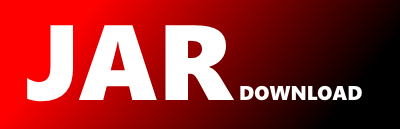
org.nuiton.i18n.I18nFileReader Maven / Gradle / Ivy
/* *##% Lutin utilities library
* Copyright (C) 2004 - 2008 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* . ##%* */
/* *
* I18nFileReader.java
*
* Created: Nov 22, 2004
*
* @author Cédric Pineau
* @version $Revision: 1550 $
*
* Last update : $Date: 2009-05-14 07:41:01 +0200 (jeu., 14 mai 2009) $
* by : $Author: tchemit $
*/
package org.nuiton.i18n;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
import java.util.Properties;
import java.util.regex.Pattern;
/** Classe assurant la lecture et les possibles traitement nécessaires à I18n. */
public class I18nFileReader extends Properties {
protected static final Pattern commentPattern = Pattern.compile("[^\\\\]#");
protected static final Pattern splitPattern = Pattern.compile("[^\\\\]=");
private static final long serialVersionUID = 3611718334066783394L;
public void load(InputStream inStream, String encodingTo) throws IOException {
Charset charsetTo = Charset.forName(encodingTo);
BufferedReader readerFile;
readerFile = new BufferedReader(new InputStreamReader(inStream, charsetTo));
String lineFile;
StringBuilder builderFile;
builderFile = new StringBuilder();
while ((lineFile = readerFile.readLine()) != null) {
builderFile.append(lineFile).append('\n');
}
readerFile.close();
super.load(new ByteArrayInputStream(builderFile.toString().getBytes()));
}
protected String interpretBackslashes(String message) {
int backslashIndex = -1;
while ((backslashIndex = message.indexOf("\\", backslashIndex + 1)) != -1) {
if (message.length() >= backslashIndex + 1) {
char charNextToBackslash = message.charAt(backslashIndex + 1);
char replacementChar;
switch (charNextToBackslash) {
case '\\':
replacementChar = '\\';
break;
case 't':
replacementChar = '\t';
break;
case 'n':
replacementChar = '\n';
break;
case ' ':
replacementChar = ' ';
break;
case '=':
replacementChar = '=';
break;
case ':':
replacementChar = ':';
break;
default:
replacementChar = '\\';
break;
}
message = message.substring(0, backslashIndex) + replacementChar + message.substring(backslashIndex + 2);
}
}
return message;
}
private static char[] chars = {'\\', '\n', '\t', ' ', '=', ':'};
protected String serializeBackslashes(String message) {
for (char c : chars) {
int charIndex = -1;
while ((charIndex = message.indexOf(c, charIndex + 2)) != -1) {
String replacementString = "" + c;
switch (c) {
case '\\':
replacementString = "\\\\";
break;
case '\t':
replacementString = "\\t";
break;
case '\n':
replacementString = "\\n";
break;
case ' ':
replacementString = "\\ ";
break;
case '=':
replacementString = "\\=";
break;
case ':':
replacementString = "\\:";
break;
}
message = message.substring(0, charIndex) + replacementString + message.substring(charIndex + 1);
}
}
return message;
}
} //I18nFileReader
© 2015 - 2025 Weber Informatics LLC | Privacy Policy