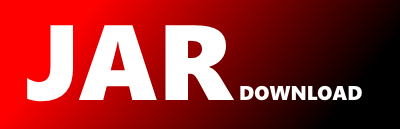
org.nuiton.eugene.plugin.AvailableDataMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-eugene-plugin Show documentation
Show all versions of maven-eugene-plugin Show documentation
maven plugin to use the eugene library
/*
* *##%
* EUGene :: Maven plugin
* Copyright (C) 2006 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package org.nuiton.eugene.plugin;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.nuiton.eugene.ModelReader;
import org.nuiton.eugene.Template;
import org.nuiton.eugene.models.Model;
import org.nuiton.eugene.models.object.ObjectModel;
import org.nuiton.eugene.writer.ChainedFileWriter;
import java.util.Arrays;
import java.util.EnumSet;
import java.util.Map;
import java.util.Set;
/**
* Obtain the list of some known data informations.
*
* Use the {@code dataTypes} property to specify a specific data type to use (otherwise
* will display all known data types).
*
* User: chemit
* Date: 24 nov. 2009
* Time: 00:22:37
*
* @goal available-data
* @requiresProject true
* @requiresDirectInvocation true
* @requiresDependencyResolution test
* @since 2.0.0
*/
public class AvailableDataMojo extends AbstractMojo {
/**
* Data type to display (let empty to see all datas).
* Can specify more than one separated by comma.
*
* Available types are :
*
* modeltype,
* modelreader,
* modeltemplate,
* writer
*
*
* Note: Let empty to display all data types.
*
* @parameter expression="${dataTypes}" default-value=""
* @since 2.0.0
*/
protected String dataTypes;
/**
* All available models (obtain by plexus, keys are plexus roles, values are a
* instance of corresponding model).
*
* @component role="org.nuiton.eugene.models.Model"
*/
protected Map modelTypes;
/**
* All available writers introspects via plexus
*
* @component role="org.nuiton.eugene.ModelReader"
*/
protected Map> modelReaders;
/**
* All available templates introspects via plexus
*
* @component role="org.nuiton.eugene.Template"
*/
protected Map> modelTemplates;
/**
* All available writers introspects via plexus
*
* @component role="org.nuiton.eugene.writer.ChainedFileWriter"
*/
protected Map writers;
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
StringBuilder buffer = new StringBuilder();
dataTypes = dataTypes == null ? "" : dataTypes.trim();
Set safeDataTypes;
if (StringUtils.isEmpty(dataTypes)) {
// treate all data types
safeDataTypes = EnumSet.allOf(AvailableData.class);
if (getLog().isDebugEnabled()) {
getLog().debug("will use all data types : " + safeDataTypes);
}
} else {
safeDataTypes = EnumSet.noneOf(AvailableData.class);
for (String s : dataTypes.split(",")) {
s = s.trim().toLowerCase();
try {
AvailableData data = AvailableData.valueOf(s);
if (getLog().isDebugEnabled()) {
getLog().debug("will use data type " + data);
}
safeDataTypes.add(data);
} catch (IllegalArgumentException e) {
getLog().warn("does not know data type : " + s + " use one of " + Arrays.toString(AvailableData.values()));
}
}
}
for (AvailableData data : safeDataTypes) {
appendData(data, buffer);
}
getLog().info("Get datas for data types : " + safeDataTypes + buffer.toString());
}
protected void appendData(AvailableData data, StringBuilder buffer) {
Map map = data.getData(this);
int size = map.size();
String dataType = data.name();
if (size == 0) {
buffer.append("\nNo available ").append(dataType).append(".");
} else if (size == 1) {
buffer.append("\nFound one ").append(dataType).append(" : ");
} else {
buffer.append("\nFound ").append(size).append(" ").append(dataType).append("s : ");
}
for (Map.Entry e : map.entrySet()) {
String name = e.getKey();
Object value = e.getValue();
buffer.append("\n [").append(name).append("] with implementation '").append(data.toString(value)).append("'");
}
}
enum AvailableData {
modeltype {
@Override
public Map getData(AvailableDataMojo mojo) {
return mojo.modelTypes;
}
},
writer {
@Override
public Map getData(AvailableDataMojo mojo) {
return mojo.writers;
}
@Override
String toString(Object data) {
ChainedFileWriter w = (ChainedFileWriter) data;
StringBuilder b = new StringBuilder(super.toString(data));
b.append("\n").append(" inputProtocol : ").append(w.getInputProtocol());
b.append("\n").append(" outputProtocol : ").append(w.getOutputProtocol(ObjectModel.NAME));
b.append("\n").append(" defaultIncludes : ").append(w.getDefaultIncludes());
b.append("\n").append(" defaultInputDirectory : ").append(w.getDefaultInputDirectory());
b.append("\n").append(" defaultTestInputDirectory : ").append(w.getDefaultTestInputDirectory());
return b.toString();
}
},
modelreader {
@Override
public Map getData(AvailableDataMojo mojo) {
return mojo.modelReaders;
}
},
modeltemplate {
@Override
public Map getData(AvailableDataMojo mojo) {
return mojo.modelTemplates;
}
};
abstract Map getData(AvailableDataMojo mojo);
String toString(Object data) {
return data.getClass().getName();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy