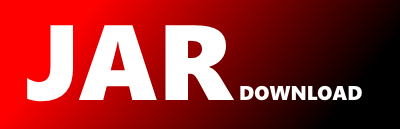
jaxx.demo.component.jaxx.editor.TimeEditorDemo Maven / Gradle / Ivy
package jaxx.demo.component.jaxx.editor;
/*-
* #%L
* JAXX :: Demo
* %%
* Copyright (C) 2008 - 2016 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.BorderLayout;
import java.awt.GridBagConstraints;
import java.awt.Insets;
import java.awt.LayoutManager;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.border.TitledBorder;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import javax.swing.text.Document;
import jaxx.demo.DemoPanel;
import jaxx.runtime.JAXXContext;
import jaxx.runtime.JAXXObjectDescriptor;
import jaxx.runtime.JAXXUtil;
import jaxx.runtime.binding.DefaultJAXXBinding;
import jaxx.runtime.swing.Table;
import jaxx.runtime.swing.editor.TimeEditor;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import static org.nuiton.i18n.I18n.t;
public class TimeEditorDemo extends DemoPanel {
/*-----------------------------------------------------------------------*/
/*------------------ Constants for all public bindings ------------------*/
/*-----------------------------------------------------------------------*/
public static final String BINDING_EDITOR_DATE = "editor.date";
public static final String BINDING_EDITOR_LABEL = "editor.label";
/*-----------------------------------------------------------------------*/
/*--------------- Constants for all none public bindings ---------------*/
/*-----------------------------------------------------------------------*/
private static final String BINDING_$JLABEL0_TEXT = "$JLabel0.text";
/*-----------------------------------------------------------------------*/
/*------------------------- Other static fields -------------------------*/
/*-----------------------------------------------------------------------*/
private static final String $jaxxObjectDescriptor = "H4sIAAAAAAAAAKVUPW8TQRAdm9jxB5CQECsRARnihoJzUEpHISjBgsgBRFJEuGHPt8QXre+W2z1ybhA/gZ8APQ0SHRWioKagQfwFhChoEbN79l0uOZIgu9hbz8x7+2Z2Zt/+gJzw4OoeCQLD8x1p96ixcXtn54G5RztynYqOZ3PpehD+MlnItqFsRXYhYaHdUvD6AF5fc3vcdahzAN1oQUnIPqOiS6mUcDmJ6AhR34rcjYD73pA1EpXG+vrXz+wr6+WbLEDAUV0JU6mehIozGWtB1rYkTOFJz0mdEWcXZXi2s4t6zynbGiNC3Cc9+gxewHgL8px4SCbh2ulT1hwaH3AJ5do67bkPiUPZogRDi7XQYnSGFKGNWjaSGNvIfkdvFYxzTZSXUFSYTdeiTMLS/5FoVMw0XtsmJqMoZjZRObGPdTC0T0UWI0C54zpP7V2dAtZOlSkYRG9oo4qbiMILtdC6qP5fVMtU5JtkxKRsTfO11PYwnzaq4Jn4+AMYCZVE+DYNZNOmzEpC8mEdJNRSMjxSo6T8kkeFz6RKIUogkZyWqJObU0uVYw9eSpyD3W3E3R23X6YNOc9HM5a+fXQgHqErHIXZQ6OgCLX3T2X664fv75vD/i/g2TOpoQfGF/uSey6nnrTV0RNh8/vSZvVNwhttKArKcPb1bM+nCNsauFEcnndBwQ0FN+4S0UWK3Pi3j58qT76cgWwTSswlVpOo+HtQlF0sZ9dlVsBvrWpFZ/cLuE4qbXhRjPRdH8fr/LJFJKmatmPhHa0EWIT5lCJESszi59/TW+9Wh4XIoLC5f4bHxcg9hrztMNuh+ikYTHnq6Je5oL7lxtOcNt8Z9S3xwXRd1+uNo5kqc10ti4GvPje1ZLVb0r7KiQwSxkxKnGM4rpyCI2+6nkW9EVnG8K7oiBw5Npz0EUgKg77u4zOyHF5rlUh80E1f0pUREpT4rhyjbQE5/gJIhxxcSQcAAA==";
private static final Log log = LogFactory.getLog(TimeEditorDemo.class);
private static final long serialVersionUID = 1L;
/*-----------------------------------------------------------------------*/
/*--------------------------- Internal states ---------------------------*/
/*-----------------------------------------------------------------------*/
private boolean allComponentsCreated;
/*-----------------------------------------------------------------------*/
/*------------------------ Protected components ------------------------*/
/*-----------------------------------------------------------------------*/
protected JPanel configPanel;
protected TimeEditorDemoModel demoModel;
protected TimeEditor editor;
protected JTextField labelConfig;
protected JLabel labelConfigLabel;
protected JPanel resultPane;
/*-----------------------------------------------------------------------*/
/*------------------------- Private components -------------------------*/
/*-----------------------------------------------------------------------*/
private TimeEditorDemo $DemoPanel0 = this;
private JLabel $JLabel0;
private JPanel $JPanel0;
private Table $Table0;
/*-----------------------------------------------------------------------*/
/*---------------------- Raw body code from script ----------------------*/
/*-----------------------------------------------------------------------*/
void $afterCompleteSetup() {
// init time editor
editor.init();
// set current time in model
demoModel.setTime(new java.util.Date());
}
/*-----------------------------------------------------------------------*/
/*---------------------------- Constructors ----------------------------*/
/*-----------------------------------------------------------------------*/
public TimeEditorDemo(LayoutManager param0, boolean param1) {
super(param0 ,param1);
$initialize();
}
public TimeEditorDemo(JAXXContext param0, LayoutManager param1, boolean param2) {
super(param0 ,param1 ,param2);
$initialize();
}
public TimeEditorDemo(LayoutManager param0) {
super(param0);
$initialize();
}
public TimeEditorDemo(JAXXContext param0, LayoutManager param1) {
super(param0 ,param1);
$initialize();
}
public TimeEditorDemo() {
$initialize();
}
public TimeEditorDemo(JAXXContext param0) {
super(param0);
$initialize();
}
public TimeEditorDemo(boolean param0) {
super(param0);
$initialize();
}
public TimeEditorDemo(JAXXContext param0, boolean param1) {
super(param0 ,param1);
$initialize();
}
/*-----------------------------------------------------------------------*/
/*--------------------------- Statics methods ---------------------------*/
/*-----------------------------------------------------------------------*/
public static JAXXObjectDescriptor $getJAXXObjectDescriptor() {
return JAXXUtil.decodeCompressedJAXXObjectDescriptor($jaxxObjectDescriptor);
}
/*-----------------------------------------------------------------------*/
/*----------------------- Public acessor methods -----------------------*/
/*-----------------------------------------------------------------------*/
public JPanel getConfigPanel() {
return configPanel;
}
public TimeEditorDemoModel getDemoModel() {
return demoModel;
}
public TimeEditor getEditor() {
return editor;
}
public JTextField getLabelConfig() {
return labelConfig;
}
public JLabel getLabelConfigLabel() {
return labelConfigLabel;
}
public JPanel getResultPane() {
return resultPane;
}
/*-----------------------------------------------------------------------*/
/*--------------------- Protected acessors methods ---------------------*/
/*-----------------------------------------------------------------------*/
protected JLabel get$JLabel0() {
return $JLabel0;
}
protected JPanel get$JPanel0() {
return $JPanel0;
}
protected Table get$Table0() {
return $Table0;
}
/*-----------------------------------------------------------------------*/
/*--------------------- Components creation methods ---------------------*/
/*-----------------------------------------------------------------------*/
protected void addChildrenToConfigPanel() {
if (!allComponentsCreated) {
return;
}
configPanel.add($JPanel0);
}
protected void addChildrenToResultPane() {
if (!allComponentsCreated) {
return;
}
resultPane.add($JLabel0, BorderLayout.CENTER);
}
protected void createConfigPanel() {
$objectMap.put("configPanel", configPanel = new JPanel());
configPanel.setName("configPanel");
}
protected void createDemoModel() {
$objectMap.put("demoModel", demoModel = new TimeEditorDemoModel());
}
protected void createEditor() {
$objectMap.put("editor", editor = new TimeEditor(this));
editor.setName("editor");
editor.setProperty("time");
}
protected void createLabelConfig() {
$objectMap.put("labelConfig", labelConfig = new JTextField());
labelConfig.setName("labelConfig");
labelConfig.setColumns(15);
}
protected void createLabelConfigLabel() {
$objectMap.put("labelConfigLabel", labelConfigLabel = new JLabel());
labelConfigLabel.setName("labelConfigLabel");
}
protected void createResultPane() {
$objectMap.put("resultPane", resultPane = new JPanel());
resultPane.setName("resultPane");
}
/*-----------------------------------------------------------------------*/
/*------------------------ Internal jaxx methods ------------------------*/
/*-----------------------------------------------------------------------*/
private void $completeSetup() {
allComponentsCreated = true;
if (log.isDebugEnabled()) {
log.debug(this);
}
// inline complete setup of $DemoPanel0
add($Table0, BorderLayout.NORTH);
// inline complete setup of $Table0
$Table0.add(configPanel, new GridBagConstraints(0, 0, 1, 1, 1.0, 0.0, 10, 1, new Insets(0, 0, 0, 0), 0, 0));
$Table0.add(editor, new GridBagConstraints(0, 1, 1, 1, 1.0, 0.0, 10, 1, new Insets(0, 0, 0, 0), 0, 0));
$Table0.add(resultPane, new GridBagConstraints(0, 2, 1, 1, 1.0, 0.0, 10, 1, new Insets(0, 0, 0, 0), 0, 0));
addChildrenToConfigPanel();
// inline complete setup of $JPanel0
$JPanel0.add(labelConfigLabel, BorderLayout.WEST);
$JPanel0.add(labelConfig, BorderLayout.CENTER);
addChildrenToResultPane();
// apply 3 data bindings
JAXXUtil.applyDataBinding(this, $bindings.keySet());
// apply 4 property setters
editor.setBean(demoModel);
editor.setBorder(new TitledBorder(t("jaxxdemo.timeeditor.editor")));
$afterCompleteSetup();
}
private void $initialize() {
if (allComponentsCreated) {
return;
}
if (log.isDebugEnabled()) {
log.debug(this);
}
$objectMap.put("$DemoPanel0", $DemoPanel0);
createDemoModel();
// inline creation of $Table0
$objectMap.put("$Table0", $Table0 = new Table());
$Table0.setName("$Table0");
createConfigPanel();
// inline creation of $JPanel0
$objectMap.put("$JPanel0", $JPanel0 = new JPanel());
$JPanel0.setName("$JPanel0");
$JPanel0.setLayout(new BorderLayout());
createLabelConfigLabel();
createLabelConfig();
createEditor();
createResultPane();
// inline creation of $JLabel0
$objectMap.put("$JLabel0", $JLabel0 = new JLabel());
$JLabel0.setName("$JLabel0");
// inline creation of $DemoPanel0
setName("$DemoPanel0");
setLayout(new BorderLayout());
// registers 3 data bindings
$registerDefaultBindings();
$completeSetup();
}
private void $registerDefaultBindings() {
// register 3 data bindings
registerDataBinding(new DefaultJAXXBinding(this, BINDING_EDITOR_DATE, true) {
@Override
public void applyDataBinding() {
if (demoModel != null) {
demoModel.addPropertyChangeListener("time", this);
}
}
@Override
public void processDataBinding() {
if (demoModel != null) {
editor.setDate(demoModel.getTime());
}
}
@Override
public void removeDataBinding() {
if (demoModel != null) {
demoModel.removePropertyChangeListener("time", this);
}
}
});
registerDataBinding(new DefaultJAXXBinding(this, BINDING_EDITOR_LABEL, true) {
@Override
public void applyDataBinding() {
if (labelConfig != null) {
$bindingSources.put("labelConfig.getDocument()", labelConfig.getDocument());
labelConfig.getDocument().addDocumentListener( JAXXUtil.getEventListener(DocumentListener.class, this, "$pr$u0"));
labelConfig.addPropertyChangeListener("document", JAXXUtil.getDataBindingUpdateListener(jaxx.demo.component.jaxx.editor.TimeEditorDemo.this, BINDING_EDITOR_LABEL));
}
}
@Override
public void processDataBinding() {
if (labelConfig != null) {
editor.setLabel(labelConfig.getText());
}
}
@Override
public void removeDataBinding() {
if (labelConfig != null) {
Document $target = (Document) $bindingSources.remove("labelConfig.getDocument()");
if ($target != null) {
$target.removeDocumentListener( JAXXUtil.getEventListener(DocumentListener.class, this, "$pr$u0"));
}
labelConfig.removePropertyChangeListener("document", JAXXUtil.getDataBindingUpdateListener(jaxx.demo.component.jaxx.editor.TimeEditorDemo.this, BINDING_EDITOR_LABEL));
}
}
public void $pr$u0(DocumentEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
propertyChange(null);
}
});
registerDataBinding(new DefaultJAXXBinding(this, BINDING_$JLABEL0_TEXT, true) {
@Override
public void applyDataBinding() {
if (demoModel != null) {
demoModel.addPropertyChangeListener("time", this);
}
}
@Override
public void processDataBinding() {
if (demoModel != null) {
$JLabel0.setText(t(t("jaxxdemo.timeeditor.value", demoModel.getTime())));
}
}
@Override
public void removeDataBinding() {
if (demoModel != null) {
demoModel.removePropertyChangeListener("time", this);
}
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy