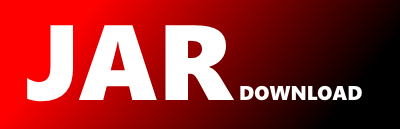
org.nuiton.util.LocaleConverter Maven / Gradle / Ivy
/*
* *##% Lutin utilities library
* Copyright (C) 2004 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* . ##%* */
package org.nuiton.util;
import org.apache.commons.beanutils.ConversionException;
import org.apache.commons.beanutils.Converter;
import static org.apache.commons.logging.LogFactory.getLog;
import org.nuiton.i18n.CountryEnum;
import org.nuiton.i18n.LanguageEnum;
import java.util.Locale;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* classe pour convertir une chaine en un objet {@link java.util.Locale}.
*
* @author chemit
*/
public class LocaleConverter implements Converter {
private static final Pattern FULL_SCOPE_PATTERN = Pattern.compile("([a-zA-Z]{2})_([a-zA-Z]{2})");
private static final Pattern MEDIUM_SCOPE_PATTERN = Pattern.compile("([a-zA-Z]{2})");
/** to use log facility, just put in your code: log.info(\"...\"); */
static org.apache.commons.logging.Log log = getLog(LocaleConverter.class);
public Object convert(Class aClass, Object value) {
if (value == null) {
throw new ConversionException("can not convert null value in " + this + " convertor");
}
if (isEnabled(aClass)) {
Object result;
if (isEnabled(value.getClass())) {
result = value;
return result;
}
if (value instanceof String) {
result = valueOf(((String) value).trim());
return result;
}
}
throw new ConversionException("could not find a convertor for type " + aClass.getName() + " and value : " + value);
}
protected Locale valueOf(String value) {
try {
Locale result = convertFullScope(value);
if (result == null) {
result = convertMediumScope(value);
}
if (result == null) {
throw new ConversionException("could not convert locale " + value);
}
return result;
} catch (Exception e) {
throw new ConversionException("could not convert locale " + value + " for reason " + e.getMessage());
}
}
private Locale convertFullScope(String value) {
Matcher m = FULL_SCOPE_PATTERN.matcher(value);
if (m.matches()) {
// found a full scope pattern (language + country)
LanguageEnum language = LanguageEnum.valueOf(m.group(1).toLowerCase());
CountryEnum country = CountryEnum.valueOf(m.group(2).toUpperCase());
if (language == null || country == null) {
// not safe
throw new ConversionException("could not convert locale " + value);
}
return new Locale(language.name(), country.name());
}
return null;
}
private Locale convertMediumScope(String value) {
Matcher m = MEDIUM_SCOPE_PATTERN.matcher(value);
if (m.matches()) {
// found a medium scope pattern (only language)
LanguageEnum language = LanguageEnum.valueOf(m.group(1).toLowerCase());
if (language == null) {
// not safe
throw new ConversionException("could not convert locale " + value);
}
return new Locale(language.name());
}
return null;
}
public LocaleConverter() {
if (log.isDebugEnabled()) {
log.debug(this);
}
}
protected boolean isEnabled(Class aClass) {
return aClass == Locale.class;
}
public Class> getType() {
return Locale.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy