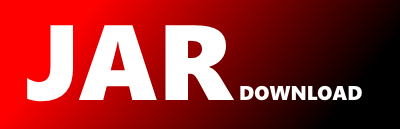
org.nuiton.jaxx.plugin.AbstractGenerateHelpMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
The newest version!
/*
* #%L
* JAXX :: Maven plugin
* %%
* Copyright (C) 2008 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import org.apache.commons.lang3.StringUtils;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Parameter;
import org.nuiton.i18n.I18nUtil;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Locale;
import java.util.Set;
/**
* Abstract Mojo to generate help stuff.
*
* @author Tony Chemit - [email protected]
* @since 2.0.0
*/
public abstract class AbstractGenerateHelpMojo extends AbstractJaxxMojo {
/**
* The locales to generate for help, separeted by comma.
*
* The first locale given is the default locale.
*
* @since 2.0.0
*/
@Parameter(property = "jaxx.locales", required = true)
protected String locales;
/**
* Where to generate helpIds files.
*
* @since 1.3
*/
@Parameter(property = "jaxx.outputHelpIds", defaultValue = "target/generated-sources/jaxx", required = true)
private File outputHelpIds;
/**
* The store of helpIds generated by the goal {@link GenerateMojo} and then
* used by the goal {@link GenerateHelpMojo}.
*
* @since 1.3
*/
@Parameter(property = "jaxx.helpIdsFilename", defaultValue = "helpIds.txt", required = true)
private String helpIdsFilename;
/**
* The store of cumulate helpIds generated by the goal {@link GenerateMojo}
* and then used by the goal {@link GenerateHelpMojo}.
*
* @since 1.3
*/
@Parameter(property = "jaxx.mergeHelpIdsFilename", defaultValue = "helpIds-all.txt", required = true)
private String mergeHelpIdsFilename;
/**
* Flag to generate the search index.
*
* @since 1.3
*/
@Parameter(property = "jaxx.generateSearch", defaultValue = "true", required = true)
protected boolean generateSearch;
/** Default locale (the first locale in {@link #localesToTreate}. */
private Locale defaultLocale;
/** Locales to treate */
private Locale[] localesToTreate;
/**
* Do the action for the given locale.
*
* @param locale the locale to treate
* @param isDefaultLocale {@code true} if given locale is de the default
* locale
* @param source where are stored help files for the given locale
* @param localePath the locale path to use (is {@code default} if
* given locale is default).
* @throws Exception if any pb
*/
protected abstract void doActionForLocale(
Locale locale,
boolean isDefaultLocale,
File source,
String localePath) throws Exception;
/** Call back after doing all stuff for all locales declared */
protected abstract void postDoAction();
/** Call back before doing all stuff for all locales declared */
protected abstract void preDoAction() throws IOException;
@Override
protected void init() throws Exception {
if (StringUtils.isEmpty(locales)) {
throw new MojoFailureException(
"You must set the 'locales' property properly (was " +
locales + ").");
}
// check there is a outHelp
if (getTargetDirectory() == null) {
throw new MojoFailureException(
"You must set the 'outputHelpXXX' property.");
}
List tmp = new ArrayList<>();
for (String loc : locales.split(",")) {
Locale l = I18nUtil.newLocale(loc);
tmp.add(l);
}
if (tmp.isEmpty()) {
throw new MojoFailureException(
"No locale to react, you must set the 'locales' property.");
}
localesToTreate = tmp.toArray(new Locale[tmp.size()]);
defaultLocale = localesToTreate[0];
createDirectoryIfNecessary(getTargetDirectory());
}
@Override
protected boolean checkSkip() {
if (!generateHelp) {
getLog().info("generateHelp flag is off, will skip goal.");
return false;
}
return true;
}
@Override
protected void doAction() throws Exception {
preDoAction();
for (Locale locale : localesToTreate) {
boolean isDefaultLocale = defaultLocale.equals(locale);
String language = locale.getLanguage();
String localePath = isDefaultLocale ? "default" : language;
File source = new File(getTargetDirectory(), localePath);
createDirectoryIfNecessary(source);
doActionForLocale(locale, isDefaultLocale, source, localePath);
}
postDoAction();
}
public File getOutputHelpIds() {
return outputHelpIds;
}
public void setOutputHelpIds(File outputHelpIds) {
this.outputHelpIds = outputHelpIds;
}
public File getHelpIdsStoreFile() {
return outputHelpIds == null ? null :
new File(outputHelpIds, helpIdsFilename);
}
public File getMergeHelpIdsStoreFile() {
return outputHelpIds == null ? null :
new File(outputHelpIds, mergeHelpIdsFilename);
}
public String getHelpIdsFilename() {
return helpIdsFilename;
}
public void setHelpIdsFilename(String helpIdsFilename) {
this.helpIdsFilename = helpIdsFilename;
}
public String getMergeHelpIdsFilename() {
return mergeHelpIdsFilename;
}
public void setMergeHelpIdsFilename(String mergeHelpIdsFilename) {
this.mergeHelpIdsFilename = mergeHelpIdsFilename;
}
protected void cleanHelpIdsStore() throws IOException {
File idsStore = getHelpIdsStoreFile();
if (idsStore.exists()) {
if (getLog().isDebugEnabled()) {
getLog().debug("delete id store " + idsStore);
}
boolean b = idsStore.delete();
if (!b) {
throw new IOException("could not delete file " + idsStore);
}
}
}
protected Set loadHelpIds(File file) throws IOException {
BufferedReader reader;
Set result = new HashSet<>();
reader = new BufferedReader(new InputStreamReader(
new FileInputStream(file), getEncoding()));
try {
String id;
while ((id = reader.readLine()) != null) {
id = id.trim();
if (!id.startsWith("#")) {
result.add(id);
}
}
if (isVerbose()) {
getLog().info("load " + result.size() +
" help ids from file " + file);
}
return result;
} finally {
reader.close();
}
}
protected void storeHelpIds(File file, Set ids) throws IOException {
createDirectoryIfNecessary(file.getParentFile());
StringBuilder buffer = new StringBuilder();
for (String helpId : ids) {
buffer.append(removeQuote(helpId)).append('\n');
}
writeFile(file, buffer.toString(), getEncoding());
if (isVerbose()) {
getLog().info("stored " + ids.size() + " help ids in " + file);
}
}
protected String removeQuote(String txt) {
if (txt.startsWith("\"")) {
txt = txt.substring(1);
}
if (txt.endsWith("\"")) {
txt = txt.substring(0, txt.length() - 1);
}
return txt;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy