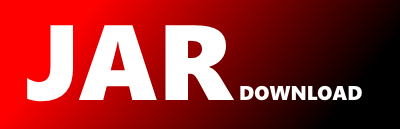
org.nuiton.jaxx.plugin.AbstractJaxxMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
The newest version!
/*
* #%L
* JAXX :: Maven plugin
* %%
* Copyright (C) 2008 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.nuiton.io.MirroredFileUpdater;
import org.nuiton.plugin.AbstractPlugin;
import org.nuiton.plugin.PluginWithEncoding;
import org.nuiton.util.FileUtil;
import java.io.File;
/**
* Abstract Jaxx Mojo.
*
* @author Tony Chemit - [email protected]
* @since 1.3
*/
public abstract class AbstractJaxxMojo extends AbstractPlugin implements PluginWithEncoding {
public abstract File getTargetDirectory();
public abstract void setTargetDirectory(File targetDirectory);
/** Current maven project. */
@Parameter(defaultValue = "${project}", readonly = true)
private MavenProject project;
/**
* Encoding used for file generation.
*
* Note: If nothing is filled here, we will use the system
* property {@code file.encoding}.
*
* @since 2.0.0
*/
@Parameter(property = "jaxx.encoding", defaultValue = "${project.build.sourceEncoding}")
private String encoding;
/**
* Verbose flag.
*
* @since 1.3
*/
@Parameter(property = "jaxx.verbose", defaultValue = "false")
private boolean verbose;
/**
* To force generation of java source for any jaxx files with no timestamp checking.
*
* By default, never force generation.
*/
@Parameter(property = "jaxx.force", defaultValue = "false")
private boolean force;
/**
* Flag to activate help id detection while parsing jaxx files.
*
* By default, not active.
*
* @since 1.3
*/
@Parameter(property = "jaxx.generateHelp", defaultValue = "false")
protected boolean generateHelp;
@Override
protected boolean checkPackaging() {
// not accept pom modules
return hasClassPath();
}
@Override
public MavenProject getProject() {
return project;
}
@Override
public boolean isVerbose() {
return verbose;
}
@Override
public void setProject(MavenProject project) {
this.project = project;
}
@Override
public void setVerbose(boolean verbose) {
this.verbose = verbose;
}
@Override
public String getEncoding() {
return encoding;
}
@Override
public void setEncoding(String encoding) {
this.encoding = encoding;
}
public boolean isForce() {
return force;
}
public void setForce(boolean force) {
this.force = force;
}
class GetLastModifiedFileAction implements FileUtil.FileAction {
protected File lastFile;
public GetLastModifiedFileAction(File lastFile) {
this.lastFile = lastFile;
}
@Override
public boolean doAction(File f) {
if (f.lastModified() > lastFile.lastModified()) {
lastFile = f;
}
return true;
}
public File getLastFile() {
return lastFile;
}
}
protected Long getLastModified(File dir) {
if (!dir.exists()) {
return null;
}
GetLastModifiedFileAction fileAction =
new GetLastModifiedFileAction(dir);
FileUtil.walkAfter(dir, fileAction);
return fileAction.getLastFile().lastModified();
}
/**
* To test if a jaxx source (or his css ) file is newser than his generated
* java source file.
*
* @author Tony Chemit - [email protected]
* @since 2.0.2
*/
public static class JaxxFileUpdater extends MirroredFileUpdater {
protected JaxxFileUpdater(String cssExtension, File sourceDirectory, File destinationDirectory) {
super(".jaxx|*." + cssExtension, ".java", sourceDirectory, destinationDirectory);
}
@Override
public File getMirrorFile(File f) {
String file = f.getAbsolutePath().substring(prefixSourceDirecotory);
String extension = FileUtil.extension(f);
String mirrorRelativePath = file.substring(0, file.length() - extension.length()) + "java";
return new File(destinationDirectory + File.separator + mirrorRelativePath);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy