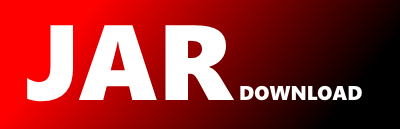
org.nuiton.eugene.plugin.CopyVersionFiles Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-eugene-plugin Show documentation
Show all versions of maven-eugene-plugin Show documentation
maven plugin to use the eugene library
/* *##%
* EUGene :: Maven plugin
* Copyright (C) 2006 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*/
package org.nuiton.eugene.plugin;
import org.nuiton.plugin.PluginIOContext;
import java.io.File;
import java.io.FileFilter;
import java.io.IOException;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.dom4j.DocumentException;
import org.dom4j.Node;
import org.dom4j.io.SAXReader;
import org.dom4j.Document;
import org.nuiton.plugin.PluginHelper;
/**
* Copy a file set to a versionned directory structure.
*
* @author chatellier
*
* @version $Revision: 755 $
*
* Last update : $Date: 2009-12-15 00:50:27 +0100 (mar., 15 déc. 2009) $ By : $Author: tchemit $
*
* @goal copyVersionFiles
*/
public class CopyVersionFiles extends EugeneAbstractMojo implements FileFilter {
/**
* Les entrées sorties du plugin.
*
*
*
* En entrée on demande des répertoires où chercher les fichiers objectmodel a convertir.
*
* En sortie on demande le répertoire ou generer les classes java.
*
* Par défaut on a les valeurs suivantes :
*
*
* <copyVersionResources>
*
* <input>target/generated-sources/models</input>
*
* <output>target/generated-sources/java</output>
*
* </copyVersionResources>
*
*
*
* Note: si {@link #testPhase} est activée, les valeurs par défaut sont :
*
*
* <copyVersionResources>
*
* <input>target/generated-sources/test-models</input>
*
* <output>target/generated-sources/test-java</output>
*
* </copyVersionResources>
*
*
* @parameter
* @since 1.0.0-rc-8
*/
protected PluginIOContext copyVersionResources;
/**
* Fichiers objectModel a lire pour determiner la version.
*
* @parameter expression="${generator.includes}" default-value="*.*model"
* @since 0.51
*/
protected String includes;
/**
* Le dossier de destination des fichiers copiés.
*
* Doit contenir un nom de modele : %MODELNAME%
*
* @parameter expression="${generator.copyVersionDir}"
* @since 0.51
* @required
*/
protected String copyVersionDir;
/**
* Les mappings a sauvegarder
*
* @parameter expression="${generator.copyVersionFiles}"
* @since 0.51
* @required
*/
protected String copyVersionFiles;
/**
* Version trouvee dans les fichiers objectModel.
*
* Type string, parce que elle peut avoir la forme "1.3.2" par exemple
*/
protected String versionFound = null;
/**
* Nom du model sauvegarde
*/
protected String modelNameFound = null;
/**
* Dossier incluant le nom de la version
*/
protected File fVersionDir;
@Override
public void doAction() throws MojoExecutionException, MojoFailureException {
// find version and model name
findVersionAndModelName();
if (versionFound == null || !versionFound.matches("[0-9]+(\\.[0-9]+)*")) {
versionFound = "0";
getLog().info("No version found in model files, setting version to '" + versionFound + "'");
} else {
getLog().info("Version '" + versionFound + "' found in model description");
}
String destDir = copyVersionDir.replace("%MODELNAME%", modelNameFound) + File.separator + versionFound;
fVersionDir = new File(destDir);
if (overwrite || !checkExistence()) {
try {
PluginHelper.copyFiles(copyVersionResources.getOutput(), fVersionDir, new String[]{copyVersionFiles}, null, true);
} catch (IOException ex) {
throw new MojoExecutionException("could not copy some files for reason " + ex.getMessage(), ex);
}
}
}
@Override
protected PluginIOContext getResources() {
return copyVersionResources;
}
@Override
protected PluginIOContext initResources() {
File defaultIn = getFileFromBasedir("target", "generated-sources", "models");
File defaultOut = getFileFromBasedir("target", "generated-sources", "java");
File defaultTestIn = getFileFromBasedir("target", "generated-sources", "test-models");
File defaultTestOut = getFileFromBasedir("target", "generated-sources", "test-java");
copyVersionResources = initResources(defaultIn, defaultOut, defaultTestIn, defaultTestOut);
return copyVersionResources;
}
/**
* Check if previous saved files are already present
* @return true
if already present,false
otherwise.
*/
protected boolean checkExistence() {
boolean exist = false;
if (fVersionDir.exists() && fVersionDir.listFiles().length > 0) {
getLog().warn("[COPY] Warning saved files for version '" + versionFound + "' and name '" + modelNameFound + "' already exists");
getLog().warn("[COPY] Copy won't be done unless copyOverwrite " + "parameter is set to 'true' or version is updated");
exist = true;
}
return exist;
}
// /**
// * Copy hibernate files.
// *
// * Using ant task
// * @throws IOException
// */
// protected void copyAction() throws IOException {
// creation du repertoire
// fVersionDir.mkdirs();
// destDirGen.mkdirs();
//
// /* Création d'un projet ant */
// Project p = createProject();
//
// /* Création de la tâche ant Copy */
// Copy copy = createCopyTask(p);
//
// /* Configuration */
// copy.setTodir(fVersionDir);
// copy.setOverwrite(true);
//
// FileSet fileSet = new FileSet();
//// fileSet.setDir(destDirGen);
// fileSet.setDir(copyVersionResources.getOutput());
// fileSet.setIncludes(copyVersionFiles);
// copy.addFileset(fileSet);
//
// /* Execution */
// copy.execute();
// }
/**
* Find version and name in object model files
*/
protected void findVersionAndModelName() {
//TODO TC-20090820 : iterate on all inputs
File srcModelDir = copyVersionResources.getInputs()[0];
File[] modelFiles = srcModelDir.listFiles(this);
//FIXME TC-20090820 this is a bit funny iterate and keep the last model values ?
// should iterate and do the treatment for each model
for (File modelFile : modelFiles) {
SAXReader saxR = new SAXReader();
Document document;
try {
document = saxR.read(modelFile);
Node node;
node = document.selectSingleNode("/objectModel/@version");
if (node != null) {
versionFound = node.getStringValue();
}
node = document.selectSingleNode("/objectModel/@name");
if (node != null) {
modelNameFound = node.getStringValue();
}
} catch (DocumentException e) {
getLog().error("Can't read document", e);
}
}
}
@Override
public boolean accept(File arg0) {
String fullPath = arg0.getAbsolutePath();
// regex
String regexInclude = includes.replaceAll("\\.", "\\.").replaceAll(
"([^\\*])\\*([^\\*])", "$1[^/]*$2").replaceAll("\\*\\*", ".*") + "$";
return fullPath.matches(regexInclude);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy