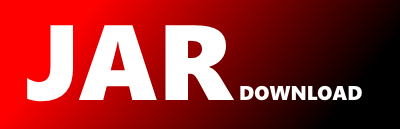
org.nuiton.jaxx.plugin.XmlHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-maven-plugin Show documentation
Show all versions of jaxx-maven-plugin Show documentation
Maven 2 plugin to generate java sources from JAXX files.
The newest version!
/*
* #%L
* JAXX :: Maven plugin
* %%
* Copyright (C) 2008 - 2017 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.jaxx.plugin;
import org.apache.maven.plugin.logging.Log;
import org.nuiton.util.SortedProperties;
import org.xml.sax.Attributes;
import org.xml.sax.ContentHandler;
import org.xml.sax.InputSource;
import org.xml.sax.Locator;
import org.xml.sax.SAXException;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.XMLReaderFactory;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import java.util.Stack;
/**
* To load {@link NodeItem} from xml.
*
* @author Tony Chemit - [email protected]
* @since 2.0.0
*/
public class XmlHelper {
public static Properties getExistingHelpIds(File file,
final boolean verbose,
final Log log)
throws SAXException, IOException {
final Properties result = new SortedProperties();
XMLReader parser = XMLReaderFactory.createXMLReader();
parser.setContentHandler(new ContentHandlerAdapter() {
String target;
String url;
@Override
public void startElement(String uri,
String localName,
String qName,
Attributes atts) throws SAXException {
if ("mapID".equals(localName)) {
target = atts.getValue("target");
url = atts.getValue("url");
if (verbose) {
log.debug("detect map entry : " + target + " : " + url);
}
result.put(target, url);
}
}
});
try (InputStream s = new FileInputStream(file)) {
parser.parse(new InputSource(s));
}
return result;
}
public static NodeItem getExistingItems(String tagName,
File file)
throws SAXException, IOException {
XMLReader parser = XMLReaderFactory.createXMLReader();
NodeItemHandler handler = new NodeItemHandler(tagName);
parser.setContentHandler(handler);
NodeItem rootItem = null;
try (InputStream s = new FileInputStream(file)) {
parser.parse(new InputSource(s));
rootItem = handler.rootItem;
}
return rootItem;
}
static class NodeItemHandler extends ContentHandlerAdapter {
NodeItem rootItem;
NodeItem currentItem;
final Stack stack;
final String tagName;
public NodeItemHandler(String tagName) {
this.tagName = tagName;
stack = new Stack<>();
}
@Override
public void startDocument() throws SAXException {
rootItem = new NodeItem("top", null);
}
@Override
public void startElement(String uri,
String localName,
String qName,
Attributes atts) throws SAXException {
if (tagName.equals(localName)) {
String target = atts.getValue("target");
String text = atts.getValue("text");
// debut d'un item
if (currentItem == null) {
// premier item
if (rootItem.getTarget().equals(target)) {
// le premier item est bien top
rootItem.setText(text);
currentItem = rootItem;
} else {
// le premier noeud n'est pas top
// en l'encapsule
stack.push(rootItem);
currentItem = new NodeItem(target, text);
rootItem.addChild(currentItem);
}
} else {
NodeItem newItem = new NodeItem(target, text);
currentItem.addChild(newItem);
currentItem = newItem;
}
currentItem.adjustTarget();
stack.push(currentItem);
}
}
@Override
public void endElement(String uri,
String localName,
String qName) throws SAXException {
if (tagName.equals(localName)) {
// fin d'un item
stack.pop();
if (!stack.isEmpty()) {
currentItem = stack.peek();
}
}
}
}
static class ContentHandlerAdapter implements ContentHandler {
@Override
public void setDocumentLocator(Locator locator) {
}
@Override
public void startDocument() throws SAXException {
}
@Override
public void endDocument() throws SAXException {
}
@Override
public void startPrefixMapping(String prefix,
String uri) throws SAXException {
}
@Override
public void endPrefixMapping(String prefix) throws SAXException {
}
@Override
public void startElement(String uri,
String localName,
String qName,
Attributes atts) throws SAXException {
}
@Override
public void endElement(String uri,
String localName,
String qName) throws SAXException {
}
@Override
public void characters(char[] ch,
int start,
int length) throws SAXException {
}
@Override
public void ignorableWhitespace(char[] ch,
int start,
int length) throws SAXException {
}
@Override
public void processingInstruction(String target,
String data) throws SAXException {
}
@Override
public void skippedEntity(String name) throws SAXException {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy