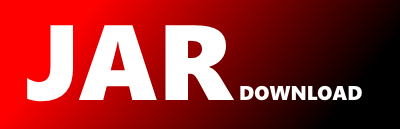
org.nuiton.jaxx.plugin.GenerateHelpFilesMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
The newest version!
/*
* #%L
* JAXX :: Maven plugin
* %%
* Copyright (C) 2008 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import org.apache.maven.plugins.annotations.Execute;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.plugins.annotations.ResolutionScope;
import org.nuiton.plugin.VelocityTemplateGenerator;
import org.nuiton.util.SortedProperties;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.util.Enumeration;
import java.util.Locale;
import java.util.Properties;
import java.util.Set;
/**
* Mojo to generate javax help files for your project.
*
* @author Tony Chemit - [email protected]
* @since 2.0.0
*/
@Mojo(name = "generate-help-files",
defaultPhase = LifecyclePhase.PROCESS_SOURCES,
requiresProject = true,
requiresDependencyResolution = ResolutionScope.COMPILE)
@Execute(goal = "generate-help-ids")
public class GenerateHelpFilesMojo extends AbstractGenerateHelpMojo {
protected static final String AUTOREMOVE_LINE =
"REMOVE THS LINE TO DISABLE AUTO-REGENERATE THE FILE";
/**
* The directory where to create or update help files.
*
* @since 2.0.0
*/
@Parameter(property = "jaxx.outputHelp", defaultValue = "${project.basedir}/src/main/help", required = true)
protected File outputHelp;
/**
* The name of the helpset to generate.
*
* @since 1.3
*/
@Parameter(property = "jaxx.helpsetName", defaultValue = "${project.artifactId}", required = true)
protected String helpsetName;
/**
* The template used to generate helpset file.
*
* Must be an existing file or a ressource in class-path
*
* @since 1.3
*/
@Parameter(property = "jaxx.helpsetTemplate", defaultValue = "/defaultHelpSet.hs.vm", required = true)
protected File helpsetTemplate;
/**
* The template used to generate helpset map file.
*
* Must be an existing file or a ressource in class-path
*
* @since 1.3
*/
@Parameter(property = "jaxx.mapTemplate", defaultValue = "/defaultMap.jhm.vm", required = true)
protected File mapTemplate;
/**
* The template used to generate helpset index file.
*
* Must be an existing file or a ressource in class-path
*
* @since 1.3
*/
@Parameter(property = "jaxx.indexTemplate", defaultValue = "/defaultIndex.xml.vm", required = true)
protected File indexTemplate;
/**
* The template used to generate helpset toc file.
*
* Must be an existing file or a ressource in class-path
*
* @since 1.3
*/
@Parameter(property = "jaxx.tocTemplate", defaultValue = "/defaultToc.xml.vm", required = true)
protected File tocTemplate;
/**
* The template used to generate helpset content file.
*
* Must be an existing file or a ressource in class-path
*
* @since 1.3
*/
@Parameter(property = "jaxx.contentTemplate", defaultValue = "/defaultContent.html.vm", required = true)
protected File contentTemplate;
protected String mapFileName;
protected String indexFileName;
protected String tocFileName;
protected int touchedFiles;
protected File idsFile;
/** The help ids discovered. */
protected Properties helpIds;
@Override
public File getTargetDirectory() {
return outputHelp;
}
@Override
public void setTargetDirectory(File targetDirectory) {
outputHelp = targetDirectory;
}
@Override
public void init() throws Exception {
if (!generateHelp) {
return;
}
// check ressources
checkResource(helpsetTemplate);
checkResource(mapTemplate);
checkResource(indexTemplate);
checkResource(tocTemplate);
checkResource(contentTemplate);
mapFileName = helpsetName + "Map.jhm";
indexFileName = helpsetName + "Index.xml";
tocFileName = helpsetName + "TOC.xml";
touchedFiles = 0;
super.init();
// load ids from idsStore file
if (isForce()) {
idsFile = getMergeHelpIdsStoreFile();
} else {
idsFile = getHelpIdsStoreFile();
}
if (!idsFile.exists()) {
return;
}
helpIds = new SortedProperties();
// loading all ids
Set ids = loadHelpIds(idsFile);
for (String id : ids) {
String path = id.replaceAll("\\.", File.separator) + ".html";
helpIds.put(id, path);
}
}
@Override
protected boolean checkSkip() {
boolean b = super.checkSkip();
if (b) {
if (!idsFile.exists()) {
// pas de fichier a traiter
if (isForce()) {
getLog().info("Force flag is on, but no helpIdStore-all" +
" to react at " + idsFile);
} else {
getLog().info("no helpIdStore to react at " + idsFile);
}
return false;
}
if (helpIds == null || helpIds.isEmpty()) {
getLog().info("No help ids to treate.");
return false;
}
}
return b;
}
@Override
protected void preDoAction() throws IOException {
}
@Override
protected void postDoAction() {
getLog().info(touchedFiles + " file(s) treated.");
}
@Override
protected void doActionForLocale(Locale locale,
boolean isDefaultLocale,
File localizedTarget,
String localePath) throws Exception {
String language = locale.getLanguage();
getLog().info("Generate help for language " + language);
if (isVerbose()) {
getLog().info(" Localized target : " + localizedTarget);
}
Properties env = new Properties();
env.put("helpSetName", helpsetName);
env.put("locale", language);
env.put("localePath", localePath);
env.put("separator", " ");
env.put("autoremoveLine", AUTOREMOVE_LINE);
String localeSuffix = isDefaultLocale ? "" : "_" + language;
String helpsetFileName = helpsetName + localeSuffix + ".hs";
env.put("helpSetFileName", helpsetFileName);
env.put("mapFileName", mapFileName);
env.put("indexFileName", indexFileName);
env.put("tocFileName", tocFileName);
env.put("generateSearch", generateSearch);
// ---------------------------------------------------------------
// --- main helpset file -----------------------------------------
// ---------------------------------------------------------------
File file = new File(getTargetDirectory(), helpsetFileName);
boolean doCreate = generateHelpsetFile(file, env);
if (doCreate) {
touchedFiles++;
}
// ---------------------------------------------------------------
// --- helpset map file ------------------------------------------
// ---------------------------------------------------------------
file = new File(localizedTarget, mapFileName);
generateMapFile(file, env);
touchedFiles++;
// ---------------------------------------------------------------
// --- helpset index file ----------------------------------------
// ---------------------------------------------------------------
file = new File(localizedTarget, indexFileName);
generateIndexFile(file, env);
touchedFiles++;
// ---------------------------------------------------------------
// --- helpset toc file ------------------------------------------
// ---------------------------------------------------------------
file = new File(localizedTarget, tocFileName);
generateTocFile(file, env);
touchedFiles++;
// ---------------------------------------------------------------
// --- helpset content files -------------------------------------
// ---------------------------------------------------------------
touchedFiles += generateContentFiles(localizedTarget, env, localePath);
}
protected int generateContentFiles(File localizedTarget,
Properties env,
String localePath) throws Exception {
int touchedFiles = 0;
VelocityTemplateGenerator gen = prepareGenerator(contentTemplate);
Enumeration> keys = helpIds.keys();
while (keys.hasMoreElements()) {
String key = (String) keys.nextElement();
String url = (String) helpIds.get(key);
url = helpsetName + File.separator + url;
File f = new File(localizedTarget, url);
boolean exist = f.exists();
if (exist) {
// check if there is a autoremoveLine in content
String content = new String(Files.readAllBytes(f.toPath()), StandardCharsets.UTF_8);
if (!content.contains(AUTOREMOVE_LINE)) {
// no regenerate marker detected, so skip this file
if (isVerbose()) {
getLog().debug("skip existing file " + f);
}
continue;
}
}
createDirectoryIfNecessary(f.getParentFile());
if (isVerbose()) {
if (exist) {
getLog().info("regenerate content file " + f);
} else {
getLog().info("generate content file " + f);
}
}
env.put("helpId", key);
env.put("helpIdUrl", localePath + "/" + url);
gen.generate(env, f);
touchedFiles++;
}
return touchedFiles;
}
protected boolean generateHelpsetFile(File file,
Properties env) throws Exception {
if (file.exists()) {
// check the autoremove line presence
String content = new String(Files.readAllBytes(file.toPath()), StandardCharsets.UTF_8);
if (!content.contains(AUTOREMOVE_LINE)) {
// no regenerate marker detected, so skip this file
if (isVerbose()) {
getLog().info("skip existing helpset main file " + file);
}
return false;
}
}
if (isVerbose()) {
if (file.exists()) {
getLog().info("regenerate helpset main file " + file);
} else {
getLog().info("generate helpset main file " + file);
}
}
doGen(helpsetTemplate, file, env);
return true;
}
protected Properties generateMapFile(File file,
Properties env) throws Exception {
boolean create;
Properties mergedHelpIds;
if (file.exists()) {
// get back the exisiting data and merge it with incoming ones
if (isVerbose()) {
getLog().info("loading existing helpset map file " + file);
}
mergedHelpIds = XmlHelper.getExistingHelpIds(file, isVerbose(),
getLog());
create = false;
} else {
mergedHelpIds = new SortedProperties();
create = true;
}
// inject new helpIds
for (Object k : helpIds.keySet()) {
mergedHelpIds.put(k, helpsetName + "/" + helpIds.get(k));
}
if (!mergedHelpIds.contains("top")) {
// on ajoute une entree vers le root du helpset
String topUrl = helpsetName + ".html";
helpIds.put("top", topUrl);
mergedHelpIds.put("top", helpsetName + "/" + topUrl);
if (isVerbose()) {
getLog().debug("add top entry with url " + topUrl);
}
}
if (isVerbose()) {
if (create) {
getLog().info("generate helpset map file " + file);
} else {
getLog().info("udpate helpset map file " + file);
}
}
env.put("helpIds", mergedHelpIds);
doGen(mapTemplate, file, env);
env.remove("helpIds");
return mergedHelpIds;
}
protected NodeItem generateIndexFile(File file,
Properties env) throws Exception {
NodeItem rootItem = null;
boolean create;
if (file.exists()) {
create = false;
rootItem = XmlHelper.getExistingItems("indexitem", file);
} else {
create = true;
}
if (rootItem == null) {
rootItem = new NodeItem("top", helpsetName);
}
// inject new index entries
for (Object k : helpIds.keySet()) {
NodeItem toc = rootItem.findChild(k + "");
if (isVerbose()) {
getLog().debug("index " + k + " : " + toc);
}
}
if (isVerbose()) {
if (create) {
getLog().info("generate helpset index file " + file);
} else {
getLog().info("udpate helpset index file " + file);
}
}
env.put("rootItem", rootItem);
doGen(indexTemplate, file, env);
env.remove("rootItem");
return rootItem;
}
protected NodeItem generateTocFile(File file,
Properties env) throws Exception {
NodeItem rootItem = null;
boolean create;
if (file.exists()) {
create = false;
rootItem = XmlHelper.getExistingItems("tocitem", file);
} else {
create = true;
}
if (rootItem == null) {
rootItem = new NodeItem("top", helpsetName);
}
// inject new toc entries
for (Object k : helpIds.keySet()) {
NodeItem toc = rootItem.findChild(k + "");
if (isVerbose()) {
getLog().debug("toc " + k + " : " + toc);
}
}
if (isVerbose()) {
if (create) {
getLog().info("generate helpset toc file " + file);
} else {
getLog().info("udpate helpset toc file " + file);
}
}
env.put("rootItem", rootItem);
doGen(tocTemplate, file, env);
env.remove("rootItem");
return rootItem;
}
protected void doGen(File template, File f,
Properties env) throws Exception {
VelocityTemplateGenerator gen = prepareGenerator(template);
gen.generate(env, f);
}
protected VelocityTemplateGenerator prepareGenerator(
File template) throws Exception {
URL templateURL = getTemplate(template);
if (isVerbose()) {
getLog().info("using template " + templateURL);
}
return new VelocityTemplateGenerator(getProject(), templateURL);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy