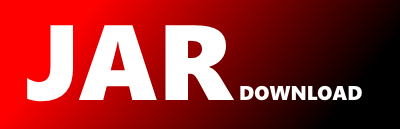
jaxx.runtime.context.JAXXContextEntryDef Maven / Gradle / Ivy
/*
* *##%
* JAXX Runtime
* Copyright (C) 2008 - 2009 CodeLutin
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* ##%*
*/
package jaxx.runtime.context;
import java.util.List;
import java.util.Map;
import jaxx.runtime.JAXXContext;
/**
* To qualify an entry in a {@link JAXXContext}.
*
* Use the factory methods newContextEntryDef
and newListContextEntryDef
type of the entry associated to the definition
* @author chemit
*/
public class JAXXContextEntryDef implements java.io.Serializable {
/** name of the entry, can be nuill for a unamed entry. */
protected String name;
/** class of the entry, can not be null */
protected Class klass;
private static final long serialVersionUID = 1L;
// public static JAXXContextEntryDef newContextEntryDef(Class klass) {
// return newContextEntryDef(null, klass);
// }
//
// public static JAXXContextEntryDef newContextEntryDef(String name, Class klass) {
// return new JAXXContextEntryDef(name, klass);
// }
//
// public static JAXXContextEntryDef> newListContextEntryDef() {
// return newListContextEntryDef(null);
// }
//
// @SuppressWarnings({"unchecked"})
// public static JAXXContextEntryDef> newListContextEntryDef(String name) {
// Class> castList = JAXXContextEntryDef.castList();
// JAXXContextEntryDef> contextEntryDef = new JAXXContextEntryDef>(name,castList);
// contextEntryDef.klass = castList;
// return contextEntryDef;
// }
public String getName() {
return name;
}
public Class getKlass() {
return klass;
}
public O getContextValue(JAXXContext context) {
return context.getContextValue(klass, name);
}
public void removeContextValue(JAXXContext context) {
context.removeContextValue(klass, name);
}
public void setContextValue(JAXXContext context, O value) {
context.setContextValue(value, name);
}
@Override
public String toString() {
return super.toString() + "<" + klass + ":" + name + ">";
}
public JAXXContextEntryDef(Class klass) {
this(null, klass);
}
@SuppressWarnings("unchecked")
public JAXXContextEntryDef(String name, Class klass) {
if (klass == null) {
throw new IllegalArgumentException("class can not be null");
}
this.name = name;
if (List.class.isAssignableFrom(klass)) {
klass = (Class) List.class;
}
this.klass = klass;
}
//
// @SuppressWarnings({"unchecked"})
// protected static Class> castList() {
// return (Class>) Collections.emptyList().getClass();
// }
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof JAXXContextEntryDef>)) {
return false;
}
JAXXContextEntryDef> that = (JAXXContextEntryDef>) o;
return klass.equals(that.klass) && !(name != null ? !name.equals(that.name) : that.name != null);
}
@Override
public int hashCode() {
int result = (name != null ? name.hashCode() : 0);
return 31 * result + klass.hashCode();
}
public boolean accept(Class> klass, String name) {
if (klass == Object.class && this.klass != Object.class) {
// try on name only
return (this.name != null && name != null && this.name.equals(name));
}
return klass.isAssignableFrom(this.klass) && (this.name == null && name == null
|| (this.name != null && name != null && this.name.equals(name)));
}
public boolean accept2(Class> klass, String name) {
return !(klass == Object.class && this.klass != Object.class) &&
this.klass.isAssignableFrom(klass) && (this.name == null &&
name == null || (this.name != null && name != null && this.name.equals(name)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy