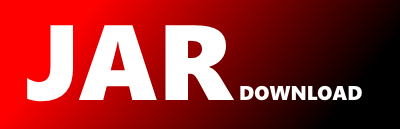
jaxx.runtime.swing.config.ConfigUIHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-config Show documentation
Show all versions of jaxx-config Show documentation
Config UI based on org.nuiton.config.ApplicationConfig
package jaxx.runtime.swing.config;
/*
* #%L
* JAXX :: Config
* %%
* Copyright (C) 2008 - 2014 Code Lutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.base.Preconditions;
import com.google.common.base.Supplier;
import jaxx.runtime.JAXXContext;
import jaxx.runtime.context.JAXXInitialContext;
import jaxx.runtime.swing.config.model.CallBackFinalizer;
import jaxx.runtime.swing.config.model.CategoryModel;
import jaxx.runtime.swing.config.model.ConfigUIModel;
import jaxx.runtime.swing.config.model.ConfigUIModelBuilder;
import jaxx.runtime.swing.config.model.OptionModel;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.config.ApplicationConfig;
import org.nuiton.config.ConfigOptionDef;
import javax.swing.Icon;
import javax.swing.table.TableCellEditor;
import java.awt.Frame;
import java.io.File;
import javax.swing.table.TableCellRenderer;
/**
* A helper to build a config ui.
*
* contains all states as method to build model, then ui and finally display it.
*
* @author Tony Chemit - [email protected]
* @since 2.5.11
*/
public class ConfigUIHelper {
public static final Log log = LogFactory.getLog(ConfigUIHelper.class);
/** internal model builder */
protected final ConfigUIModelBuilder modelBuilder;
/** internal model after builder flush */
protected ConfigUIModel model;
protected ConfigUI ui;
public ConfigUIHelper(Supplier config) {
modelBuilder = new ConfigUIModelBuilder();
modelBuilder.createModel(config);
}
public ConfigUIHelper(Supplier config, File configFile) {
modelBuilder = new ConfigUIModelBuilder();
modelBuilder.createModel(config, configFile);
}
public ConfigUIHelper(ApplicationConfig config, File configFile) {
modelBuilder = new ConfigUIModelBuilder();
modelBuilder.createModel(config, configFile);
}
public ConfigUIHelper(ApplicationConfig config) {
modelBuilder = new ConfigUIModelBuilder();
modelBuilder.createModel(config);
}
public ConfigUIModel getModel() {
if (model == null) {
model = modelBuilder.flushModel();
}
return model;
}
public ConfigUIModelBuilder addCategory(String categoryName, String categoryLabel)
throws IllegalStateException, NullPointerException {
modelBuilder.addCategory(categoryName, categoryLabel);
return modelBuilder;
}
public ConfigUIModelBuilder addCategory(String categoryName, String categoryLabel, String categoryCallback)
throws IllegalStateException, NullPointerException {
modelBuilder.addCategory(categoryName, categoryLabel, categoryCallback);
return modelBuilder;
}
public ConfigUIModelBuilder addOption(ConfigOptionDef def)
throws IllegalStateException, NullPointerException {
modelBuilder.addOption(def);
return modelBuilder;
}
@Deprecated
public ConfigUIModelBuilder addOption(ConfigOptionDef def, String propertyName)
throws IllegalStateException, NullPointerException {
modelBuilder.addOption(def, propertyName);
return modelBuilder;
}
public ConfigUIModelBuilder setOptionPropertyName(String propertyName)
throws IllegalStateException, NullPointerException {
modelBuilder.setOptionPropertyName(propertyName);
return modelBuilder;
}
public ConfigUIModelBuilder setOptionShortLabel(String shortLabel)
throws IllegalStateException, NullPointerException {
modelBuilder.setOptionShortLabel(shortLabel);
return modelBuilder;
}
public ConfigUIModelBuilder setOptionEditor(TableCellEditor editor)
throws IllegalStateException, NullPointerException {
modelBuilder.setOptionEditor(editor);
return modelBuilder;
}
public ConfigUIModelBuilder setOptionRenderer(TableCellRenderer renderer)
throws IllegalStateException, NullPointerException {
modelBuilder.setOptionRenderer(renderer);
return modelBuilder;
}
public ConfigUIModelBuilder registerCallBack(String name,
String description,
Icon icon,
Runnable action) {
modelBuilder.registerCallBack(name, description, icon, action);
return modelBuilder;
}
public ConfigUIModelBuilder setOptionCallBack(String name) {
modelBuilder.setOptionCallBack(name);
return modelBuilder;
}
public ConfigUIModelBuilder setModel(ConfigUIModel model) throws IllegalStateException {
modelBuilder.setModel(model);
return modelBuilder;
}
public ConfigUIModelBuilder setCategory(CategoryModel categoryModel)
throws IllegalStateException {
modelBuilder.setCategory(categoryModel);
return modelBuilder;
}
public ConfigUIModelBuilder setOption(OptionModel optionModel)
throws IllegalStateException {
modelBuilder.setOption(optionModel);
return modelBuilder;
}
public ConfigUIModelBuilder setFinalizer(CallBackFinalizer finalizer) {
modelBuilder.setFinalizer(finalizer);
return modelBuilder;
}
public ConfigUIModelBuilder setCloseAction(Runnable runnable) {
modelBuilder.setCloseAction(runnable);
return modelBuilder;
}
/**
* Construire l'ui de configuration (sous forme de panel)
*
* @param parentContext le context applicatif
* @param defaultCategory la categorie a selectionner
* @return l'ui instanciate
*/
public ConfigUI buildUI(JAXXContext parentContext,
String defaultCategory) {
ConfigUIModel model = getModel();
JAXXContext tx = new JAXXInitialContext().add(parentContext).add(model);
ui = new ConfigUI(tx);
ui.init(defaultCategory);
return ui;
}
public void displayUI(Frame parentUI, boolean undecorated) {
Preconditions.checkNotNull(ui, "UI was not build, use before the *buildUI* method");
ui.getHandler().displayUI(parentUI, undecorated);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy