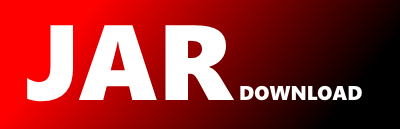
jaxx.compiler.java.JavaElementFactory Maven / Gradle / Ivy
/*
* #%L
* JAXX :: Compiler
* %%
* Copyright (C) 2008 - 2014 Code Lutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package jaxx.compiler.java;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.util.StringUtil;
/**
* Factory of any element in a {@link JavaFile}.
*
* Always pass by this factory to have common behaviour (imports,...)
*
* @author Tony Chemit - [email protected]
* @since 2.4
*/
public class JavaElementFactory {
/** Logger. */
static private final Log log = LogFactory.getLog(JavaElementFactory.class);
public static JavaFile newFile(int modifiers, String className) {
return new JavaFile(modifiers, className);
}
public static JavaArgument newArgument(String type,
String name) {
return newArgument(type, name, false);
}
public static JavaArgument newArgument(String type,
String name,
boolean isFinal) {
return new JavaArgument(type, name, isFinal);
}
public static JavaField newField(int modifiers,
String returnType,
String name,
boolean override) {
return newField(modifiers, returnType, name, override, null);
}
public static JavaField newField(int modifiers,
String returnType,
String name,
boolean override,
String initializer,
String... initializerTypes) {
return new JavaField(modifiers,
returnType,
name,
override,
initializer,
initializerTypes
);
}
public static JavaConstructor newConstructor(int modifiers,
String name,
String body,
String[] exceptions,
JavaArgument... arguments) {
return new JavaConstructor(modifiers,
name,
arguments,
exceptions,
body
);
}
public static JavaConstructor newConstructor(int modifiers,
String name,
String body,
JavaArgument... arguments) {
return newConstructor(modifiers,
name,
body,
StringUtil.EMPTY_STRING_ARRAY,
arguments
);
}
public static JavaMethod newMethod(int modifiers,
String returnType,
String name,
String body,
boolean override,
String[] exceptions,
JavaArgument... arguments) {
if (log.isDebugEnabled()) {
log.debug(name + " returns : " + returnType);
}
return new JavaMethod(modifiers,
returnType,
name,
arguments,
exceptions,
body,
override
);
}
public static JavaMethod newMethod(int modifiers,
String returnType,
String name,
String body,
boolean override,
JavaArgument... arguments) {
return newMethod(modifiers,
returnType,
name,
body,
override,
StringUtil.EMPTY_STRING_ARRAY,
arguments
);
}
public static JavaField cloneField(JavaField field) {
return newField(
field.getModifiers(),
field.getType(),
field.getName(),
field.isOverride(),
field.getInitializer(),
field.getInitializerTypes());
}
public static JavaMethod cloneMethod(JavaMethod method) {
String[] incomingExceptions = method.getExceptions();
String[] exceptions = new String[incomingExceptions.length];
System.arraycopy(incomingExceptions, 0, exceptions, 0, exceptions.length);
JavaArgument[] oldArguments = method.getArguments();
int nbArguments = oldArguments.length;
JavaArgument[] arguments = new JavaArgument[nbArguments];
for (int i = 0; i < nbArguments; i++) {
JavaArgument argument = oldArguments[i];
arguments[i] = cloneArgument(argument);
}
return newMethod(
method.getModifiers(),
method.getReturnType(),
method.getName(),
method.getBody(),
method.isOverride(),
exceptions,
arguments);
}
public static JavaArgument cloneArgument(JavaArgument argument) {
return newArgument(
argument.getType(),
argument.getName(),
argument.isFinal()
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy