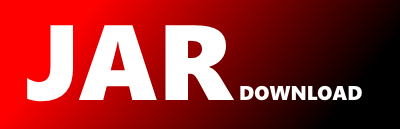
jaxx.demo.JRadioButtonDemo Maven / Gradle / Ivy
package jaxx.demo;
import jaxx.demo.*;
import java.awt.*;
import java.awt.event.*;
import java.beans.*;
import java.io.*;
import java.lang.*;
import java.util.*;
import javax.swing.*;
import javax.swing.border.*;
import javax.swing.event.*;
import jaxx.runtime.swing.JAXXButtonGroup;
import jaxx.runtime.swing.HBox;
import jaxx.runtime.swing.VBox;
import jaxx.runtime.swing.Table;
import static org.nuiton.i18n.I18n._;
import static jaxx.runtime.Util.createImageIcon;
public class JRadioButtonDemo extends jaxx.demo.DemoPanel {
private static java.lang.String $jaxxObjectDescriptor = "H4sIAAAAAAAAAKVUTWsTURS9iU2aNkm/raUo1JqFoEz8pGBLNW2ptaQqLUgxG18yj2bKZN7zzZt0dCGCW5GuXLhR9y7di7h05db/IOI/8L43SWYiYxpIFjPJnXPPPefNufn0C1KugPOHxPcN4TnSalBju7S//6B6SGtyg7o1YXHJBASfRBKSFcianbor4UKlrNqLrfbiOmtw5lAn0r1chlFXPrOpW6dUSjgddNRct7jXKS/73BNtto6YOLYPf34nj82XH5MAPkdVGbSwcFJX6GCoDEnLlDCFk5qkaBPnAGUIyzlAnXlVW7eJ694nDfoUXsBwGdKcCCSTsNi/Vc2h+30uIVvYoA32kDjUviJhXos1sWJs7xLTYmuelMxREM51U1rCiNnukHCmy517hFqNrTXmh+h04RH+vhoPVY8ULteBjxWig69JmFO+/RY++kw1jP+n77qqzvRC3IhBZArbZVKl9k18BV1TdbVbZ06EXJi1xRhzKq4B4K5gHscjETCtcZgvI8xXGIBEBVLCw7KEiUoYxV0sBSFsVTWBrr5/9a759vOXW+3E5XFGvgsSWRBMABeMUyEtNWI8iJknLbu4Q/hyBUZcauN26e2ZiQjYa5VRBPJPqjZDtRlbxK1ja2r459dvs09+nILkJozajJibROHvwYisC3TJbNPnt+9oJbmjDF4nlCYJ03UmrOfMkcQu2daB09BpnlqxHNty6AKRGP+qJ+mqj+5nIu47kr4fv945++bcUvsEEoHCf2DhKaQeQzpg19vWWqTY7cpyl3omCxcmboUS6p7nrcAX9PVinNXJpjr4WsSogl7yPXW7rJWrb0bfhNlqGK4eVNN9UGWCF0/NAXmGJPV72eqHI9UktkcHIlHlpR4Ms30xlAZm2ByYoZeLuYFd9MfQy8XJDBgKq4YLDmMrJpFkoWo5Jv47rsbzzSPfX+NsLLT8BwAA";
protected jaxx.runtime.swing.JAXXButtonGroup radioButtons;
private jaxx.demo.JRadioButtonDemo $DemoPanel0 = this;
private javax.swing.JLabel $JLabel5;
private javax.swing.JRadioButton $JRadioButton2;
private javax.swing.JRadioButton $JRadioButton3;
private javax.swing.JRadioButton $JRadioButton4;
private jaxx.runtime.swing.VBox $VBox1;
private boolean allComponentsCreated;
private boolean contextInitialized = true;
java.util.Map $previousValues = new java.util.HashMap();
private java.beans.PropertyChangeListener $DataSource6 = new jaxx.runtime.DataBindingListener($DemoPanel0, "$JLabel5.icon");
/*---------------------------------------------------------------------------------*/
/*-- Statics methods --------------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public static jaxx.runtime.JAXXObjectDescriptor $getJAXXObjectDescriptor() {
return jaxx.runtime.Util.decodeCompressedJAXXObjectDescriptor($jaxxObjectDescriptor);
}
/*---------------------------------------------------------------------------------*/
/*-- Constructors -----------------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public JRadioButtonDemo() {
super();
$initialize();
}
public JRadioButtonDemo(jaxx.runtime.JAXXContext parentContext) {
super(parentContext);
$initialize();
}
/*---------------------------------------------------------------------------------*/
/*-- JAXXObject implementation ----------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public void applyDataBinding(String $binding) {
if ("$JLabel5.icon".equals($binding)) {
if (radioButtons != null) {
radioButtons.addPropertyChangeListener("selectedValue", $DataSource6);
}
} else {
super.applyDataBinding($binding);
return;
}
processDataBinding($binding);
}
public void processDataBinding(String $dest, boolean $force) {
if (!$force && $activeBindings.contains($dest)) {
return;
}
$activeBindings.add($dest);
try {
if ("$JLabel5.icon".equals($dest)) {
if (radioButtons != null) {
$JLabel5.setIcon((new ImageIcon(getClass().getResource("images/" + radioButtons.getSelectedValue()))));
}
} else {
super.processDataBinding($dest, true);
}
} finally {
$activeBindings.remove($dest);
}
}
public void removeDataBinding(String $binding) {
if ("$JLabel5.icon".equals($binding)) {
if (radioButtons != null) {
radioButtons.removePropertyChangeListener("selectedValue", $DataSource6);
}
} else {
super.removeDataBinding($binding);
}
}
/*---------------------------------------------------------------------------------*/
/*-- public acessor methods -------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
public jaxx.runtime.swing.JAXXButtonGroup getRadioButtons() {
return radioButtons;
}
/*---------------------------------------------------------------------------------*/
/*-- protected acessors methods ---------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
protected jaxx.demo.JRadioButtonDemo get$DemoPanel0() {
return $DemoPanel0;
}
protected javax.swing.JLabel get$JLabel5() {
return $JLabel5;
}
protected javax.swing.JRadioButton get$JRadioButton2() {
return $JRadioButton2;
}
protected javax.swing.JRadioButton get$JRadioButton3() {
return $JRadioButton3;
}
protected javax.swing.JRadioButton get$JRadioButton4() {
return $JRadioButton4;
}
protected jaxx.runtime.swing.VBox get$VBox1() {
return $VBox1;
}
protected java.lang.String get$jaxxObjectDescriptor() {
return $jaxxObjectDescriptor;
}
protected java.util.Map get$previousValues() {
return $previousValues;
}
protected boolean getAllComponentsCreated() {
return allComponentsCreated;
}
protected boolean getContextInitialized() {
return contextInitialized;
}
/*---------------------------------------------------------------------------------*/
/*-- ui creation methods ----------------------------------------------------------*/
/*---------------------------------------------------------------------------------*/
private void $completeSetup() {
allComponentsCreated = true;
addChildrenToDemoPanel();
$VBox1.add($JRadioButton2);
$VBox1.add($JRadioButton3);
$VBox1.add($JRadioButton4);
{ javax.swing.ButtonGroup $buttonGroup = radioButtons; $JRadioButton2.putClientProperty("$buttonGroup", $buttonGroup); $buttonGroup.add($JRadioButton2); }
{ javax.swing.ButtonGroup $buttonGroup = radioButtons; $JRadioButton3.putClientProperty("$buttonGroup", $buttonGroup); $buttonGroup.add($JRadioButton3); }
{ javax.swing.ButtonGroup $buttonGroup = radioButtons; $JRadioButton4.putClientProperty("$buttonGroup", $buttonGroup); $buttonGroup.add($JRadioButton4); }
applyDataBinding("$JLabel5.icon");
}
private void $initialize() {
if (allComponentsCreated || !contextInitialized) {
return;
}
$objectMap.put("$DemoPanel0", this);
$VBox1 = new jaxx.runtime.swing.VBox();
$objectMap.put("$VBox1", $VBox1);
$VBox1.setName("$VBox1");
create$JRadioButton2();
create$JRadioButton3();
create$JRadioButton4();
$JLabel5 = new javax.swing.JLabel();
$objectMap.put("$JLabel5", $JLabel5);
$JLabel5.setName("$JLabel5");
createRadioButtons();
$DemoPanel0.removeDataBinding("top.name");
$DemoPanel0.setName("$DemoPanel0");
$completeSetup();
}
protected void addChildrenToDemoPanel() {
if (!allComponentsCreated) {
return;
}
demoPanel.add($VBox1);
demoPanel.add($JLabel5);
}
protected void create$JRadioButton2() {
$JRadioButton2 = new javax.swing.JRadioButton();
$objectMap.put("$JRadioButton2", $JRadioButton2);
$JRadioButton2.setName("$JRadioButton2");
$JRadioButton2.setSelected(true);
$JRadioButton2.setText(_("Animal"));
{ $JRadioButton2.putClientProperty("$value", "Lynx.jpg"); Object $buttonGroup = $JRadioButton2.getClientProperty("$buttonGroup"); if ($buttonGroup instanceof jaxx.runtime.swing.JAXXButtonGroup) { ((jaxx.runtime.swing.JAXXButtonGroup) $buttonGroup).updateSelectedValue(); } }
}
protected void create$JRadioButton3() {
$JRadioButton3 = new javax.swing.JRadioButton();
$objectMap.put("$JRadioButton3", $JRadioButton3);
$JRadioButton3.setName("$JRadioButton3");
$JRadioButton3.setText(_("Vegetable"));
{ $JRadioButton3.putClientProperty("$value", "Tomato.jpg"); Object $buttonGroup = $JRadioButton3.getClientProperty("$buttonGroup"); if ($buttonGroup instanceof jaxx.runtime.swing.JAXXButtonGroup) { ((jaxx.runtime.swing.JAXXButtonGroup) $buttonGroup).updateSelectedValue(); } }
}
protected void create$JRadioButton4() {
$JRadioButton4 = new javax.swing.JRadioButton();
$objectMap.put("$JRadioButton4", $JRadioButton4);
$JRadioButton4.setName("$JRadioButton4");
$JRadioButton4.setText(_("Mineral"));
{ $JRadioButton4.putClientProperty("$value", "Amethyst.jpg"); Object $buttonGroup = $JRadioButton4.getClientProperty("$buttonGroup"); if ($buttonGroup instanceof jaxx.runtime.swing.JAXXButtonGroup) { ((jaxx.runtime.swing.JAXXButtonGroup) $buttonGroup).updateSelectedValue(); } }
}
protected void createDemoPanel() {
demoPanel = new jaxx.runtime.swing.HBox();
$objectMap.put("demoPanel", demoPanel);
demoPanel.setName("demoPanel");
((jaxx.runtime.swing.HBox) demoPanel).setHorizontalAlignment(0);
((jaxx.runtime.swing.HBox) demoPanel).setVerticalAlignment(0);
}
protected void createRadioButtons() {
radioButtons = new jaxx.runtime.swing.JAXXButtonGroup();
$objectMap.put("radioButtons", radioButtons);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy