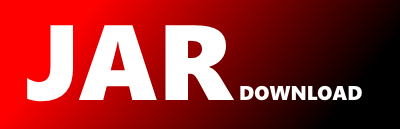
org.nuiton.jaxx.application.bean.JavaBeanObjectUtil Maven / Gradle / Ivy
package org.nuiton.jaxx.application.bean;
/*
* #%L
* JAXX :: Application API
* %%
* Copyright (C) 2008 - 2014 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.base.Preconditions;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import org.apache.commons.beanutils.PropertyUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.jaxx.application.ApplicationTechnicalException;
import java.beans.PropertyChangeListener;
import java.beans.PropertyChangeListenerProxy;
/**
* Created on 8/14/14.
*
* @author Tony Chemit - [email protected]
* @since 2.10
*/
public class JavaBeanObjectUtil {
/** Logger. */
private static final Log log = LogFactory.getLog(JavaBeanObjectUtil.class);
public static void removeAllPropertyChangeListeners(Iterable extends JavaBeanObject> beans) {
for (JavaBeanObject bean : beans) {
removeAllPropertyChangeListeners(bean);
}
}
public static void removeAllPropertyChangeListeners(JavaBeanObject bean) {
removePropertyChangeListeners(bean, Predicates.alwaysTrue());
}
public static void removeAllRemovablePropertyChangeListeners(JavaBeanObject bean) {
removePropertyChangeListeners(bean, new Predicate() {
@Override
public boolean apply(PropertyChangeListener input) {
return input instanceof RemoveablePropertyChangeListener;
}
});
}
public static void removePropertyChangeListeners(JavaBeanObject bean, Predicate predicate) {
PropertyChangeListener[] propertyChangeListeners = bean.getPropertyChangeListeners();
for (PropertyChangeListener listener : propertyChangeListeners) {
if (listener instanceof PropertyChangeListenerProxy) {
PropertyChangeListenerProxy listenerProxy = (PropertyChangeListenerProxy) listener;
listener = listenerProxy.getListener();
}
if (predicate.apply(listener)) {
if (log.isDebugEnabled()) {
log.debug("Remove listener: " + listener);
}
bean.removePropertyChangeListener(listener);
}
}
}
public static void setProperty(Object bean, String property, Object value) {
Preconditions.checkNotNull(bean);
Preconditions.checkNotNull(property);
try {
PropertyUtils.setSimpleProperty(bean, property, value);
} catch (Exception e) {
throw new ApplicationTechnicalException(String.format("Could not set property %1s not found on object of type %2s", property, bean.getClass().getName()), e);
}
}
public static Object getProperty(Object bean, String property) {
Preconditions.checkNotNull(bean);
Preconditions.checkNotNull(property);
try {
return PropertyUtils.getSimpleProperty(bean, property);
} catch (Exception e) {
throw new ApplicationTechnicalException(String.format("Could not get property %1s on object of type %2s", property, bean.getClass().getName()), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy