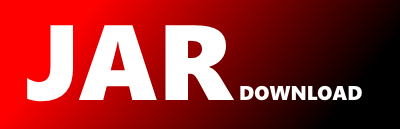
org.nuiton.jaxx.application.swing.action.ApplicationActionUIHandler Maven / Gradle / Ivy
package org.nuiton.jaxx.application.swing.action;
/*
* #%L
* JAXX :: Application Swing
* %%
* Copyright (C) 2008 - 2014 Code Lutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import jaxx.runtime.SwingUtil;
import jaxx.runtime.validator.swing.SwingValidator;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.decorator.Decorator;
import org.nuiton.jaxx.application.swing.AbstractApplicationUIHandler;
import org.nuiton.jaxx.application.type.ApplicationProgressionModel;
import javax.swing.JComponent;
import java.awt.Component;
import java.awt.Cursor;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import java.awt.event.ComponentListener;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import static org.nuiton.i18n.I18n.t;
/**
* @author Tony Chemit - [email protected]
* @since 2.8
*/
public class ApplicationActionUIHandler extends AbstractApplicationUIHandler {
/** Logger. */
private static final Log log =
LogFactory.getLog(ApplicationActionUIHandler.class);
protected PropertyChangeListener progressionListener = new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
String propertyName = evt.getPropertyName();
if (ApplicationProgressionModel.PROPERTY_MESSAGE.equals(propertyName)) {
// change message
String newMessage = (String) evt.getNewValue();
ui.getTaskActionLabel().setText("" + newMessage + "");
ui.pack();
} else if (ApplicationProgressionModel.PROPERTY_TOTAL.equals(propertyName)) {
// change total progressbar max
ui.getTaskProgressBar().setMaximum((Integer) evt.getNewValue());
} else if (ApplicationProgressionModel.PROPERTY_CURRENT.equals(propertyName)) {
// change value of progress bar
ui.getTaskProgressBar().setValue((Integer) evt.getNewValue());
}
}
};
protected ComponentListener listener = new ComponentAdapter() {
boolean moving;
boolean resizing;
@Override
public void componentMoved(ComponentEvent e) {
Component mainUI = (Component) e.getSource();
if (!moving && mainUI.isShowing()) {
moving = true;
try {
setLocation(mainUI);
} finally {
moving = false;
}
}
}
@Override
public void componentResized(ComponentEvent e) {
Component mainUI = (Component) e.getSource();
if (!resizing && mainUI.isShowing()) {
resizing = true;
try {
setSize(mainUI);
} finally {
resizing = false;
}
}
}
};
//------------------------------------------------------------------------//
//-- AbstractTuttiUIHandler methods --//
//------------------------------------------------------------------------//
@Override
public void beforeInit(ApplicationActionUI ui) {
this.ui = ui;
ApplicationActionUIModel model = new ApplicationActionUIModel();
ui.setContextValue(model);
model.addPropertyChangeListener(ApplicationActionUIModel.PROPERTY_ACTION, new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
// udpate global label
AbstractApplicationAction action = (AbstractApplicationAction) evt.getNewValue();
if (log.isDebugEnabled()) {
log.debug("Action to use: " + action);
}
if (action == null) {
// stoping action
hideAction();
} else {
// starting action
showAction(action);
}
}
});
model.addPropertyChangeListener(ApplicationActionUIModel.PROPERTY_PROGRESSION_MODEL, new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
// change progression model
ApplicationProgressionModel oldValue = (ApplicationProgressionModel) evt.getOldValue();
ApplicationProgressionModel newValue = (ApplicationProgressionModel) evt.getNewValue();
if (log.isDebugEnabled()) {
log.debug("progression model: " + newValue);
}
if (oldValue != null) {
oldValue.removePropertyChangeListener(progressionListener);
}
if (newValue == null) {
// remove progression model
ApplicationActionUIHandler.this.ui.getTaskPanel().setVisible(false);
} else {
// use progression model
ApplicationActionUIHandler.this.ui.getTaskPanel().setVisible(true);
newValue.addPropertyChangeListener(progressionListener);
}
}
});
}
@Override
public void afterInit(ApplicationActionUI ui) {
initUI(this.ui);
// installation layer de blocage en mode busy
SwingUtil.setLayerUI(this.ui.getRootPanel(), this.ui.getBusyBlockLayerUI());
this.ui.setCursor(Cursor.getPredefinedCursor(Cursor.WAIT_CURSOR));
}
@Override
protected JComponent getComponentToFocus() {
return null;
}
@Override
public void onCloseUI() {
}
@Override
public SwingValidator getValidator() {
return null;
}
@Override
public Component getTopestUI() {
return null;
}
@Override
public Decorator getDecorator(Class beanType, String decoratorContext) {
return null;
}
//------------------------------------------------------------------------//
//-- Internal methods --//
//------------------------------------------------------------------------//
protected void hideAction() {
ui.setVisible(false);
}
protected void showAction(AbstractApplicationAction action) {
ui.setTitle(t("jaxx.application.title.actionUI",
getContext().getConfiguration().getApplicationName(),
getContext().getConfiguration().getVersion(),
action.getActionDescription()));
ui.getGlobalActionLabel().setText(
t("jaxx.application.message.action.running",
action.getActionDescription()));
ui.pack();
Component mainUI = getContext().getMainUI();
if (mainUI != null) {
mainUI.addComponentListener(listener);
setLocation(mainUI);
setSize(mainUI);
}
try {
ui.setVisible(true);
} finally {
if (mainUI != null) {
mainUI.removeComponentListener(listener);
}
}
}
protected void setLocation(Component mainUI) {
Component component = getContext().getBodyUI();
Component status = getContext().getStatusUI();
int width = component == null ? 0 : component.getWidth();
int height = component == null ? 0 : component.getHeight() + status.getHeight();
int x;
int y;
if (height == 0) {
x = mainUI.getX() + 5;
y = mainUI.getY() + 15;
} else {
x = mainUI.getX() + (mainUI.getWidth() - width);
y = mainUI.getY() + (mainUI.getHeight() - height);
}
ui.setLocation(x, y);
}
protected void setSize(Component mainUI) {
// Better to let his own size to the action progress bar
// see http://forge.codelutin.com/issues/3263
// Container component = mainUI.getBody();
// int width = component == null ? 0 : component.getWidth();
ui.pack();
// if (width != 0 && ui.getWidth() < width) {
// ui.setSize(width, ui.getHeight());
// }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy