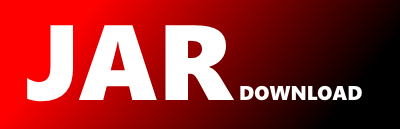
org.nuiton.jaxx.plugin.GenerateHelpIdsMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
/*
* #%L
* JAXX :: Maven plugin
*
* $Id: GenerateHelpIdsMojo.java 2602 2013-03-09 07:21:41Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/jaxx/tags/jaxx-2.5.23/jaxx-maven-plugin/src/main/java/org/nuiton/jaxx/plugin/GenerateHelpIdsMojo.java $
* %%
* Copyright (C) 2008 - 2010 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import com.google.common.base.Charsets;
import com.google.common.io.Closeables;
import com.google.common.io.Files;
import jaxx.compiler.decorators.HelpRootCompiledObjectDecorator;
import org.apache.commons.lang3.StringUtils;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.nuiton.util.SortedProperties;
import java.io.File;
import java.io.IOException;
import java.io.Reader;
import java.io.Writer;
import java.util.HashSet;
import java.util.Locale;
import java.util.Set;
/**
* Generate the help ids files from the previous jaxx generation.
*
* Created: 22 déc. 2009
*
* @author tchemit
* @since 2.0.0
*/
@Mojo(name = "generate-help-ids",
defaultPhase = LifecyclePhase.PROCESS_SOURCES, requiresProject = true)
public class GenerateHelpIdsMojo extends AbstractGenerateHelpMojo {
public static final String INPUT_FILENAME_FULL_FORMAT = "%s-%s_%s.properties";
public static final String INPUT_FILENAME_FORMAT = "%s-%s.properties";
/**
* Flag to merge ids into input directory.
*
* @since 2.5.12
*/
@Parameter(property = "jaxx.mergeIdsToInput",
defaultValue = "false",
required = true)
protected boolean mergeIdsToInput;
/**
* Flag to remove obsolete ids into input files.
*
* Important Note: Be sure to use this after a clean, or
* using with {@code jaxx.force} property to get all ids of any jaxx files
* detected, otherwise you could loose some data. USE WITH CAUTION.
*
* Note: Only used when {@code mergeIdsToInput} parameter
* is on.
*
* @since 2.5.12
*/
@Parameter(property = "jaxx.strictMode",
defaultValue = "false",
required = true)
protected boolean strictMode;
/**
* Directory where to merge (create) input files.
*
* Note: Only used when {@code mergeIdsToInput} parameter
* is on.
*
* @since 2.5.12
*/
@Parameter(property = "jaxx.inputHelpDirectory",
defaultValue = "src/main/help",
required = true)
protected File inputHelpDirectory;
/**
* Prefix of input files.
*
* Note: {@code -locale.properties} will be added to each
* generated file (example: {@code helpMapping-fr_FR.properties}).
*
* Note: Only used when {@code mergeIdsToInput} parameter
* is on.
*
* @since 2.5.12
*/
@Parameter(property = "jaxx.inputHelpFilenamePrefix",
defaultValue = "helpMapping",
required = true)
protected String inputHelpFilenamePrefix;
/** help ids to react. */
protected Set helpIds;
@Override
public void init() throws Exception {
if (!generateHelp) {
return;
}
super.init();
// check there is some bundle
if (getHelpIdsFilename() == null) {
throw new MojoFailureException(
"you must set the 'helpIdStore' property.");
}
if (getMergeHelpIdsFilename() == null) {
throw new MojoFailureException(
"you must set the 'helpIdStoreAll' property.");
}
helpIds = HelpRootCompiledObjectDecorator.getHelpIds();
// always clean helpIdsStore before all
cleanHelpIdsStore();
if (mergeIdsToInput) {
createDirectoryIfNecessary(inputHelpDirectory);
}
}
@Override
protected boolean checkSkip() {
boolean b = super.checkSkip();
if (b) {
if (strictMode) {
// always launch goal
getLog().info("Strict mode is on, will use all detected ids.");
} else if (helpIds.isEmpty()) {
// no ids detected in this compilation round
getLog().info("No help ids to treate, will skip goal.");
b = false;
}
}
return b;
}
@Override
protected void preDoAction() throws IOException {
// store current jaxx session detected help ids
File idsStore = getHelpIdsStoreFile();
getLog().info("Store detected help ids to " + idsStore);
storeHelpIds(idsStore, helpIds);
// store merged help ids (to make possible a force on help generation)
File idsStoreAll = getMergeHelpIdsStoreFile();
getLog().info("Merge help ids to " + idsStoreAll);
if (idsStoreAll.exists()) {
Set allIds = loadHelpIds(idsStoreAll);
helpIds.addAll(allIds);
}
storeHelpIds(idsStoreAll, helpIds);
}
@Override
protected void doActionForLocale(Locale locale,
boolean isDefaultLocale,
File source,
String localePath) throws Exception {
// nothing to do specific to locale
if (mergeIdsToInput) {
String filename;
if (StringUtils.isNotEmpty(locale.getCountry())) {
filename = String.format(
INPUT_FILENAME_FULL_FORMAT,
inputHelpFilenamePrefix,
locale.getLanguage(),
locale.getCountry());
} else {
filename = String.format(
INPUT_FILENAME_FORMAT,
inputHelpFilenamePrefix,
locale.getLanguage());
}
File inputFile = new File(inputHelpDirectory, filename);
getLog().info("Use input file: " + inputFile);
SortedProperties p = new SortedProperties();
if (inputFile.exists()) {
Reader reader = Files.newReader(inputFile, Charsets.UTF_8);
try {
p.load(reader);
reader.close();
} finally {
Closeables.closeQuietly(reader);
}
}
Set existingId = p.stringPropertyNames();
Set newIds = new HashSet();
SortedProperties resultProperties = p;
// get all detected ids
for (String helpId : helpIds) {
newIds.add(removeQuote(helpId));
}
if (strictMode) {
// remove all obsolete ids
for (String s : existingId) {
if (!newIds.contains(s)) {
resultProperties.remove(s);
}
}
// update existing ids
existingId.retainAll(newIds);
} else {
// remove all existing ids (no need to treat them again)
newIds.removeAll(existingId);
}
if (newIds.isEmpty()) {
getLog().info("No keys to add.");
} else {
if (strictMode) {
getLog().info("Regenerate files with " + newIds.size() + " keys.");
} else {
getLog().info("Add " + newIds.size() + " keys.");
}
for (String helpId : newIds) {
if (!resultProperties.containsKey(helpId)) {
resultProperties.put(helpId, "");
}
}
Writer writer = Files.newWriter(inputFile, Charsets.UTF_8);
try {
resultProperties.store(writer, "Generated by " + getClass().getName());
writer.close();
} finally {
Closeables.closeQuietly(writer);
}
}
}
}
@Override
protected void postDoAction() {
helpIds.clear();
}
@Override
public File getTargetDirectory() {
return getOutputHelpIds();
}
@Override
public void setTargetDirectory(File targetDirectory) {
setOutputHelpIds(targetDirectory);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy