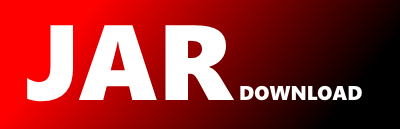
org.nuiton.i18n.bundle.I18nBundleScope Maven / Gradle / Ivy
/*
* *##% Lutin utilities library
* Copyright (C) 2004 - 2008 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* . ##%* */
package org.nuiton.i18n.bundle;
import java.util.Locale;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.nuiton.i18n.I18nUtil;
/**
* The enumaration defines the scope of a bundle entry.
*
* There is three scope possible:
*
* - {@link #GENERAL} : for a bundle entry with no locale specialized information, eg :
bundle.properties
* - {@link #LANGUAGE} : for a bundle entry with language locale specialized information, eg :
bundle-en.properties
* - {@link #FULL} : for a bundle entry with full locale specialized information, eg :
bundle-en_GB.properties
*
*
* We define a order relation, from general to full scope :
*
* {@link #GENERAL} < {@link #LANGUAGE} < {@link #FULL}
*
* Scopes are inclusives, in a search of entries, eg the search of en_GB
will include en
scope...
*
* The {@link #patternAll} is the searching pattern of bundle of the scope.
*
* The method {@link #getMatcher(String)} obtain from the {@link #patternAll} the matcher for a bundle path.
*
* The method {@link #getLocale(Matcher)} obtain from the {@link #patternAll} matched in a bundle path, the
* corresponding locale.
*
* The class offer also a static method {@link #valueOf(java.util.Locale)} to obtain the scope of a locale.
*
* @author chemit
*/
public enum I18nBundleScope {
/** default scope (with no language, nor country information) */
// GENERAL("(.*18n/.+)\\.properties") {
GENERAL("(.*/.+)\\.properties") {
@Override
public Locale getLocale(Matcher matcher) {
// no locale for general bundle
return null;
}
},
/** language scope (no country information) */
// LANGUAGE("(.*18n/.+)-(\\w\\w)\\.properties") {
LANGUAGE("(.*/.+)-(\\w\\w)\\.properties") {
@Override
public Locale getLocale(Matcher matcher) {
Locale result = null;
if (matcher.matches()) {
result = I18nUtil.newLocale(matcher.group(2));
}
return result;
}
},
/** full scope : language + country */
// FULL("(.*18n/.+)-(\\w\\w_\\w\\w)\\.properties") {
FULL("(.*/.+)-(\\w\\w_\\w\\w)\\.properties") {
@Override
public Locale getLocale(Matcher matcher) {
Locale result = null;
if (matcher.matches()) {
result = I18nUtil.newLocale(matcher.group(2));
}
return result;
}
};
/** pattern used to detect bundle entry */
private final Pattern patternAll;
/**
* Obtain the scope of a given locale
.
*
* The given locale can be null, which means {@link I18nBundleScope#GENERAL} scope.
*
* @param locale given locale to convert
* @return the scope of given locale
*/
public static I18nBundleScope valueOf(Locale locale) {
if (locale == null || locale.getLanguage() == null || locale.getLanguage().length() == 0) {
return GENERAL;
}
if (locale.getCountry() == null || locale.getCountry().length() == 0) {
return LANGUAGE;
}
return FULL;
}
/**
* get a matcher fro the given path for this scope
*
* @param path the path to treate
* @return the bunle detect matcher
*/
public Matcher getMatcher(String path) {
return patternAll.matcher(path);
}
/**
* get the locale for a given matcher.
*
* @param matcher the scope matcher to use
* @return the locale
*/
public abstract Locale getLocale(Matcher matcher);
/**
* @param matcher the scope matcher to use
* @return the prefix of the bundle
*/
public String getBundlePrefix(Matcher matcher) {
String result = null;
if (matcher.matches()) {
result = matcher.group(1);
}
return result;
}
private I18nBundleScope(String patternAll) {
this.patternAll = Pattern.compile(patternAll);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy