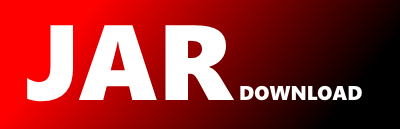
jaxx.demo.I18nEditorDemo Maven / Gradle / Ivy
package jaxx.demo;
import jaxx.demo.*;
import java.awt.*;
import java.awt.event.*;
import java.beans.*;
import java.io.*;
import java.lang.*;
import java.util.*;
import javax.swing.*;
import javax.swing.border.*;
import javax.swing.event.*;
import jaxx.runtime.swing.JAXXButtonGroup;
import jaxx.runtime.swing.HBox;
import jaxx.runtime.swing.VBox;
import jaxx.runtime.swing.Table;
import static org.nuiton.i18n.I18n._;
import static jaxx.runtime.Util.createImageIcon;
public class I18nEditorDemo extends jaxx.demo.DemoPanel {
private static java.lang.String $jaxxObjectDescriptor = "H4sIAAAAAAAAAKVUTW/TQBCdhKZN0xYaCFGFCgq04oDAafkQiFZ89UO0SguilajIhU28alxt7MW7adwLQuKKUE8cuAB3jtwR4siJK/8BIf4Bs2s7TsFKLJrDJhnPe/Pe6o0//oSMcOHsDvE8w23Z0mpSY/Xu1taD2g6ty0Uq6q7FpeOC/0mlIV2FEbNTFxKmqhUFLwfw8oLT5I5N7S70XAVyQu4xKhqUSgknfURdiPJGpzzn8ZYbsnXExLG9//0rvW+++JAG8DiqyqGFUj9U5GCgAmnLlHAcJ+2SMiP2NspwLXsbdY6p2gIjQqyTJn0Gz2GoAoOcuEgm4Vxyq5pD4z0uYWR6kTadh8SmbEbChBZrYsVYmb1hL5kWIlUD5xoyKGHYDPvD9tCbaKNSY5PUGI3as9OrFVKjbBZtKQde0OZXVdNopzfPnDphdKnJ5Z4/WsJ0zAiqn3UpPEgTjrys/ucPPhsNRnRghXjslRhs0cc+tmRj3dmknuzLcrUfy0rdsf9lceGEdo0xNKIYRjlJVSHjtrAsYbwaJfYRlvysBlVNoKvvXr7dffPp880wmGM4Y+xAS9ceYVC463DqSkuNOOansSUtVl4jfK4Kw4IyXEK9ZIUuARtBGUUgf17BDAUz7hPRQGhm6MeXr8Wn349AehlyzCHmMlH9KzAsGy66dJjp8dt3tJLRdhbPcaUJL5Sp61xWgTg6bxJJSjXLNjELtzy0W+iy29Hwbf/V2uTr09dDyylf0l9tke3MExi0bGbZVG9hsGCxWzfCBW2ZTrRIcauVUt/jPNiE8/q8EOdtQGKUcDvm/eklInHlay1J0ZsCGVq8+jWTkFOVL6rjUjxDMRGDDu+1/2WQMOQHXfQQMnFoK6cObSUZw70eGiYTXEYWw91W74weSvrz9LuNM4e+jWQMvW6jlPQ21LuvhxLF8wdCSCoOBggAAA==";
protected jaxx.runtime.swing.editor.I18nEditor localeEditor;
protected jaxx.runtime.swing.editor.I18nEditor localeEmptyEditor;
protected jaxx.runtime.swing.editor.I18nEditor localeWithNoIconEditor;
protected jaxx.runtime.swing.editor.I18nEditor localeWithNoTextEditor;
private jaxx.demo.I18nEditorDemo $DemoPanel0 = this;
private javax.swing.JLabel $JLabel1;
private javax.swing.JLabel $JLabel2;
private javax.swing.JLabel $JLabel3;
private javax.swing.JLabel $JLabel4;
private boolean allComponentsCreated;
private boolean contextInitialized = true;
java.util.Map $previousValues = new java.util.HashMap();
/*---------------------------------------------------------------------------------*/
/*-- Statics methods --------------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public static jaxx.runtime.JAXXObjectDescriptor $getJAXXObjectDescriptor() {
return jaxx.runtime.Util.decodeCompressedJAXXObjectDescriptor($jaxxObjectDescriptor);
}
/*---------------------------------------------------------------------------------*/
/*-- Constructors -----------------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public I18nEditorDemo() {
super();
$initialize();
}
public I18nEditorDemo(jaxx.runtime.JAXXContext parentContext) {
super(parentContext);
$initialize();
}
/*---------------------------------------------------------------------------------*/
/*-- JAXXObject implementation ----------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public void applyDataBinding(String $binding) {
super.applyDataBinding($binding);
processDataBinding($binding);
}
public void processDataBinding(String $dest, boolean $force) {
super.processDataBinding($dest, true);
}
public void removeDataBinding(String $binding) {
super.removeDataBinding($binding);
}
/*---------------------------------------------------------------------------------*/
/*-- public acessor methods -------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public jaxx.runtime.swing.editor.I18nEditor getLocaleEditor() {
return localeEditor;
}
public jaxx.runtime.swing.editor.I18nEditor getLocaleEmptyEditor() {
return localeEmptyEditor;
}
public jaxx.runtime.swing.editor.I18nEditor getLocaleWithNoIconEditor() {
return localeWithNoIconEditor;
}
public jaxx.runtime.swing.editor.I18nEditor getLocaleWithNoTextEditor() {
return localeWithNoTextEditor;
}
/*---------------------------------------------------------------------------------*/
/*-- protected acessors methods ---------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
protected jaxx.demo.I18nEditorDemo get$DemoPanel0() {
return $DemoPanel0;
}
protected javax.swing.JLabel get$JLabel1() {
return $JLabel1;
}
protected javax.swing.JLabel get$JLabel2() {
return $JLabel2;
}
protected javax.swing.JLabel get$JLabel3() {
return $JLabel3;
}
protected javax.swing.JLabel get$JLabel4() {
return $JLabel4;
}
protected java.lang.String get$jaxxObjectDescriptor() {
return $jaxxObjectDescriptor;
}
protected java.util.Map get$previousValues() {
return $previousValues;
}
protected boolean getAllComponentsCreated() {
return allComponentsCreated;
}
protected boolean getContextInitialized() {
return contextInitialized;
}
/*---------------------------------------------------------------------------------*/
/*-- ui creation methods ----------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
private void $completeSetup() {
allComponentsCreated = true;
addChildrenToDemoPanel();
$JLabel1.setLabelFor((localeEmptyEditor));
$JLabel2.setLabelFor((localeEditor));
localeEditor.setLocales((java.util.Arrays.asList(org.nuiton.i18n.I18n.getLoader().getLocales())));
$JLabel3.setLabelFor((localeWithNoTextEditor));
localeWithNoTextEditor.setLocales((java.util.Arrays.asList(org.nuiton.i18n.I18n.getLoader().getLocales())));
$JLabel4.setLabelFor((localeWithNoIconEditor));
localeWithNoIconEditor.setLocales((java.util.Arrays.asList(org.nuiton.i18n.I18n.getLoader().getLocales())));
}
private void $initialize() {
if (allComponentsCreated || !contextInitialized) {
return;
}
$objectMap.put("$DemoPanel0", this);
$JLabel1 = new javax.swing.JLabel();
$objectMap.put("$JLabel1", $JLabel1);
$JLabel1.setName("$JLabel1");
$JLabel1.setText(_("Empty I18n editor:"));
createLocaleEmptyEditor();
$JLabel2 = new javax.swing.JLabel();
$objectMap.put("$JLabel2", $JLabel2);
$JLabel2.setName("$JLabel2");
$JLabel2.setText(_("I18n editor:"));
createLocaleEditor();
$JLabel3 = new javax.swing.JLabel();
$objectMap.put("$JLabel3", $JLabel3);
$JLabel3.setName("$JLabel3");
$JLabel3.setText(_("I18n editor with no text :"));
createLocaleWithNoTextEditor();
$JLabel4 = new javax.swing.JLabel();
$objectMap.put("$JLabel4", $JLabel4);
$JLabel4.setName("$JLabel4");
$JLabel4.setText(_("I18n editor with no icon :"));
createLocaleWithNoIconEditor();
$DemoPanel0.removeDataBinding("top.name");
$DemoPanel0.setName("$DemoPanel0");
$completeSetup();
}
protected void addChildrenToDemoPanel() {
if (!allComponentsCreated) {
return;
}
demoPanel.add($JLabel1, new GridBagConstraints(0, 0, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add(localeEmptyEditor, new GridBagConstraints(1, 0, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add($JLabel2, new GridBagConstraints(0, 1, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add(localeEditor, new GridBagConstraints(1, 1, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add($JLabel3, new GridBagConstraints(0, 2, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add(localeWithNoTextEditor, new GridBagConstraints(1, 2, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add($JLabel4, new GridBagConstraints(0, 3, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
demoPanel.add(localeWithNoIconEditor, new GridBagConstraints(1, 3, 1, 1, 0.0, 0.0, 10, 1, new Insets(3, 3, 3, 3), 0, 0));
}
protected void createDemoPanel() {
demoPanel = new jaxx.runtime.swing.Table();
$objectMap.put("demoPanel", demoPanel);
demoPanel.setName("demoPanel");
}
protected void createLocaleEditor() {
localeEditor = new jaxx.runtime.swing.editor.I18nEditor();
$objectMap.put("localeEditor", localeEditor);
localeEditor.removeDataBinding("content.name");
localeEditor.setName("localeEditor");
localeEditor.removeDataBinding("content.locales");
}
protected void createLocaleEmptyEditor() {
localeEmptyEditor = new jaxx.runtime.swing.editor.I18nEditor();
$objectMap.put("localeEmptyEditor", localeEmptyEditor);
localeEmptyEditor.removeDataBinding("content.name");
localeEmptyEditor.setName("localeEmptyEditor");
}
protected void createLocaleWithNoIconEditor() {
localeWithNoIconEditor = new jaxx.runtime.swing.editor.I18nEditor();
$objectMap.put("localeWithNoIconEditor", localeWithNoIconEditor);
localeWithNoIconEditor.removeDataBinding("content.name");
localeWithNoIconEditor.setName("localeWithNoIconEditor");
localeWithNoIconEditor.removeDataBinding("content.locales");
localeWithNoIconEditor.removeDataBinding("content.showIcon");
localeWithNoIconEditor.setShowIcon(false);
}
protected void createLocaleWithNoTextEditor() {
localeWithNoTextEditor = new jaxx.runtime.swing.editor.I18nEditor();
$objectMap.put("localeWithNoTextEditor", localeWithNoTextEditor);
localeWithNoTextEditor.removeDataBinding("content.name");
localeWithNoTextEditor.setName("localeWithNoTextEditor");
localeWithNoTextEditor.removeDataBinding("content.locales");
localeWithNoTextEditor.removeDataBinding("content.showText");
localeWithNoTextEditor.setShowText(false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy