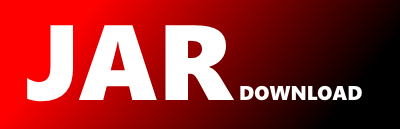
org.nuiton.eugene.plugin.writer.ModelChainedFileWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-eugene-plugin Show documentation
Show all versions of maven-eugene-plugin Show documentation
maven plugin to use the eugene library
/*
* *##%
* EUGene :: Maven plugin
* Copyright (C) 2006 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package org.nuiton.eugene.plugin.writer;
import org.apache.commons.lang.StringUtils;
import org.nuiton.eugene.ModelReader;
import org.nuiton.eugene.Template;
import org.nuiton.eugene.models.Model;
import org.nuiton.eugene.writer.ChainedFileWriterConfiguration;
import org.nuiton.plugin.PluginHelper;
import org.nuiton.plugin.PluginIOContext;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Properties;
/**
* To write model files from zargo files.
*
* @author tchemit
* @plexus.component role="org.nuiton.eugene.writer.ChainedFileWriter" role-hint="model2Java"
* @since 2.0.0
*/
public class ModelChainedFileWriter extends BaseChainedFileWriter {
public static final String PROP_GENERATED_PACKAGES = "generatedPackages";
public static final String PROP_EXCLUDE_TEMPLATES = "excludetemplates";
public static final String PROP_TEMPLATES = "templates";
public static final String PROP_TEMPLATES_LIST = "templatesList";
public static final String PROP_DEFAULT_PACKAGE = "defaultPackage";
public static final String PROP_MODEL_READER = "modelReader";
public static final String PROP_READER = "reader";
public ModelChainedFileWriter() {
super(
PROP_TEMPLATES, "templates",
PROP_TEMPLATES_LIST, "templatesList",
PROP_EXCLUDE_TEMPLATES, "excludetemplates",
PROP_READER, "reader",
PROP_MODEL_READER, "modelReader",
PROP_GENERATED_PACKAGES, "generatedPackages",
PROP_DEFAULT_PACKAGE, "defaultPackage"
);
}
@Override
public String getInputProtocol() {
return "model";
}
@Override
public String getOutputProtocol(String modelType) {
// nothing after java files
return null;
}
@Override
public boolean acceptModel(String modelType) {
// accept all models
return acceptObjectModelOrStateModel(modelType);
}
@Override
public boolean acceptInclude(String include) {
return include.startsWith("model:") || include.endsWith(".objectmodel") || include.endsWith(".statemodel");
}
@Override
public String getDefaultIncludes() {
return "**/*.*model";
}
@Override
public String getDefaultInputDirectory() {
return "src/main/models";
}
@Override
public String getDefaultOutputDirectory() {
return "java";
}
@Override
public String getDefaultTestInputDirectory() {
return "src/test/models";
}
@Override
public String getDefaultTestOutputDirectory() {
return "test-java";
}
public String getDefaultPackage() {
return getProperty(PROP_DEFAULT_PACKAGE, String.class);
}
public String[] getExcludeTemplates() {
return getProperty(PROP_EXCLUDE_TEMPLATES, String[].class);
}
public String getGeneratedPackages() {
return getProperty(PROP_GENERATED_PACKAGES, String.class);
}
public List> getTemplatesList() {
return getProperty(PROP_TEMPLATES_LIST, List.class);
}
public String getTemplates() {
return getProperty(PROP_TEMPLATES, String.class);
}
protected ModelReader> getModelReader() {
return getProperty(PROP_MODEL_READER, ModelReader.class);
}
protected String getReader() {
return getProperty(PROP_READER, String.class);
}
@Override
protected void initWriter(ChainedFileWriterConfiguration configuration) {
super.initWriter(configuration);
// obtain a reader
ClassLoader loader = configuration.getClassLoader();
if (getModelReader() == null) {
if (getReader() != null) {
// use a specific reader
String reader = getReader();
try {
ClassLoader fixedClassLoader = loader;
ModelReader> modelReader = (ModelReader>) Class.forName(reader, true, fixedClassLoader).newInstance();
//TODO : should check that the reader is compatible with given modelType
properties.put(PROP_MODEL_READER, modelReader);
} catch (Exception eee) {
throw new IllegalStateException("could not obtain reader " + reader, eee);
}
} else {
String modelType = configuration.getModelType();
ModelReader> modelReader = configuration.getModelReaders().get(modelType);
if (modelReader == null) {
throw new IllegalStateException("could not find a model reader for modelType : " + modelType + ", availables readers : " + configuration.getModelReaders().values());
}
properties.put(PROP_MODEL_READER, modelReader);
}
}
Properties templateProperties = new Properties();
templateProperties.setProperty(Template.PROP_DEFAULT_PACKAGE, getDefaultPackage());
templateProperties.setProperty(Template.PROP_OVERWRITE, String.valueOf(configuration.isOverwrite()));
templateProperties.setProperty(Template.PROP_ENCODING, configuration.getEncoding());
templateProperties.setProperty(Template.PROP_LAST_MODIFIED_SOURCE, String.valueOf(getModelReader().getLastModifiedSource()));
String generatedPackages = getGeneratedPackages();
if (StringUtils.isEmpty(generatedPackages)) {
getLog().info(" generating all packages");
} else {
templateProperties.put(Template.PROP_GENERATED_PACKAGES, generatedPackages);
getLog().info(" generating only for packages " + generatedPackages);
}
// init templates
List> templatesList = new ArrayList>();
String[] templatesNames = getTemplates().split(",");
for (String templateName : templatesNames) {
// remove trailing spaces
templateName = templateName.trim();
Template template;
template = (Template) configuration.getModelTemplates().get(templateName);
if (template == null) {
getLog().warn("template [" + templateName + "] is not registred via plexus, try to load it directly");
try {
template = (Template) Class.forName(templateName, true, loader).newInstance();
} catch (Exception e) {
throw new IllegalStateException("Can't obtain template [" + templateName + "] for reason " + e.getMessage(), e);
}
} else {
getLog().info("will use the template [" + templateName + "]");
}
// will use this template
templatesList.add(template);
// set the properties of the template
template.setProperties(templateProperties);
}
properties.put(PROP_TEMPLATES_LIST, templatesList);
}
@Override
public void generate(ChainedFileWriterConfiguration configuration, File outputDir, File inputDirectory, String includePattern) throws IOException {
PluginIOContext ioContext = new PluginIOContext();
ioContext.setInput(inputDirectory);
ioContext.setOutput(outputDir);
// obtain files
List modelFiles = new ArrayList();
String[] includePatterns = includePattern.split(",");
getLog().info("Generating from " + inputDirectory + " : " + includePattern);
for (File srcDirGen : ioContext.getInputs()) {
if (configuration.isVerbose()) {
getLog().info("Search for " + Arrays.toString(includePatterns) + " in " + srcDirGen.getAbsolutePath());
}
List currentFiles = PluginHelper.getIncludedFiles(srcDirGen, includePatterns, null);
modelFiles.addAll(currentFiles);
}
// read the model
Model model = getModelReader().read(modelFiles.toArray(new File[modelFiles.size()]));
// apply all templates to the model
for (Template template : getTemplatesList()) {
getLog().info("Apply " + template.getClass().getSimpleName() + " generator");
// apply template
template.applyTemplate(model, ioContext.getOutput());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy