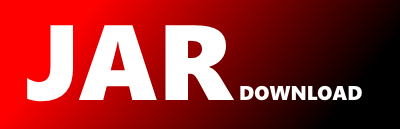
org.nuiton.jaxx.plugin.GenerateHelpSearchMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
The newest version!
/*
* #%L
* JAXX :: Maven plugin
* %%
* Copyright (C) 2008 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import com.sun.java.help.search.Indexer;
import org.apache.maven.plugins.annotations.Execute;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.plugins.annotations.ResolutionScope;
import org.codehaus.plexus.util.FileUtils;
import org.nuiton.plugin.PluginHelper;
import java.io.File;
import java.io.IOException;
import java.io.PrintStream;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import java.util.Locale;
/**
* Generate the javax search index help for your project.
*
* The current files should be generated always in a generated directory and not in
* your src directories (this is mainly binary files not to be stored in scm system)...
*
* @author Tony Chemit - [email protected]
* @since 2.0.0
*/
@Mojo(name = "generate-help-search",
defaultPhase = LifecyclePhase.PROCESS_SOURCES,
requiresProject = true,
requiresDependencyResolution = ResolutionScope.COMPILE)
@Execute(goal = "generate-help-files")
public class GenerateHelpSearchMojo extends AbstractGenerateHelpMojo {
/**
* The directory where to create or update help search index files.
*
* @since 2.0.0
*/
@Parameter(property = "jaxx.outputHelpSearch", defaultValue = "${project.basedir}/target/generated-sources/help", required = true)
protected File outputHelpSearch;
/**
* The directory where to pick content files to generate the index.
*
* @since 2.0.0
*/
@Parameter(property = "jaxx.inputHelp", defaultValue = "${project.basedir}/src/main/help", required = true)
protected File inputHelp;
protected String timestamp;
@Override
public void init() throws Exception {
if (!generateHelp || !generateSearch) {
return;
}
timestamp = "-" + System.currentTimeMillis();
super.init();
}
@Override
public File getTargetDirectory() {
return outputHelpSearch;
}
@Override
public void setTargetDirectory(File targetDirectory) {
outputHelpSearch = targetDirectory;
}
@Override
protected boolean checkSkip() {
boolean b = super.checkSkip();
if (b) {
if (!generateSearch) {
getLog().info("generateSearch flag is off, will skip goal.");
return false;
}
}
return b;
}
@Override
protected void doActionForLocale(Locale locale,
boolean isDefaultLocale,
File localizedTarget,
String localePath) throws Exception {
String language = locale.getLanguage();
File source = new File(inputHelp, localePath);
File target = new File(localizedTarget, "JavaHelpSearch");
// File target = new File(getTargetDirectory(), localePath + File.separator + "JavaHelpSearch");
// detect if need to generate
boolean generate = false;
if (isForce()) {
// always generate if force flag is on
generate = true;
} else if (!target.exists()) {
// target does not exist, must generate it
generate = true;
} else {
// see if there is something new in source
Long sourceLast = getLastModified(source);
Long targetLast = getLastModified(target);
if (isVerbose()) {
getLog().info("lastModified of source : " + sourceLast);
getLog().info("lastModified of target : " + targetLast);
}
if (targetLast == null || sourceLast == null ||
targetLast < sourceLast) {
// something is newer in source than in target
generate = true;
}
}
if (!generate) {
getLog().info("Nothing to generate for language " + language +
" - all files are up to date.");
return;
}
getLog().info("Generate help search index for language " + language);
if (isVerbose()) {
getLog().info(" from " + source);
getLog().info(" to " + target);
}
createDirectoryIfNecessary(source);
// createDirectoryIfNecessary(localizedTarget);
createDirectoryIfNecessary(target);
// ---------------------------------------------------------------
// --- generate search index -------------------------------------
// ---------------------------------------------------------------
generateSearchIndex(source, target, locale);
}
@Override
protected void preDoAction() throws IOException {
}
@Override
protected void postDoAction() {
// add resources to the project
addResourceDir(getTargetDirectory(), "**/*");
}
protected void generateSearchIndex(File source,
File target,
Locale locale) throws Exception {
long t0 = System.nanoTime();
Method m = Indexer.class.getDeclaredMethod("main", String[].class);
// remove old index
FileUtils.deleteDirectory(target);
//copy resources to a tmp dir (without any VCS infos)
File tmpDir = getFileFromBasedir("target", "jaxx-tmp", "indexer-" + locale + timestamp);
if (isVerbose()) {
getLog().info("copy files to " + tmpDir + " for indexing them.");
}
FileUtils.copyDirectoryStructure(source, tmpDir);
// FileUtils.copyDirectory(source, tmpDir, "**/*", StringUtils.join(DirectoryScanner.DEFAULTEXCLUDES, ",")+",**/*.jpg");
// prepare arguments of Indexer.main calling
List params = new ArrayList<>();
params.add("-verbose");
params.add("-db");
params.add(target.getAbsolutePath());
params.add("-logfile");
File logFile = getFileFromBasedir("target", "generated-sources", "jaxx", "indexer-" + locale + ".log");
params.add(logFile.getAbsolutePath());
params.add(tmpDir.getAbsolutePath());
PrintStream out = System.out;
PrintStream err = System.err;
// invoke Indexer.main
try {
m.invoke(null, (Object) params.toArray(new String[params.size()]));
} finally {
System.setOut(out);
System.setErr(err);
}
if (isVerbose()) {
getLog().info(
"Search Index generated for " + locale + " in " +
PluginHelper.convertTime(System.nanoTime() - t0));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy