modifier);
}
public void setFinal(boolean isFinal) {
addOrRemoveModifier(ObjectModelJavaModifier.FINAL, isFinal);
}
public void setTransient(boolean isTransient) {
addOrRemoveModifier(ObjectModelJavaModifier.TRANSIENT, isTransient);
}
public void setStatic(boolean isStatic) {
super.setStatic(isStatic);
}
@Override
protected Set getAuthorizedModifiers() {
if (authorizedModifiers == null) {
// http://docs.oracle.com/javase/specs/jls/se7/html/jls-8.html#jls-8.3.1
// static final transient volatile
Set modifiers = Sets.newHashSet(
(ObjectModelModifier) ObjectModelJavaModifier.STATIC, // Force cast because of generics limitation
ObjectModelJavaModifier.FINAL,
ObjectModelJavaModifier.TRANSIENT,
ObjectModelJavaModifier.VOLATILE,
ObjectModelUMLModifier.AGGREGATE,
ObjectModelUMLModifier.COMPOSITE,
ObjectModelUMLModifier.NAVIGABLE,
ObjectModelUMLModifier.ORDERED,
ObjectModelUMLModifier.UNIQUE
);
Iterables.addAll(modifiers, ObjectModelJavaModifier.visibilityModifiers);
authorizedModifiers = ImmutableSet.copyOf(modifiers);
}
return authorizedModifiers;
}
public void setNavigable(boolean navigable) {
addOrRemoveModifier(ObjectModelUMLModifier.NAVIGABLE, navigable);
}
@Override
public void setUnique(boolean isUnique) {
super.setUnique(isUnique);
}
/**
* Returns whether this attribute is an aggregate or not.
*
* @return a boolean indicating whether this attribute is an aggregate or
* not.
*/
@Override
public boolean isAggregate() {
return modifiers.contains(ObjectModelUMLModifier.AGGREGATE);
}
/**
* Returns whether this attribute is an composite or not.
*
* @return a boolean indicating whether this attribute is an composite or
* not.
*/
@Override
public boolean isComposite() {
return modifiers.contains(ObjectModelUMLModifier.COMPOSITE);
}
@Override
public String getVisibility() {
String visibility = DEFAULT_VISIBILITY.toString(); // default
if (modifiers.contains(ObjectModelJavaModifier.PUBLIC)) {
visibility = ObjectModelJavaModifier.PUBLIC.toString();
} else if (modifiers.contains(ObjectModelJavaModifier.PRIVATE)) {
visibility = ObjectModelJavaModifier.PRIVATE.toString();
}
if (modifiers.contains(ObjectModelJavaModifier.PACKAGE)) {
visibility = ObjectModelJavaModifier.PACKAGE.toString();
}
return visibility;
}
public void setAssociationClassName(String associationClassName) {
this.associationClassName = associationClassName;
}
/**
* Returns whether this attribute reference a model classifier or not.
*
* @return a boolean indicating whether this attribute reference a model
* classifier or not.
*/
@Override
public boolean referenceClassifier() {
return getClassifier() != null;
}
/**
* Returns the classifier referenced by this attribute or null if it does
* not reference a model classifier.
*
* @return the ObjectModelClassfifier referenced by this attribute or null
* if it does not reference a model classifier.
*/
@Override
public ObjectModelClassifier getClassifier() {
return getModel().getClassifier(type);
}
/**
* Returns the attribute used to reference this class at the other end of
* the association or null if this is not an association, or if it is not
* bi-directionnal. @ see #getClassifier()
*
* @return the ObjectModelAttribute used to reference this class at the
* other end of the association or null if this is not an
* association, or if it is not bi-directionnal.
*/
@Override
public ObjectModelAttribute getReverseAttribute() {
ObjectModelAttribute reverseAttribute = null;
ObjectModelClassifier classifier = getClassifier();
if (classifier instanceof ObjectModelClass) {
reverseAttribute = classifier.getAttribute(getReverseAttributeName());
}
return reverseAttribute;
}
@Override
public String getReverseAttributeName() {
if (StringUtils.isEmpty(reverseAttributeName)) {
reverseAttributeName = GeneratorUtil
.toLowerCaseFirstLetter(getDeclaringElement().getName());
}
return reverseAttributeName;
}
@Override
public String getName() {
if (StringUtils.isEmpty(name) && getClassifier() != null) {
name = GeneratorUtil.toLowerCaseFirstLetter(getClassifier()
.getName());
}
return name;
}
@Override
public int getReverseMaxMultiplicity() {
return reverseMaxMultiplicity;
}
/**
* Returns the association class associated with this association, or null
* if there is none.
*
* @return a ObjectModelClass corresponding to the association class
* associated with this association, or null if there is none.
*/
@Override
public ObjectModelClass getAssociationClass() {
return getModel().getClass(associationClassName);
}
/**
* Returns whether this association has an associated association class, ot
* not.
*
* @return a boolean indicating whether this association has an associated
* association class, ot not.
*/
@Override
public boolean hasAssociationClass() {
return getAssociationClass() != null;
}
/**
* Returns whether this attribute is final or not.
*
* @return a boolean indicating whether this attribute is final or not.
*/
@Override
public boolean isFinal() {
return modifiers.contains(ObjectModelJavaModifier.FINAL);
}
public boolean isTransient() {
return modifiers.contains(ObjectModelJavaModifier.TRANSIENT);
}
@Override
public boolean isNavigable() {
return modifiers.contains(ObjectModelUMLModifier.NAVIGABLE);
}
@Override
public ObjectModelImplRef addStereotype(ObjectModelImplRef stereotype) {
String stereotypeName = stereotype.getName();
if (EugeneStereoTypes.STEREOTYPE_ORDERED.equals(stereotypeName)) {
setOrdered(true);
}
return super.addStereotype(stereotype);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy