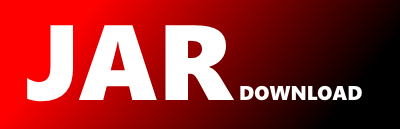
jaxx.demo.component.jaxx.editor.FileEditorDemo Maven / Gradle / Ivy
package jaxx.demo.component.jaxx.editor;
/*
* #%L
* JAXX :: Demo
* $Id:$
* $HeadURL:$
* %%
* Copyright (C) 2008 - 2013 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.BorderLayout;
import java.awt.GridBagConstraints;
import java.awt.Insets;
import java.awt.LayoutManager;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.JCheckBox;
import javax.swing.JLabel;
import javax.swing.JTextField;
import jaxx.demo.DemoPanel;
import jaxx.runtime.JAXXContext;
import jaxx.runtime.JAXXObjectDescriptor;
import jaxx.runtime.JAXXUtil;
import jaxx.runtime.SwingUtil;
import jaxx.runtime.binding.SimpleJAXXObjectBinding;
import jaxx.runtime.swing.Table;
import jaxx.runtime.swing.editor.FileEditor;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import static org.nuiton.i18n.I18n._;
public class FileEditorDemo extends DemoPanel {
/*-----------------------------------------------------------------------*/
/*---------------- Constants for all javaBean properties ----------------*/
/*-----------------------------------------------------------------------*/
public static final String PROPERTY_ACCEPT_ALL_FILE_FILTER_USED = "acceptAllFileFilterUsed";
public static final String PROPERTY_DIRECTORY_ENABLED = "directoryEnabled";
public static final String PROPERTY_EXTS = "exts";
public static final String PROPERTY_EXTS_DESCRIPTION = "extsDescription";
public static final String PROPERTY_FILE_ENABLED = "fileEnabled";
public static final String PROPERTY_TITLE = "title";
/*-----------------------------------------------------------------------*/
/*------------------ Constants for all public bindings ------------------*/
/*-----------------------------------------------------------------------*/
public static final String BINDING_ACCEPT_ALL_FILE_FILTER_USED_FIELD_SELECTED = "acceptAllFileFilterUsedField.selected";
public static final String BINDING_DIRECTORY_ENABLED_FIELD_SELECTED = "directoryEnabledField.selected";
public static final String BINDING_EXTS_DESCRIPTION_FIELD_ENABLED = "extsDescriptionField.enabled";
public static final String BINDING_EXTS_DESCRIPTION_FIELD_TEXT = "extsDescriptionField.text";
public static final String BINDING_EXTS_FIELD_ENABLED = "extsField.enabled";
public static final String BINDING_EXTS_FIELD_TEXT = "extsField.text";
public static final String BINDING_FILE_CHOOSER_ACCEPT_ALL_FILE_FILTER_USED = "fileChooser.acceptAllFileFilterUsed";
public static final String BINDING_FILE_CHOOSER_DIRECTORY_ENABLED = "fileChooser.directoryEnabled";
public static final String BINDING_FILE_CHOOSER_EXTS = "fileChooser.exts";
public static final String BINDING_FILE_CHOOSER_EXTS_DESCRIPTION = "fileChooser.extsDescription";
public static final String BINDING_FILE_CHOOSER_FILE_ENABLED = "fileChooser.fileEnabled";
public static final String BINDING_FILE_CHOOSER_TITLE = "fileChooser.title";
public static final String BINDING_FILE_ENABLED_FIELD_SELECTED = "fileEnabledField.selected";
public static final String BINDING_TITLE_FIELD_TEXT = "titleField.text";
/*-----------------------------------------------------------------------*/
/*------------------------- Other static fields -------------------------*/
/*-----------------------------------------------------------------------*/
private static final String $jaxxObjectDescriptor = "H4sIAAAAAAAAAKVWz08TQRSeVtrSVqGCRVRUwEZjjFsRiTEQBQoVSQUjmBDrwWl3pIvT3XV3KsvF+Cf4J+jdi4k3T8aDZw9ejP+CMR68Gt/MtrttmdKSbdLd7fvxzffevP0673+hiG2hiR3sOIpV05lWJcrqwtbWemmHlNkSscuWZjLDQu4nFEbhIkqqnt1m6EKxwNOz9fRszqiahk70puzZAkrYbI8Su0IIY+hsa0bZtrMbnnvWMWtWA9UjJUN9++d3+I36+l0YIccEdmkoZbxbll9JXwGFNZWhIVjpJc5SrG8DDUvTt4HvMW7LUWzba7hKXqBXKFZAURNbAMbQZO8lCwyR75gMJTNLpGo8wDqh1xhSBFkVLEq5AeHaiKoBiJLXKFkWjzzNNAVQlKEI0xglDKU4S4UzV1zmfkgfcZh4HvBtg9zW4KgZepv7JC6XickWKOXrwpcR65FNoEXH/XUWDYMSrPtZKVWzYFYMa29ZxyVKVG5P+/7kM16E1BXLbHIzdGK0ZdvsXShFET4eGfcS+jOrBVwSvRvilJx6qGvlQae92IToUV4jFAoYaYnehD4IR2tGA32K/z7f6ku3V1kHTrcA5yqk/HzRcOS41yW4qabueJQuyfOnJflxvqVe4qQ88YYk8UTbLHTDmJFgjHUYmE6FiFHIVQzDJhZDGcme75v7OoCFzrREg2QovmT473SoiCJWDcwwUsX9KvMQXK6+jLbpCwcU3n8jw98//fyYbxaVtDS0SRPhZTctw4SqNL70oKsoNabR7H1szhZR3CZUjA94xyTENupuIAfrua8bT1dWsF0BiEjsx+cvI0+/HUHhPEpQA6t5zOPvoTirWNAFg6qOeWdeMDq62w/XFOfGUJTiPaMGmjUwp2KGx0uarkKnbzvQhDFJEzwmpfjXv8MbH+YbjQgBsVMdw/1mRB6jqKZTTSdCX+vSKdXTpGmTmmr4EikTzRC/J8y6asyL65Ks0j4GIw3CMOeuPo4ZSGKpxgjUypNWRBH8aVVgnuuKyc1r/HJXjjDRO8K6HCHTQ1397uy46tmBycXATC73hPDkAA5XAnO42kM3YsT/K+lAJBt4Yw+B0KGUqZ4QqgdwmA5cxSEQOlQxE3gobvawpZ3OHQfA3uoBVnowCYDnnagCYMhOYAHg2s9WAaDcI2UXkP9L2yvZMAwAAA==";
private static final Log log = LogFactory.getLog(FileEditorDemo.class);
private static final long serialVersionUID = 1L;
/*-----------------------------------------------------------------------*/
/*--------------------------- Internal states ---------------------------*/
/*-----------------------------------------------------------------------*/
private boolean allComponentsCreated;
/*-----------------------------------------------------------------------*/
/*------------------------ Protected components ------------------------*/
/*-----------------------------------------------------------------------*/
protected Boolean acceptAllFileFilterUsed;
protected JCheckBox acceptAllFileFilterUsedField;
protected Boolean directoryEnabled;
protected JCheckBox directoryEnabledField;
protected String exts;
protected String extsDescription;
protected JTextField extsDescriptionField;
protected JTextField extsField;
protected FileEditor fileChooser;
protected Boolean fileEnabled;
protected JCheckBox fileEnabledField;
protected String title;
protected JTextField titleField;
/*-----------------------------------------------------------------------*/
/*------------------------- Private components -------------------------*/
/*-----------------------------------------------------------------------*/
private FileEditorDemo $DemoPanel0 = this;
private JLabel $JLabel0;
private JLabel $JLabel1;
private JLabel $JLabel2;
private JLabel $JLabel3;
private JLabel $JLabel4;
private JLabel $JLabel5;
private Table $Table0;
/*-----------------------------------------------------------------------*/
/*---------------------------- Constructors ----------------------------*/
/*-----------------------------------------------------------------------*/
public FileEditorDemo(LayoutManager param0, boolean param1) {
super(param0 ,param1);
$initialize();
}
public FileEditorDemo(JAXXContext param0, LayoutManager param1, boolean param2) {
super(param0 ,param1 ,param2);
$initialize();
}
public FileEditorDemo() {
$initialize();
}
public FileEditorDemo(JAXXContext param0) {
super(param0);
$initialize();
}
public FileEditorDemo(LayoutManager param0) {
super(param0);
$initialize();
}
public FileEditorDemo(JAXXContext param0, LayoutManager param1) {
super(param0 ,param1);
$initialize();
}
public FileEditorDemo(boolean param0) {
super(param0);
$initialize();
}
public FileEditorDemo(JAXXContext param0, boolean param1) {
super(param0 ,param1);
$initialize();
}
/*-----------------------------------------------------------------------*/
/*--------------------------- Statics methods ---------------------------*/
/*-----------------------------------------------------------------------*/
public static JAXXObjectDescriptor $getJAXXObjectDescriptor() {
return JAXXUtil.decodeCompressedJAXXObjectDescriptor($jaxxObjectDescriptor);
}
/*-----------------------------------------------------------------------*/
/*---------------------------- Event methods ----------------------------*/
/*-----------------------------------------------------------------------*/
public void doActionPerformed__on__acceptAllFileFilterUsedField(ActionEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
setAcceptAllFileFilterUsed(acceptAllFileFilterUsedField.isSelected());
}
public void doActionPerformed__on__directoryEnabledField(ActionEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
setDirectoryEnabled(directoryEnabledField.isSelected());
}
public void doActionPerformed__on__fileEnabledField(ActionEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
setFileEnabled(fileEnabledField.isSelected());
}
public void doKeyReleased__on__extsDescriptionField(KeyEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
setExtsDescription(extsDescriptionField.getText());
}
public void doKeyReleased__on__extsField(KeyEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
setExts(extsField.getText());
}
public void doKeyReleased__on__titleField(KeyEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
setTitle(titleField.getText());
}
/*-----------------------------------------------------------------------*/
/*----------------------- Public acessor methods -----------------------*/
/*-----------------------------------------------------------------------*/
public Boolean getAcceptAllFileFilterUsed() {
return acceptAllFileFilterUsed;
}
public JCheckBox getAcceptAllFileFilterUsedField() {
return acceptAllFileFilterUsedField;
}
public Boolean getDirectoryEnabled() {
return directoryEnabled;
}
public JCheckBox getDirectoryEnabledField() {
return directoryEnabledField;
}
public String getExts() {
return exts;
}
public String getExtsDescription() {
return extsDescription;
}
public JTextField getExtsDescriptionField() {
return extsDescriptionField;
}
public JTextField getExtsField() {
return extsField;
}
public FileEditor getFileChooser() {
return fileChooser;
}
public Boolean getFileEnabled() {
return fileEnabled;
}
public JCheckBox getFileEnabledField() {
return fileEnabledField;
}
public String getTitle() {
return title;
}
public JTextField getTitleField() {
return titleField;
}
public Boolean isAcceptAllFileFilterUsed() {
return acceptAllFileFilterUsed !=null && acceptAllFileFilterUsed;
}
public Boolean isDirectoryEnabled() {
return directoryEnabled !=null && directoryEnabled;
}
public Boolean isFileEnabled() {
return fileEnabled !=null && fileEnabled;
}
/*-----------------------------------------------------------------------*/
/*----------------------- Public mutator methods -----------------------*/
/*-----------------------------------------------------------------------*/
public void setAcceptAllFileFilterUsed(Boolean acceptAllFileFilterUsed) {
Boolean oldValue = this.acceptAllFileFilterUsed;
this.acceptAllFileFilterUsed = acceptAllFileFilterUsed;
firePropertyChange(PROPERTY_ACCEPT_ALL_FILE_FILTER_USED, oldValue, acceptAllFileFilterUsed);
}
public void setDirectoryEnabled(Boolean directoryEnabled) {
Boolean oldValue = this.directoryEnabled;
this.directoryEnabled = directoryEnabled;
firePropertyChange(PROPERTY_DIRECTORY_ENABLED, oldValue, directoryEnabled);
}
public void setExts(String exts) {
String oldValue = this.exts;
this.exts = exts;
firePropertyChange(PROPERTY_EXTS, oldValue, exts);
}
public void setExtsDescription(String extsDescription) {
String oldValue = this.extsDescription;
this.extsDescription = extsDescription;
firePropertyChange(PROPERTY_EXTS_DESCRIPTION, oldValue, extsDescription);
}
public void setFileEnabled(Boolean fileEnabled) {
Boolean oldValue = this.fileEnabled;
this.fileEnabled = fileEnabled;
firePropertyChange(PROPERTY_FILE_ENABLED, oldValue, fileEnabled);
}
public void setTitle(String title) {
String oldValue = this.title;
this.title = title;
firePropertyChange(PROPERTY_TITLE, oldValue, title);
}
/*-----------------------------------------------------------------------*/
/*--------------------- Protected acessors methods ---------------------*/
/*-----------------------------------------------------------------------*/
protected JLabel get$JLabel0() {
return $JLabel0;
}
protected JLabel get$JLabel1() {
return $JLabel1;
}
protected JLabel get$JLabel2() {
return $JLabel2;
}
protected JLabel get$JLabel3() {
return $JLabel3;
}
protected JLabel get$JLabel4() {
return $JLabel4;
}
protected JLabel get$JLabel5() {
return $JLabel5;
}
protected Table get$Table0() {
return $Table0;
}
/*-----------------------------------------------------------------------*/
/*--------------------- Components creation methods ---------------------*/
/*-----------------------------------------------------------------------*/
protected void createAcceptAllFileFilterUsed() {
$objectMap.put("acceptAllFileFilterUsed", acceptAllFileFilterUsed = Boolean.TRUE);
}
protected void createAcceptAllFileFilterUsedField() {
$objectMap.put("acceptAllFileFilterUsedField", acceptAllFileFilterUsedField = new JCheckBox());
acceptAllFileFilterUsedField.setName("acceptAllFileFilterUsedField");
acceptAllFileFilterUsedField.addActionListener(JAXXUtil.getEventListener(ActionListener.class, "actionPerformed", this, "doActionPerformed__on__acceptAllFileFilterUsedField"));
}
protected void createDirectoryEnabled() {
$objectMap.put("directoryEnabled", directoryEnabled = Boolean.TRUE);
}
protected void createDirectoryEnabledField() {
$objectMap.put("directoryEnabledField", directoryEnabledField = new JCheckBox());
directoryEnabledField.setName("directoryEnabledField");
directoryEnabledField.addActionListener(JAXXUtil.getEventListener(ActionListener.class, "actionPerformed", this, "doActionPerformed__on__directoryEnabledField"));
}
protected void createExts() {
$objectMap.put("exts", exts = "txt, png");
}
protected void createExtsDescription() {
$objectMap.put("extsDescription", extsDescription = "Text (*.txt), Image (*.png)");
}
protected void createExtsDescriptionField() {
$objectMap.put("extsDescriptionField", extsDescriptionField = new JTextField());
extsDescriptionField.setName("extsDescriptionField");
extsDescriptionField.setColumns(15);
extsDescriptionField.addKeyListener(JAXXUtil.getEventListener(KeyListener.class, "keyReleased", this, "doKeyReleased__on__extsDescriptionField"));
}
protected void createExtsField() {
$objectMap.put("extsField", extsField = new JTextField());
extsField.setName("extsField");
extsField.setColumns(15);
extsField.addKeyListener(JAXXUtil.getEventListener(KeyListener.class, "keyReleased", this, "doKeyReleased__on__extsField"));
}
protected void createFileChooser() {
$objectMap.put("fileChooser", fileChooser = new FileEditor());
fileChooser.setName("fileChooser");
}
protected void createFileEnabled() {
$objectMap.put("fileEnabled", fileEnabled = Boolean.FALSE);
}
protected void createFileEnabledField() {
$objectMap.put("fileEnabledField", fileEnabledField = new JCheckBox());
fileEnabledField.setName("fileEnabledField");
fileEnabledField.addActionListener(JAXXUtil.getEventListener(ActionListener.class, "actionPerformed", this, "doActionPerformed__on__fileEnabledField"));
}
protected void createTitle() {
$objectMap.put("title", title = "Open file");
}
protected void createTitleField() {
$objectMap.put("titleField", titleField = new JTextField());
titleField.setName("titleField");
titleField.setColumns(15);
titleField.addKeyListener(JAXXUtil.getEventListener(KeyListener.class, "keyReleased", this, "doKeyReleased__on__titleField"));
}
/*-----------------------------------------------------------------------*/
/*------------------------ Internal jaxx methods ------------------------*/
/*-----------------------------------------------------------------------*/
private void $completeSetup() {
allComponentsCreated = true;
if (log.isDebugEnabled()) {
log.debug(this);
}
// inline complete setup of $DemoPanel0
add($Table0, BorderLayout.CENTER);
// inline complete setup of $Table0
$Table0.add($JLabel0, new GridBagConstraints(0, 0, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(titleField, new GridBagConstraints(1, 0, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add($JLabel1, new GridBagConstraints(0, 1, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(directoryEnabledField, new GridBagConstraints(1, 1, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add($JLabel2, new GridBagConstraints(0, 2, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(fileEnabledField, new GridBagConstraints(1, 2, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add($JLabel3, new GridBagConstraints(0, 3, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(extsField, new GridBagConstraints(1, 3, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add($JLabel4, new GridBagConstraints(0, 4, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(extsDescriptionField, new GridBagConstraints(1, 4, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add($JLabel5, new GridBagConstraints(0, 5, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(acceptAllFileFilterUsedField, new GridBagConstraints(1, 5, 1, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
$Table0.add(fileChooser, new GridBagConstraints(0, 6, 2, 1, 1.0, 0.0, 10, 2, new Insets(3, 3, 3, 3), 0, 0));
// apply 14 data bindings
JAXXUtil.applyDataBinding(this, $bindings.keySet());
}
private void $initialize() {
if (allComponentsCreated) {
return;
}
if (log.isDebugEnabled()) {
log.debug(this);
}
$objectMap.put("$DemoPanel0", $DemoPanel0);
createTitle();
createExts();
createExtsDescription();
createAcceptAllFileFilterUsed();
createDirectoryEnabled();
createFileEnabled();
// inline creation of $Table0
$objectMap.put("$Table0", $Table0 = new Table());
$Table0.setName("$Table0");
// inline creation of $JLabel0
$objectMap.put("$JLabel0", $JLabel0 = new JLabel());
$JLabel0.setName("$JLabel0");
$JLabel0.setText(_("jaxxdemo.fileEditor.titleLbl"));
createTitleField();
// inline creation of $JLabel1
$objectMap.put("$JLabel1", $JLabel1 = new JLabel());
$JLabel1.setName("$JLabel1");
$JLabel1.setText(_("jaxxdemo.fileEditor.directoryEnabled"));
createDirectoryEnabledField();
// inline creation of $JLabel2
$objectMap.put("$JLabel2", $JLabel2 = new JLabel());
$JLabel2.setName("$JLabel2");
$JLabel2.setText(_("jaxxdemo.fileEditor.fileEnabled"));
createFileEnabledField();
// inline creation of $JLabel3
$objectMap.put("$JLabel3", $JLabel3 = new JLabel());
$JLabel3.setName("$JLabel3");
$JLabel3.setText(_("jaxxdemo.fileEditor.extsLbl"));
createExtsField();
// inline creation of $JLabel4
$objectMap.put("$JLabel4", $JLabel4 = new JLabel());
$JLabel4.setName("$JLabel4");
$JLabel4.setText(_("jaxxdemo.fileEditor.extsDescLbl"));
createExtsDescriptionField();
// inline creation of $JLabel5
$objectMap.put("$JLabel5", $JLabel5 = new JLabel());
$JLabel5.setName("$JLabel5");
$JLabel5.setText(_("jaxxdemo.fileEditor.acceptAllFileFilterUsed"));
createAcceptAllFileFilterUsedField();
createFileChooser();
// inline creation of $DemoPanel0
setName("$DemoPanel0");
setLayout(new BorderLayout());
// registers 14 data bindings
$registerDefaultBindings();
$completeSetup();
}
private void $registerDefaultBindings() {
// register 14 data bindings
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_TITLE_FIELD_TEXT, true ,"title") {
@Override
public void processDataBinding() {
SwingUtil.setText(titleField, getTitle());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_DIRECTORY_ENABLED_FIELD_SELECTED, true ,"directoryEnabled") {
@Override
public void processDataBinding() {
directoryEnabledField.setSelected(isDirectoryEnabled());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_ENABLED_FIELD_SELECTED, true ,"fileEnabled") {
@Override
public void processDataBinding() {
fileEnabledField.setSelected(isFileEnabled());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_EXTS_FIELD_ENABLED, true ,"fileEnabled") {
@Override
public void processDataBinding() {
extsField.setEnabled(isFileEnabled());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_EXTS_FIELD_TEXT, true ,"exts") {
@Override
public void processDataBinding() {
SwingUtil.setText(extsField, getExts());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_EXTS_DESCRIPTION_FIELD_ENABLED, true ,"fileEnabled") {
@Override
public void processDataBinding() {
extsDescriptionField.setEnabled(isFileEnabled());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_EXTS_DESCRIPTION_FIELD_TEXT, true ,"extsDescription") {
@Override
public void processDataBinding() {
SwingUtil.setText(extsDescriptionField, getExtsDescription());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_ACCEPT_ALL_FILE_FILTER_USED_FIELD_SELECTED, true ,"acceptAllFileFilterUsed") {
@Override
public void processDataBinding() {
acceptAllFileFilterUsedField.setSelected(isAcceptAllFileFilterUsed());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_CHOOSER_ACCEPT_ALL_FILE_FILTER_USED, true ,"acceptAllFileFilterUsed") {
@Override
public void processDataBinding() {
fileChooser.setAcceptAllFileFilterUsed(isAcceptAllFileFilterUsed());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_CHOOSER_DIRECTORY_ENABLED, true ,"directoryEnabled") {
@Override
public void processDataBinding() {
fileChooser.setDirectoryEnabled(isDirectoryEnabled());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_CHOOSER_EXTS, true ,"exts") {
@Override
public void processDataBinding() {
fileChooser.setExts(getExts());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_CHOOSER_EXTS_DESCRIPTION, true ,"extsDescription") {
@Override
public void processDataBinding() {
fileChooser.setExtsDescription(getExtsDescription());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_CHOOSER_FILE_ENABLED, true ,"fileEnabled") {
@Override
public void processDataBinding() {
fileChooser.setFileEnabled(isFileEnabled());
}
});
registerDataBinding(new SimpleJAXXObjectBinding(this, BINDING_FILE_CHOOSER_TITLE, true ,"title") {
@Override
public void processDataBinding() {
fileChooser.setTitle(_(getTitle()));
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy