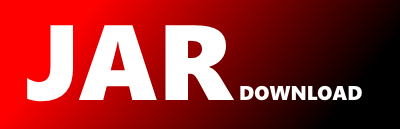
org.nuiton.jaxx.plugin.NodeItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
The newest version!
/*
* #%L
* JAXX :: Maven plugin
* %%
* Copyright (C) 2008 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import static org.nuiton.i18n.I18n.t;
public class NodeItem {
String absoluteTarget;
private String target;
private String text;
private List childs;
public NodeItem(String target, String text) {
this.target = target;
absoluteTarget = target;
this.text = text;
}
public String getTarget() {
return target;
}
public String getAbsoluteTarget() {
return absoluteTarget;
}
public String getText() {
return text;
}
public List getChilds() {
return childs;
}
public void setText(String text) {
this.text = text;
}
public NodeItem findChild(String path) {
NodeItem result = null;
String[] paths = path.split("\\.");
for (String p : paths) {
if (result == null) {
// first node
if (target.equals(p)) {
result = this;
continue;
}
result = getChild(p);
if (result == null) {
result = new NodeItem(p, null);
addChild(result);
adjutsAbsoluteTarget(result);
}
continue;
}
NodeItem child = result.getChild(p);
if (child == null) {
child = new NodeItem(p, null);
result.addChild(child);
result.adjutsAbsoluteTarget(child);
result = child;
} else {
result = child;
}
}
return result;
}
public NodeItem getChild(int index) {
return childs.get(index);
}
public NodeItem getChild(String target) {
if (isLeaf()) {
return null;
}
for (NodeItem i : childs) {
if (i.target.equals(target)) {
return i;
}
}
return null;
}
public void addChild(NodeItem child) {
if (childs == null) {
childs = new ArrayList<>();
}
childs.add(child);
}
public void adjutsAbsoluteTarget(NodeItem child) {
if (!"top".equals(target)) {
// on ne prefixe pas les fils direct du root
child.absoluteTarget = absoluteTarget + "." + child.target;
}
}
public void adjustTarget() {
int index = target.lastIndexOf(".");
if (index > -1) {
target = target.substring(index + 1);
}
}
public boolean isLeaf() {
return childs == null || childs.isEmpty();
}
public void applyI18n(String prefix, String suffix) {
String key = prefix + getAbsoluteTarget() + suffix;
text = t(key);
if (!isLeaf()) {
for (NodeItem i : getChilds()) {
i.applyI18n(prefix, suffix);
}
}
}
public void extractI18n(Set keys, String prefix, String suffix) {
String key = prefix + getAbsoluteTarget() + suffix;
keys.add(key);
if (!isLeaf()) {
for (NodeItem i : getChilds()) {
i.extractI18n(keys, prefix, suffix);
}
}
}
@Override
public String toString() {
return super.toString() + "";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy